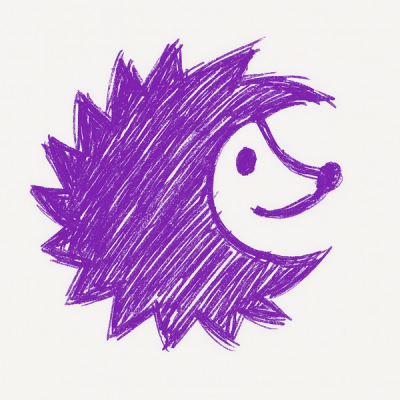
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
deferred-exec
Advanced tools
Deferred based tool to run exec commands. Lets you use exec, execFile and spawn in a sane way.
Version: 0.3.1
Install the module with: npm install deferred-exec
or add it to your project's package.json
file.
You can also clone this repo and npm install folderOfClonedRepo
to get a branch or development copy.
All calls return a promise, which means it's easy to do stuff when they complete or fail:
var dexec = require( 'deferred-exec' );
dexec( 'echo "yay"' )
.done( function( stdout, stderr, command ) {
console.log( stdout ); // logs "yay"
})
.fail( function( error ) {
console.log( "it didn't work :( got code:", error.code );
});
Since they are deferreds, you can pass them around in your code:
var dexec = require( 'deferred-exec' );
var command = dexec( 'echo "gotcha"' );
doSomethingWithCommand( command );
// meanwhile, in some other part of your application
function doSomethingWithCommand( command ) {
command.done( function( stdout, stderr, command ) {
console.log( 'just ran', command, 'and got', stdout );
});
}
Use Underscore.Deferred if you want to use
_.when
to group multiple commands. (Note: you can use underscore.deferred with lodash)
var dexec = require( 'deferred-exec' );
// require and mixin lodash with _.deferred
var _ = require( 'lodash' );
_.mixin( require( 'underscore.deferred' ) );
var commandA = dexec( 'ls /etc' );
var commandB = dexec( 'echo "hi"' );
// when both commands succeed
_.when( commandA, commandB )
.done( function( a, b ){
console.log( 'commandA output:', a[0] );
console.log( 'commandB output:', b[0] );
});
Or run a file using .file
var dexec = require( 'deferred-exec' );
dexec.file( './someFile.sh' )
.done( function( stdout, stderr, fileName ) {
console.log( 'ran', fileName, 'got', stdout );
});
Or take advantage of spawning a new progress and getting its output during execution:
var dexec = require( 'deferred-exec' );
dexec.spawn( 'cat', [ '/var/log/syslog' ] )
.progress( function( stdout, stderr, command ) {
/* this function will get called with every piece of
data from the returne result */
})
.done( function( stdout, stderr, command ) {
/* all done! total value's available of course */
});
For deferred-exec
details see child_process.exec for available options
For deferred-exec.file
details see child_process.execFile
for available options
For deferred-exec.spawn
details see child_progress.spawn for available options
All methods support an options object. deferred-exec
adds two possible options to this object:
This defaults to true
, which trims the last bit of trailing while space (a new line) from the output.
Set to false
if you don't want your final output trimmed.
The default is utf8
, so if you want one of the other types supported you can specify it here.
Assuming `var dexec = require( 'deferred-exec' ):
All methods return a promise. Check out the Deferred Documentation.
Only .spawn
utilizes the notify()
method.
In lieu of a formal styleguide, take care to maintain the existing coding style. Add unit tests for any new or changed functionality. Lint and test your code using grunt.
Copyright (c) 2012 Dan Heberden
Licensed under the MIT license.
FAQs
Deferred based tool to run exec commands
The npm package deferred-exec receives a total of 14 weekly downloads. As such, deferred-exec popularity was classified as not popular.
We found that deferred-exec demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.