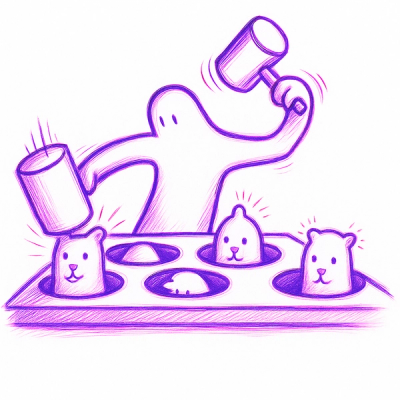
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
This project is an attempt to create a unified API for node.js SQL DB driver errors. Each driver throws their own kind of errors and libraries like knex, Bookshelf and objection.js simply pass these errors through. It's usually very difficult to reason with these errors. This library wraps those errors to error classes that are the same for all drivers. The wrapped error classes also expose useful information about the errors.
NOTE: Only MySQL, Sqlite3, MSSQL and PostgreSQL are officially supported (tested).
If you have an idea for an error we should handle, please open an issue and we'll see what we can do to add it.
const {
wrapError,
DBError,
UniqueViolationError,
NotNullViolationError
} = require('db-errors');
function errorHandler(err) {
// wrapError function takes any error and returns a DBError subclass instance if
// the input was an error thrown by the supported database drivers. Otherwise
// the input error is returned.
err = wrapError(err);
if (err instanceof UniqueViolationError) {
console.log(`Unique constraint ${err.constraint} failed for table ${err.table} and columns ${err.columns}`);
} else if (err instanceof NotNullViolationError) {
console.log(`Not null constraint failed for table ${err.table} and column ${err.column}`);
} else if (err instanceof DBError) {
console.log(`Some unknown DB error ${dbError.nativeError}`);
}
}
class DBError extends Error {
// The error thrown by the database client.
nativeError: Error
}
Base class for all errors.
class ConstraintViolationError extends DBError {
// No own properties.
}
A base class for all constraint violation errors
class UniqueViolationError extends ConstraintViolationError {
// The columns that failed.
//
// Available for: postgres, sqlite
columns: string[]
// The table that has the columns.
//
// Available for: postgres, sqlite
table: string
// The constraint that was violated.
//
// Available for: postgres, mysql
constraint: string
}
class NotNullViolationError extends ConstraintViolationError {
// The column that failed.
//
// Available for: postgres, sqlite, mysql
column: string
// The table that has the columns.
//
// Available for: postgres, sqlite
table: string
}
class ForeignKeyViolationError extends ConstraintViolationError {
// The table that has the foreign key.
//
// Available for: postgres, mysql
table: string
// The constraint that was violated.
//
// Available for: postgres, mysql
constraint: string
}
// This is not available for MySql since MySql doesn't have check constraints.
class CheckViolationError extends ConstraintViolationError {
// The table that has the check constraint.
//
// Available for: postgres
table: string
// The constraint that was violated.
//
// Available for: postgres
constraint: string
}
// Invalid data (string too long, invalid date etc.)
//
// NOTE: SQLite uses dynamic typing and doesn't throw this error.
class DataError extends DBError {
// There is no easy way to parse data from these kind
// of errors.
}
Run the following commands in the repo root:
docker-compose up
node setup-test-db.js
Run tests:
npm test
FAQs
Unified node.js error API for mysql, postgres and sqlite3
The npm package db-errors receives a total of 140,955 weekly downloads. As such, db-errors popularity was classified as popular.
We found that db-errors demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.