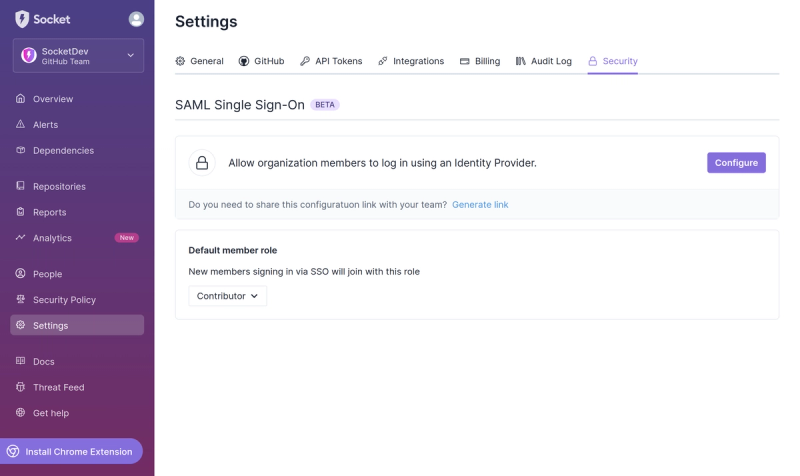
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
discord-bot-lib
Advanced tools
Readme
A basic discord bot lib for node.js but the library doesn't cache discord objects.
$ npm install discord-bot-lib
// require the lib
const Client = require('discord-bot-lib');
// create the client
const bot = new Client({
token: "Discord Token",
prefix: "/"
});
bot.addEventListener('ready', data => {
console.log(`The bot is ready! Serving ${data.guilds.length} guilds.`);
});
bot.addEventListener('error', async (ctx, err) => {
await ctx.send(`Whoopsies! The bot did an oopsie woopsie!\n\`${err}\``);
});
bot.addEventListener('close', () => console.log("Goodbye, world! :c"));
// simple command handler example
bot.command({
name: "ping",
aliases: ["pong"],
run: async (ctx) => {
return await ctx.send('Pong');
}
});
// run the bot
bot.run();
FAQs
A basic discord bot library for node.js but the library doesn't cache discord objects.
The npm package discord-bot-lib receives a total of 0 weekly downloads. As such, discord-bot-lib popularity was classified as not popular.
We found that discord-bot-lib demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.