Comparing version 1.1.0 to 1.2.0
# disparity changelog | ||
## v1.2.0 (2015/04/01) | ||
- add `unifiedNoColor` support. | ||
## v1.1.0 (2015/04/01) | ||
@@ -4,0 +8,0 @@ |
@@ -12,2 +12,3 @@ 'use strict'; | ||
unified: argv.indexOf('-u') !== -1 || argv.indexOf('--unified') !== -1, | ||
noColor: argv.indexOf('--no-color') !== -1, | ||
filePath1: argv[argv.length - 2] || '--', | ||
@@ -26,2 +27,6 @@ filePath2: argv[argv.length - 1] || '--', | ||
if (args.noColor && args.chars) { | ||
args.errors.push('Error: "--no-color" flag can only be used with "--unified" diff.'); | ||
} | ||
return args; | ||
@@ -60,3 +65,4 @@ }; | ||
if (args.unified) { | ||
out.write(disparity.unified(f1, f2, p1, p2)); | ||
var method = args.noColor ? 'unifiedNoColor' : 'unified'; | ||
out.write(disparity[method](f1, f2, p1, p2)); | ||
return 0; | ||
@@ -63,0 +69,0 @@ } |
@@ -10,2 +10,3 @@ 'use strict'; | ||
exports.unified = unified; | ||
exports.unifiedNoColor = unifiedNoColor; | ||
exports.chars = chars; | ||
@@ -92,3 +93,2 @@ exports.removed = 'removed'; | ||
function replaceInvisibleChars(str) { | ||
@@ -101,3 +101,2 @@ return str | ||
function rightAlign(val, nChars) { | ||
@@ -109,3 +108,2 @@ val = val.toString(); | ||
function removeLinesOutOfContext(lines, context) { | ||
@@ -146,3 +144,2 @@ var diffMap = {}; | ||
function unified(oldStr, newStr, filePathOld, filePathNew) { | ||
@@ -153,2 +150,15 @@ if (newStr === oldStr) { | ||
var changes = unifiedNoColor(oldStr, newStr, filePathOld, filePathNew) | ||
.replace(/^\-.*/gm, red('$&')) | ||
.replace(/^\+.*/gm, green('$&')) | ||
.replace(/^@@.+/gm, yellow('$&')); | ||
return changes; | ||
} | ||
function unifiedNoColor(oldStr, newStr, filePathOld, filePathNew) { | ||
if (newStr === oldStr) { | ||
return ''; | ||
} | ||
filePathOld = filePathOld || ''; | ||
@@ -170,8 +180,3 @@ filePathNew = filePathNew || filePathOld; | ||
changes = changes | ||
.replace(/^\-.*/gm, red('$&')) | ||
.replace(/^\+.*/gm, green('$&')) | ||
.replace(/^@@.+/gm, yellow('$&')); | ||
return changes; | ||
} |
{ | ||
"name": "disparity", | ||
"version": "1.1.0", | ||
"version": "1.2.0", | ||
"description": "Colorized string diff ideal for text/code that spans through multiple lines", | ||
@@ -5,0 +5,0 @@ "main": "disparity.js", |
@@ -47,7 +47,8 @@ # disparity | ||
// text displayed just before the diff | ||
header: '\u001b[41mmremoved\u001b[49m \u001b[42madded\u001b[49m\n\n' | ||
header: '\u001b[41m' + disparity.removed '\u001b[49m \u001b[42m' + | ||
disparity.added + '\u001b[49m\n\n' | ||
}; | ||
``` | ||
 | ||
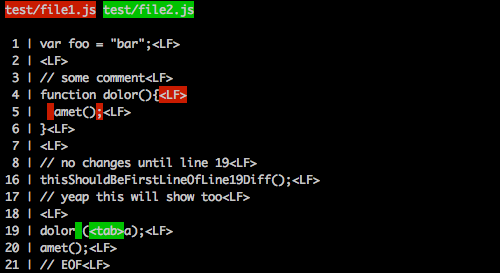 | ||
@@ -59,2 +60,4 @@ ### unified(oldStr, newStr[, filePathOld, filePathNew]):String | ||
Will return an empty string if `oldStr === newStr`; | ||
```js | ||
@@ -65,6 +68,18 @@ var diff = disparity.unified(file1, file2); | ||
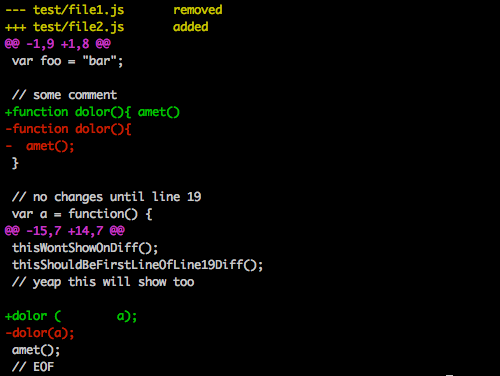 | ||
### unifiedNoColor(oldStr, newStr[, filePathOld, filePathNew]):String | ||
Returns [unified diff](http://en.wikipedia.org/wiki/Diff_utility#Unified_format). | ||
Useful for terminals that [doesn't support colors](https://www.npmjs.com/package/supports-color). | ||
