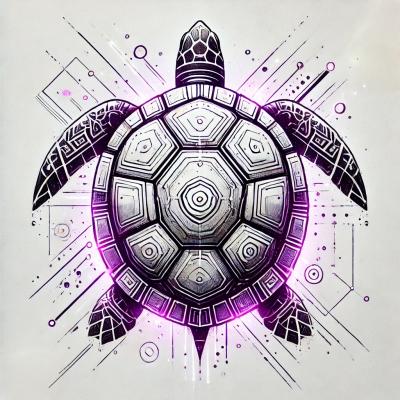
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
A simple way for solving $ref
values:
var deref = require('deref');
$ = deref();
var a = {
id: 'a',
type: 'object',
properties: {
b: {
$ref: 'b'
}
}
};
var b = {
id: 'b',
type: 'string'
};
var c = {
id: 'c',
type: 'array',
items: {
$ref: 'a'
}
};
console.log($(c, [b, a]).id);
// output: http://json-schema.org/c#
console.log($(c, [b, a], true).items.properties.b.type);
// output: string
var schema = {
id: 'http://x.y.z/rootschema.json#',
schema1: {
id: '#foo'
},
schema2: {
id: 'otherschema.json',
nested: {
id: '#bar'
},
alsonested: {
id: 't/inner.json#a'
}
},
schema3: {
id: 'some://where.else/completely#'
}
};
console.log(deref.util.normalizeSchema(schema).schema2.nested.id);
// output: http://x.y.z/otherschema.json#bar
Since 0.3.0
the schema id
will always be normalized internally to #
when it's not provided.
This way the passed schema can be self-referenced using $ref
's which is the expected behavior.
I know the id
keyword is not required but while #/
is a self-reference we can assume #
as the schema-id.
deref
use that id
for store and find $ref
's, even self-references.
Without it is complex determine what to resolve. :beers:
The resulting function of calling deref()
can accept three arguments:
fakeroot (string)
Used on missing $schema
values for resolve into fully qualified URIs.
console.log($('http://example.com', { id: '#foo' }).id);
// output: http://example.com#foo
If missing will use http://json-schema.org/schema
.
schema (object)
The JSON-Schema object for dereferencing.
refs (array)
Any additional schemas used while dereferencing.
Since 0.2.4
passing an object is not longer supported.
ex (boolean)
Whether do full dereferencing or not, false
by default.
Since 0.6.0
all inner references are not dereferenced by default.
All other references are always dereferenced regardless the value of ex
.
$('http://example.com', schema, true);
$(schema, refs, true);
$(schema, true);
Aside the basics of $
, this function will include:
$.refs (object)
An registry of dereferenced schemas.
$.util (object)
Exposes the internal helpers used by deref
.
isURL(path)
parseURI(href)
resolveURL(base, href)
getDocumentURI(path)
findByRef(uri, refs)
resolveSchema(schema, refs)
normalizeSchema(fakeroot, schema)
Any refs
passed MUST be an object of normalized schemas.
Note that calling $(schema)
will not read/download any local/remote files.
FAQs
JSON-Schema $ref resolution
The npm package deref receives a total of 10,313 weekly downloads. As such, deref popularity was classified as popular.
We found that deref demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.