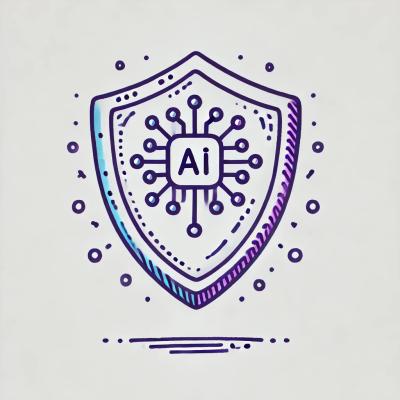
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
detect-port-alt
Advanced tools
The detect-port-alt npm package is a utility that helps in checking if a port is already in use and if so, it can suggest an alternative port that is free. This is particularly useful in network programming and development environments where handling port conflicts efficiently is crucial.
Check if a port is in use
This feature allows you to check if a specific port (e.g., 3000) is available. If the port is occupied, it suggests another free port.
const detectPort = require('detect-port-alt');
detectPort(3000, (err, port) => {
if (err) {
console.error(err);
} else if (port === 3000) {
console.log('Port 3000 is available');
} else {
console.log('Port 3000 is occupied. Try port:', port);
}
});
Promise-based port detection
This feature provides a promise-based API to check for port availability, making it easier to integrate with modern JavaScript applications using async/await.
const detectPort = require('detect-port-alt');
async function getAvailablePort(startingPort) {
try {
const port = await detectPort(startingPort);
console.log(`Port ${port} is available`);
return port;
} catch (error) {
console.error('Error:', error);
}
}
getAvailablePort(3000);
Portfinder is a popular npm package that also helps in finding an open port on the current machine. Unlike detect-port-alt, portfinder provides more configuration options such as setting a range of ports to check. This can be particularly useful when you need to find multiple free ports within a specific range.
Get-port is another similar package that provides an easy-to-use API to get an available port. It's very minimalistic compared to detect-port-alt and does not provide callback support, focusing solely on promises, which makes it suitable for use in applications fully based on modern JavaScript features.
JavaScript Implementation of Port Detector
$ npm i detect-port --save
const detect = require('detect-port');
/**
* callback usage
*/
detect(port, (err, _port) => {
if (err) {
console.log(err);
}
if (port == _port) {
console.log(`port: ${port} was not occupied`);
} else {
console.log(`port: ${port} was occupied, try port: ${_port}`);
}
});
/**
* for a yield syntax instead of callback function implement
*/
const co = require('co');
co(function *() {
const _port = yield detect(port);
if (port == _port) {
console.log(`port: ${port} was not occupied`);
} else {
console.log(`port: ${port} was occupied, try port: ${_port}`);
}
});
/**
* use as a promise
*/
detect(port)
.then(_port => {
if (port == _port) {
console.log(`port: ${port} was not occupied`);
} else {
console.log(`port: ${port} was occupied, try port: ${_port}`);
}
})
.catch(err => {
console.log(err);
});
$ npm i detect-port -g
# get an available port randomly
$ detect
# detect pointed port
$ detect 80
# more help
$ detect --help
FAQs
detect available port
The npm package detect-port-alt receives a total of 731,452 weekly downloads. As such, detect-port-alt popularity was classified as popular.
We found that detect-port-alt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.