Will return an empty string if `oldStr === newStr`; | ||
 | ||
```js | ||
var diff = disparity.unifiedNoColor(file1, file2); | ||
console.log(diff); | ||
``` | ||
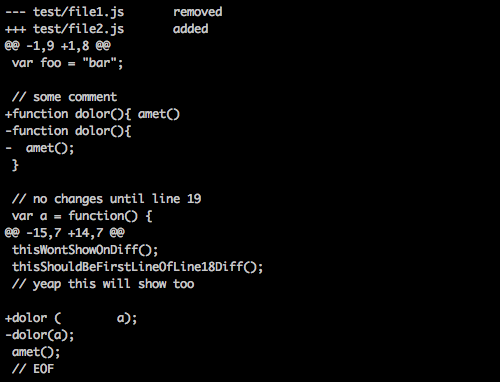 | ||
### removed:String | ||
@@ -96,4 +111,5 @@ | ||
Options: | ||
-c, --chars Output char diff (default mode). | ||
-u, --unified Output unified diff. | ||
-c, --chars Output char diff (default mode). | ||
--no-color Don't output colors. | ||
-v, --version Display current version. | ||
@@ -100,0 +116,0 @@ -h, --help Display this help. |
@@ -11,3 +11,3 @@ [41mremoved[49m [42madded[49m | ||
8 | // no changes until line 19<LF> | ||
16 | thisShouldBeFirstLineOfLine18Diff();<LF> | ||
16 | thisShouldBeFirstLineOfLine19Diff();<LF> | ||
17 | // yeap this will show too<LF> | ||
@@ -14,0 +14,0 @@ 18 | <LF> |
@@ -16,3 +16,3 @@ var foo = "bar"; | ||
thisWontShowOnDiff(); | ||
thisShouldBeFirstLineOfLine18Diff(); | ||
thisShouldBeFirstLineOfLine19Diff(); | ||
// yeap this will show too | ||
@@ -19,0 +19,0 @@ |
@@ -15,3 +15,3 @@ var foo = "bar"; | ||
thisWontShowOnDiff(); | ||
thisShouldBeFirstLineOfLine18Diff(); | ||
thisShouldBeFirstLineOfLine19Diff(); | ||
// yeap this will show too | ||
@@ -18,0 +18,0 @@ |
@@ -29,2 +29,3 @@ 'use strict'; | ||
var diff, expected; | ||
var file1 = readFile('file1.js'); | ||
@@ -36,4 +37,4 @@ var file2 = readFile('file2.js'); | ||
var diff = disparity.chars(file1, file2); | ||
var expected = readFile('chars.txt'); | ||
diff = disparity.chars(file1, file2); | ||
expected = readFile('chars.txt'); | ||
compare(diff, expected, 'chars'); | ||
@@ -52,2 +53,9 @@ | ||
// unifiedNoColor | ||
// ============== | ||
diff = disparity.unifiedNoColor(file1, file2, 'test/file1.js', 'test/file2.js'); | ||
expected = readFile('unified_no_color.txt'); | ||
compare(diff, expected, 'unified_no_color'); | ||
// cli.parse | ||
@@ -94,2 +102,10 @@ // ========= | ||
args = cli.parse(['--no-color', 'foo.js', 'bar.js']); | ||
assert.ok(args.errors.length, '--no-color errors'); | ||
assert.ok(args.noColor, '--no-color'); | ||
args = cli.parse(['--unified', '--no-color', 'foo.js', 'bar.js']); | ||
assert.ok(!args.errors.length, '--unified --no-color errors'); | ||
assert.ok(args.noColor, '--no-color'); | ||
// cli.run | ||
@@ -148,1 +164,12 @@ // ======= | ||
assert.equal(err.data, ''); | ||
code = run({ | ||
unified: true, | ||
noColor: true, | ||
filePath1: 'test/file1.js', | ||
filePath2: 'test/file2.js' | ||
}); | ||
expected = readFile('unified_no_color.txt'); | ||
assert.ok(!code, 'exit code chars'); | ||
assert.equal(out.data, expected); | ||
assert.equal(err.data, ''); |
@@ -16,3 +16,3 @@ [31m--- test/file1.js removed[39m | ||
thisWontShowOnDiff(); | ||
thisShouldBeFirstLineOfLine18Diff(); | ||
thisShouldBeFirstLineOfLine19Diff(); | ||
// yeap this will show too | ||
@@ -19,0 +19,0 @@ |
@@ -16,3 +16,3 @@ [31m--- removed[39m | ||
thisWontShowOnDiff(); | ||
thisShouldBeFirstLineOfLine18Diff(); | ||
thisShouldBeFirstLineOfLine19Diff(); | ||
// yeap this will show too | ||
@@ -19,0 +19,0 @@ |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
108836
18
377
121