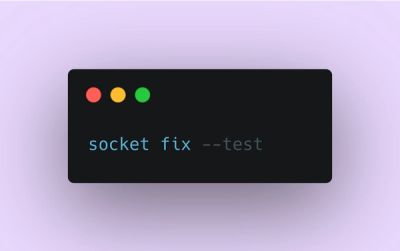
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
cypress-page-object-model
Advanced tools
Cypress Page Object Basic Model ( Ready To Use ) - UI Test Automation Design Pattern for Cypress.io
A production-ready Cypress automation framework with Page Object Model, supporting both UI and API testing.
git clone https://github.com/padmarajnidagundi/Cypress-POM-Ready-To-Use
cd Cypress-POM-Ready-To-Use
npm run presetup # Install all dependencies
npm run setup # Install Cypress
npm run verify # Verify Cypress installation
npm run cypress:open # Open Cypress Test Runner
npm run test:ui # Run UI tests
npm run test:api # Run API tests
npm run test:parallel # Run all tests in parallel
npm run test:ci # Run tests in CI mode
If you encounter the error 'cypress' is not recognized as an internal or external command
, follow these steps:
npm cache clean --force
rm -rf node_modules
rm -rf package-lock.json
npm run presetup
npm run verify
npm run cypress:open
// cypress/pageObjects/pages/loginPage.ts
import BasePage from '../basePage'
class LoginPage extends BasePage {
private selectors = {
username: '#username',
password: '#password',
submitBtn: '[data-testid="login-btn"]'
}
login(username: string, password: string) {
this.getElement(this.selectors.username).type(username)
this.getElement(this.selectors.password).type(password)
this.getElement(this.selectors.submitBtn).click()
}
}
// cypress/e2e/ui/login.cy.ts
import LoginPage from '../../pageObjects/pages/loginPage'
describe('Login Tests', () => {
const loginPage = new LoginPage()
beforeEach(() => {
loginPage.visit('/login')
})
it('should login successfully', () => {
loginPage.login('testuser', 'password')
// assertions
})
})
// cypress/e2e/api/users.cy.ts
describe('Users API', () => {
it('should create a new user', () => {
cy.request({
method: 'POST',
url: '/api/users',
body: {
name: 'Test User',
role: 'QA'
}
}).then(response => {
expect(response.status).to.eq(201)
})
})
})
Selectors
[data-testid="login-button"]
Test Organization
cypress/
βββ e2e/
β βββ api/ # API Tests
β β βββ users/
β β βββ auth/
β βββ ui/ # UI Tests
β βββ login/
β βββ dashboard/
βββ pageObjects/
β βββ components/ # Reusable components
β βββ pages/ # Page specific objects
βββ fixtures/ # Test data
// cypress.config.js
module.exports = {
env: {
apiUrl: 'https://api.dev.example.com',
userRole: 'admin',
featureFlags: {
newUI: true
}
}
}
npm run test:ci
pipeline {
agent any
stages {
stage('Test') {
steps {
sh 'npm ci'
sh 'npm run test:ci'
}
}
}
}
This framework uses Mochawesome for comprehensive HTML reporting. Get detailed insights with screenshots, videos, and test execution metrics.
npm run report:clean # Clean previous reports
npm run report:merge # Merge multiple report JSONs
npm run report:generate # Generate HTML from JSON
npm run test:report # Full test execution with reports
Report Features
Report Structure
cypress/reports/
βββ html/ # HTML reports
β βββ assets/ # Screenshots, videos
β βββ report.html # Main report
β βββ report.json # Combined results
βββ json/ # Individual test JSONs
cypress.config.js
:module.exports = defineConfig({
reporter: 'cypress-mochawesome-reporter',
reporterOptions: {
charts: true, // Enable charts
reportPageTitle: 'Test Report', // Custom title
embeddedScreenshots: true, // Inline screenshots
inlineAssets: true, // Inline assets
saveAllAttempts: false, // Save only failures
reportDir: 'cypress/reports/html',
overwrite: false,
html: true,
json: true
}
})
Viewing Reports
cypress/reports/html/report.html
in any browserCI/CD Integration
- name: Generate Test Report
if: always()
run: npm run test:report
- name: Upload Test Report
if: always()
uses: actions/upload-artifact@v4
with:
name: test-report
path: cypress/reports/html
Time Travel
cypress/screenshots
cypress/videos
Logging
cy.log()
for debug informationWe welcome contributions that help improve this Cypress Page Object Model framework! Here's how you can contribute:
Page Objects
Test Examples
Documentation
Fork and Clone
# Fork this repository on GitHub
git clone https://github.com/YOUR_USERNAME/Cypress-POM-Ready-To-Use.git
cd Cypress-POM-Ready-To-Use
npm install
Create a Branch
git checkout -b feature/your-feature-name
Make Changes
Contribution Guidelines
/**
* Example of a well-documented page object
*/
class ExamplePage extends BasePage {
private selectors = {
submitButton: '[data-testid="submit"]'
}
/**
* Submits the form with given data
* @param {string} data - The data to submit
* @returns {Cypress.Chainable}
*/
submitForm(data: string) {
return this.getElement(this.selectors.submitButton).click()
}
}
Run Tests
npm run test # Run all tests
npm run lint # Check code style
npm run build # Ensure build passes
Submit PR
main
branchcypress/
βββ e2e/ # Add tests here
β βββ api/ # API test examples
β βββ ui/ # UI test examples
βββ pageObjects/ # Add page objects here
β βββ components/ # Reusable components
β βββ pages/ # Page implementations
βββ support/ # Add custom commands here
βββ commands/ # Custom command implementations
Naming Conventions
LoginPage.ts
submitForm()
'should successfully submit form'
File Organization
Testing Standards
docs/
folderError with cypress.config.js
const { defineConfig } = require('cypress')
Solution: Ensure proper configuration setup:
npm install @cypress/browserify-preprocessor --save-dev
const { defineConfig } = require("cypress");
const createBundler = require("@cypress/browserify-preprocessor");
module.exports = defineConfig({
viewportWidth: 1920,
viewportHeight: 1080,
watchForFileChanges: false,
// ... other configurations
});
TypeScript Support
{
"devDependencies": {
"@cypress/browserify-preprocessor": "^3.0.2",
"@types/node": "^20.11.16",
"typescript": "^5.3.3"
}
}
Running Tests
npm run test:ui
npm run test:api
npm run test:parallel
Environment Configuration
https://reqres.in
https://react-redux.realworld.io
https://example.cypress.io
Page Object Model
Test Organization
cypress/e2e/api/
cypress/e2e/ui/
cypress/e2e/integration/
Performance
cy.session()
for login stateMIT License - feel free to use in your projects
FAQs
Cypress Page Object Basic Model ( Ready To Use ) - UI Test Automation Design Pattern for Cypress.io
The npm package cypress-page-object-model receives a total of 34 weekly downloads. As such, cypress-page-object-model popularity was classified as not popular.
We found that cypress-page-object-model demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
Weβre excited to announce a powerful new capability in Socket: historical data and enhanced analytics.