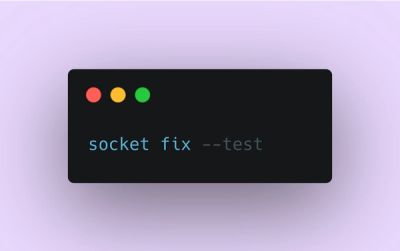
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
deepseek-api
Advanced tools
DeepSeek API is a powerful Node.js library designed to interact with DeepSeek's chat system. With this library, you can create chat sessions, send messages, and handle chat streams efficiently.
To install the DeepSeek API package, use the following command:
npm install deepseek-api
DeepSeek uses Cloudflare for changing IPs, which might cause issues when using this API server-side with tokens obtained from your browser. We are actively working on a workaround solution to address this.
import { sendMessage, createNewChat, chats } from 'deepseek-api';
createNewChat(token: string, id?: string): Promise<string | { error: string }>
Creates a new chat session and stores its details in the chats
map.
Parameters:
token
(string): The authentication token for DeepSeek.id
(string, optional): A custom chat ID. If not provided, the original ID from DeepSeek will be used.Returns:
Example:
const chatID = await createNewChat('your-token-here');
console.log(`Chat created with ID: ${chatID}`);
sendMessage(text: string, chat: { id: string; token: string; parent_id?: string }, callback: Function): Promise<any>
Sends a message to the specified chat session and streams the response.
Parameters:
text
(string): The message to send.chat
(object): The chat details, including:
id
(string): The chat session ID.token
(string): The authentication token.parent_id
(string, optional): The ID of the message to reply to (defaults to the last message in the chat).callback
(Function): A callback function to process each chunk of the response.Returns:
Example:
await sendMessage('Hello, DeepSeek!', {
id: 'chat-id-here',
token: 'your-token-here'
}, (chunk) => {
console.log('Stream chunk:', chunk);
});
chats
A Map
object that stores all chat details, indexed by their IDs. Each chat entry includes:
id
: The chat session ID.token
: The authentication token.cookies
: Any associated cookies (currently null
).requestChatStream(payload: object, text: string): Promise<any>
Initiates a chat stream request.
streamResponse(response: any, callback: Function): Promise<any>
Processes a streaming response, invoking the callback for each chunk.
To authenticate with DeepSeek:
Log in to DeepSeek Chat.
Retrieve the token using the browser's console:
localStorage.getItem('userToken');
The token will look like this:
{"value":"your-token-here","__version":"0"}
Use the value
field from the token object as your authentication token.
Here is a complete example of creating a chat session and sending a message:
import { createNewChat, sendMessage } from 'deepseek-api';
(async () => {
const token = 'your-token-here';
// Step 1: Create a new chat session
const chatID = await createNewChat(token);
if (typeof chatID === 'string') {
console.log(`Chat session created: ${chatID}`);
// Step 2: Send a message
await sendMessage('Hello, DeepSeek!', {
id: chatID,
token: token
}, (chunk) => {
console.log('Response chunk:', chunk);
});
} else {
console.error('Error creating chat session:', chatID.error);
}
})();
chats
map before sending a message.This project is licensed under the MIT License. See the LICENSE file for details.
This package currently retrieves raw JSON responses directly from the DeepSeek API. We are actively working on enhancing the library to parse this data and provide a user-friendly interface that mimics a real chat experience. Stay tuned for updates!
Contributions, issues, and feature requests are welcome! Feel free to check the issues page.
The library now provides structured response objects for different types of messages:
{
type: 'message',
content: 'The message content',
id: 'message-id',
role: 'assistant',
metadata: {
model: 'model-name',
usage: { /* token usage stats */ },
finish_reason: 'stop'
},
timestamp: '2024-01-01T00:00:00.000Z'
}
// Done Response
{
type: 'done',
timestamp: '2024-01-01T00:00:00.000Z'
}
// Error Response
{
type: 'error',
error: 'error_type',
details: 'Error details',
timestamp: '2024-01-01T00:00:00.000Z'
}
// Thinking State
{
type: 'thinking',
status: 'thinking_status',
timestamp: '2024-01-01T00:00:00.000Z'
}
// Search Results
{
type: 'search',
results: [/* search results */],
timestamp: '2024-01-01T00:00:00.000Z'
}
Here's how to use the enhanced parsing features:
import { createNewChat, sendMessage } from 'deepseek-api';
(async () => {
const token = 'your-token-here';
const chatID = await createNewChat(token);
if (typeof chatID === 'string') {
await sendMessage('Write a JavaScript function', {
id: chatID,
token: token
}, (response) => {
switch(response.type) {
case 'message':
console.log('Content:', response.content);
break;
case 'thinking':
console.log('AI is thinking...');
break;
case 'error':
console.error('Error:', response.error);
break;
case 'done':
console.log('Response complete');
break;
}
});
}
})();
message
: Regular message contentthinking
: AI processing statussearch
: Search results when enablederror
: Error informationdone
: Completion indicatorFAQs
Simple api for accessing deepseek free api
The npm package deepseek-api receives a total of 139 weekly downloads. As such, deepseek-api popularity was classified as not popular.
We found that deepseek-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.