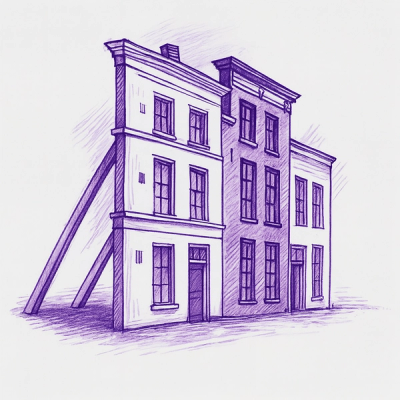
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
The 'di' npm package is a lightweight dependency injection (DI) library for JavaScript and Node.js. It allows developers to manage dependencies in a modular and scalable way, which is particularly useful in large applications or when implementing inversion of control (IoC) patterns.
Dependency Registration
This feature allows you to register dependencies with the injector. In the code sample, an API key is registered as a value dependency.
const Injector = require('di');
const injector = new Injector();
injector.value('apiKey', '12345');
Dependency Resolution
This feature involves resolving and injecting dependencies into services or other components. The code sample demonstrates how to resolve a 'MyService' instance with 'apiKey' injected into it.
const Injector = require('di');
const injector = new Injector();
function MyService(apiKey) { this.apiKey = apiKey; }
injector.value('apiKey', '12345');
injector.service('myService', MyService, ['apiKey']);
const myServiceInstance = injector.get('myService');
Child Injectors
Child injectors inherit dependencies from their parent injector but can also override them. This feature is useful for creating isolated scopes.
const Injector = require('di');
const parentInjector = new Injector();
const childInjector = parentInjector.createChild();
parentInjector.value('apiKey', '12345');
childInjector.get('apiKey');
Inversify is a powerful and flexible inversion of control library for JavaScript & Node.js apps powered by TypeScript. It offers more advanced features like decorators and metadata reflection, which makes it more suitable for complex projects compared to the simpler 'di' package.
BottleJS is a minimalistic dependency injection micro container for JavaScript. It provides similar functionalities to 'di' but also includes middleware support and is slightly more feature-rich while still being lightweight.
Awilix is a dependency injection container for Node.js with support for ES6 Classes and a focus on modularity and testability. It differs from 'di' by offering more comprehensive configuration options and automatic resolution of dependencies.
Heavily influenced by AngularJS and its implementation of dependency injection. Inspired by Guice and Pico Container.
FAQs
Dependency Injection for Node.js. Heavily inspired by AngularJS.
The npm package di receives a total of 3,253,374 weekly downloads. As such, di popularity was classified as popular.
We found that di demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.