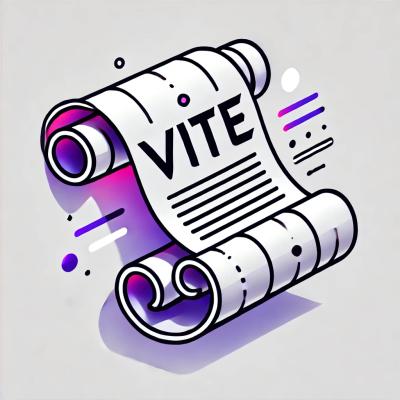
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
decision-tree
Advanced tools
This Node.js module implements a Decision Tree using the ID3 Algorithm
npm install decision-tree
var DecisionTree = require('decision-tree');
var training_data = [
{"color":"blue", "shape":"square", "liked":false},
{"color":"red", "shape":"square", "liked":false},
{"color":"blue", "shape":"circle", "liked":true},
{"color":"red", "shape":"circle", "liked":true},
{"color":"blue", "shape":"hexagon", "liked":false},
{"color":"red", "shape":"hexagon", "liked":false},
{"color":"yellow", "shape":"hexagon", "liked":true},
{"color":"yellow", "shape":"circle", "liked":true}
];
var test_data = [
{"color":"blue", "shape":"hexagon", "liked":false},
{"color":"red", "shape":"hexagon", "liked":false},
{"color":"yellow", "shape":"hexagon", "liked":true},
{"color":"yellow", "shape":"circle", "liked":true}
];
var class_name = "liked";
var features = ["color", "shape"];
var dt = new DecisionTree(class_name, features);
dt.train(training_data);
Alternately, you can also create and train the tree when instantiating the tree itself:
var dt = new DecisionTree(training_data, class_name, features);
var predicted_class = dt.predict({
color: "blue",
shape: "hexagon"
});
var accuracy = dt.evaluate(test_data);
var treeJson = dt.toJSON();
var treeJson = dt.toJSON();
var preTrainedDecisionTree = new DecisionTree(treeJson);
Alternately, you can also import a previously trained model on an existing tree instance, assuming the features & class are the same:
var treeJson = dt.toJSON();
dt.import(treeJson);
FAQs
NodeJS implementation of decision tree using ID3 algorithm
The npm package decision-tree receives a total of 664 weekly downloads. As such, decision-tree popularity was classified as not popular.
We found that decision-tree demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.