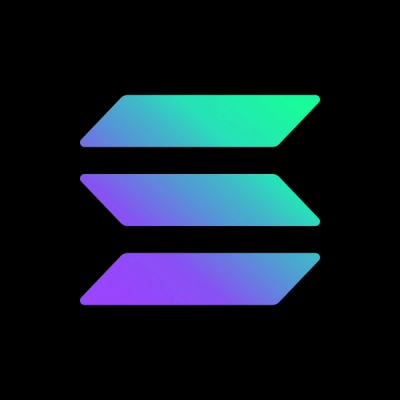
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
deterministic-hash
Advanced tools
A deterministic value hashing algorithm for node.js.
Pass any Javascript value and receive a deterministic hash (in hexadecimal) of the value. If an object is passed, only own enumerable string-keyed properties are used, the order of the keys does not matter as long as all of the values match.
import deterministicHash from 'deterministic-hash';
const objA = { a: 'x', arr: [1,2,3,4], b: 'y' };
const objB = { b: 'y', a: 'x', arr: [1,2,3,4] };
deterministicHash({
c: [ objA, objB ],
b: objA,
e: objB,
f: ()=>{ Math.random(); },
g: Symbol('Unique identity'),
h: new Error('AHHH')
});
// -> 455b32a99a84efad551409b39bd91fc028a98343
deterministicHash({
h: new Error('AHHH'),
e: objB,
g: Symbol('Unique identity'),
b: objA,
f: ()=>{ Math.random(); },
c: [ objA, objB ]
});
// -> 455b32a99a84efad551409b39bd91fc028a98343
A hash algorithm can be passed as the second argument. This takes any value that is valid for crypto.createHash
. The default is sha1
.
deterministicHash('value', 'sha1');
// -> f32b67c7e26342af42efabc674d441dca0a281c5
deterministicHash('value', 'sha256');
// -> cd42404d52ad55ccfa9aca4adc828aa5800ad9d385a0671fbcbf724118320619
deterministicHash('value', 'sha512');
// -> ec2c83edecb60304d154ebdb85bdfaf61a92bd142e71c4f7b25a15b9cb5f3c0ae301cfb3569cf240e4470031385348bc296d8d99d09e06b26f09591a97527296
Currently this has only been tested on Node.js 16.3.x
. More tests are to come and this section will be updated as I test them.
FAQs
A deterministic value hashing algorithm for node.js.
The npm package deterministic-hash receives a total of 2 weekly downloads. As such, deterministic-hash popularity was classified as not popular.
We found that deterministic-hash demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.