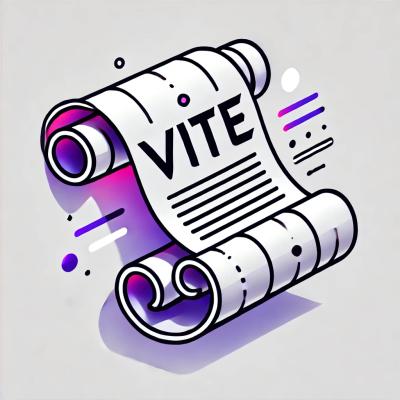
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
csp_evaluator
Advanced tools
Evaluate Content Security Policies for a wide range of bypasses and weaknesses
The csp_evaluator npm package is a tool designed to evaluate Content Security Policies (CSP) for potential security issues. It helps developers and security professionals ensure that their CSPs are robust and effective in mitigating various web security threats.
Evaluate CSP
This feature allows you to evaluate a given Content Security Policy (CSP) string for potential security issues. The `evaluate` function analyzes the CSP and returns an array of findings that highlight potential weaknesses or misconfigurations.
const cspEvaluator = require('csp_evaluator');
const csp = "default-src 'self'; script-src 'none';";
const findings = cspEvaluator.evaluate(csp);
console.log(findings);
Generate CSP Report
This feature generates a detailed report of the CSP evaluation. The `generateReport` function provides a comprehensive analysis of the CSP, including recommendations for improving security.
const cspEvaluator = require('csp_evaluator');
const csp = "default-src 'self'; script-src 'none';";
const report = cspEvaluator.generateReport(csp);
console.log(report);
The csp-parse package is used to parse and manipulate Content Security Policies. While it focuses more on parsing and transforming CSPs, it does not provide the same level of security evaluation and reporting as csp_evaluator.
Helmet-csp is a middleware for setting Content Security Policy headers in Express applications. It helps in defining and enforcing CSPs but does not offer evaluation or analysis of the policies like csp_evaluator.
The csp-header package is designed to help generate CSP headers for web applications. It provides utilities for building CSP strings but lacks the evaluation and security analysis features found in csp_evaluator.
Please note: this is not an official Google product.
CSP Evaluator allows developers and security experts to check if a Content Security Policy (CSP) serves as a strong mitigation against cross-site scripting attacks. It assists with the process of reviewing CSP policies, and helps identify subtle CSP bypasses which undermine the value of a policy. CSP Evaluator checks are based on a large-scale study and are aimed to help developers to harden their CSP and improve the security of their applications. This tool is provided only for the convenience of developers and Google provides no guarantees or warranties for this tool.
CSP Evaluator comes with a built-in list of common CSP allowlist bypasses which reduce the security of a policy. This list only contains popular bypasses and is by no means complete.
The CSP Evaluator library + frontend is deployed here: https://csp-evaluator.withgoogle.com/
This library is published to https://www.npmjs.com/package/csp_evaluator
. You
can install it via:
npm install csp_evaluator
To build, run:
npm install && tsc --build
To run unit tests, run:
npm install && npm test
import {CspEvaluator} from "csp_evaluator/dist/evaluator.js";
import {CspParser} from "csp_evaluator/dist/parser.js";
const parsed = new CspParser("script-src https://google.com").csp;
console.log(new CspEvaluator(parsed).evaluate());
FAQs
Evaluate Content Security Policies for a wide range of bypasses and weaknesses
The npm package csp_evaluator receives a total of 878,950 weekly downloads. As such, csp_evaluator popularity was classified as popular.
We found that csp_evaluator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.