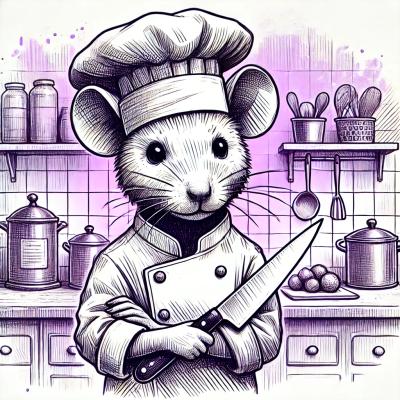
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
datafactory
Advanced tools
A lightweight node package for creating test data, demo data and fixtures.
import DataFactory from 'datafactory';
let _id = 0;
function generateId() {
return _id++;
}
// Each root key will become a builder function on the factory
let demoData = new DataFactory({
organization: {
_id: generateId,
name: 'Example organization'
},
user: {
_id: generateId,
name: 'Joe Smith',
// You can use a function to build a property for the document
organizationId: (group) => {
// Assign the user to the first organization in the group by default
if (group && group.data.organization) return group.data.organization[0]._id;
}
},
// You can use a function to build the entire document
tweet: (group) => {
const tweet = {
_id: generateId(),
text: 'Hi!'
};
// Assign the tweet to the first user in the group by default
if (group && group.data.user) {
tweet.userId = group.data.user[0]._id;
}
return tweet;
}
});
// Generate an organization, a user for the organization, and a tweet for the user -- you can chain calls.
let group = demoData.createGroup().organization().user().tweet({
text: 'Joe: cool demo data!'
});
// Create another user and a tweet for them
let elisabeth = group.user({
name: 'Elisabeth'
}).value;
group.tweet({
userId: elisabeth._id,
text: 'Elisabeth: Thanks!'
});
console.log('Generated documents\n\n', group.data);
console output
Generated documents
{
organization: [{
_id: 0,
name: 'Example organization'
}],
user: [{
_id: 1,
name: 'Joe Smith',
organizationId: 0
}, {
_id: 3,
name: 'Elisabeth',
organizationId: 0
}],
tweet: [{
_id: 2,
text: 'Joe: cool demo data!',
userId: 1
}, {
_id: 4,
text: 'Elisabeth: Thanks!',
userId: 3
}]
};
Follow the airbnb styleguide.
I recommend setting up the babel
and eslint
plugins for sublime.
Requires node ^5.0.0
.
npm install
npm run production
- Build task that generates minified scripts for productionnpm run precommit
- Run the unit tests and generate a minified scriptnpm run clean
- Remove the dist
foldernpm run eslint:source
- Lint the sourcenpm run eslint:common
- Lint the unit tests shared by Karma and Mochanpm run clean
- Remove the coverage report and the dist foldernpm run test
- Runs unit tests for both server and the browsernpm run test:browser
- Runs the unit tests for browser / clientnpm run test:server
- Runs the unit tests on the servernpm run watch:server
- Run all unit tests for server & watch files for changesnpm run watch:browser
- Run all unit tests for browser & watch files for changesnpm run karma:firefox
- Run all unit tests with Karma & Firefoxnpm run karma:chrome
- Run all unit tests with Karma & Chromenpm run packages
- List installed packagesnpm run package:purge
- Remove all dependenciesnpm run package:reinstall
- Reinstall all dependenciesnpm run package:updates
- shows a list over dependencies with a higher version number then the current one - if anynpm run package:upgrade
- Automaticly upgrade all dependencies and update package.jsonThank you @kflash for trolly the skeleton this project was started with.
MIT © Dispatch
FAQs
A lightweight node package for creating test / demo data and fixtures.
The npm package datafactory receives a total of 0 weekly downloads. As such, datafactory popularity was classified as not popular.
We found that datafactory demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.