dropbox-file-picker
Simple Dropbox chooser replacement (files and folders picker) with browser and Electron support.
List layout example:
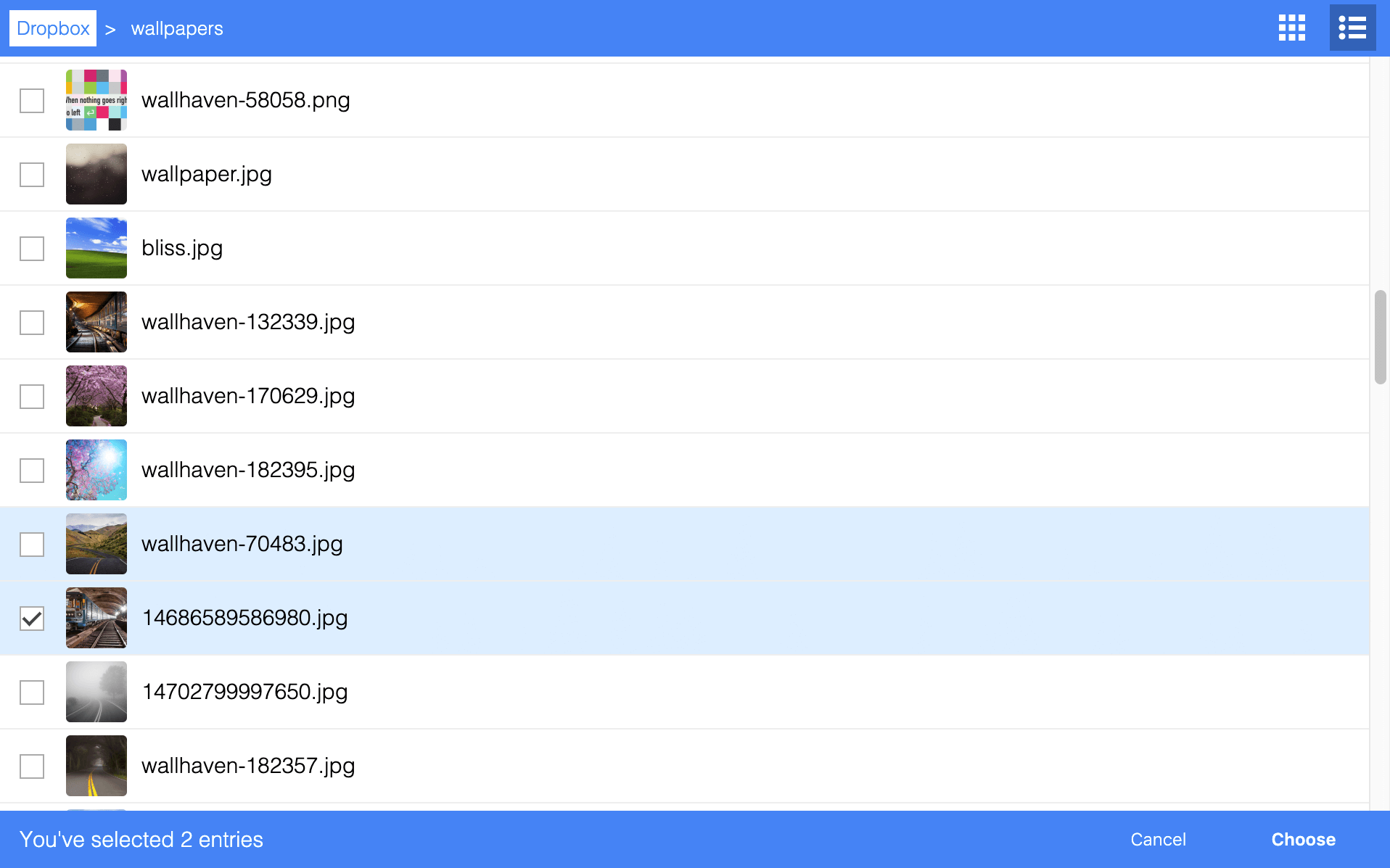
Grid layout example:
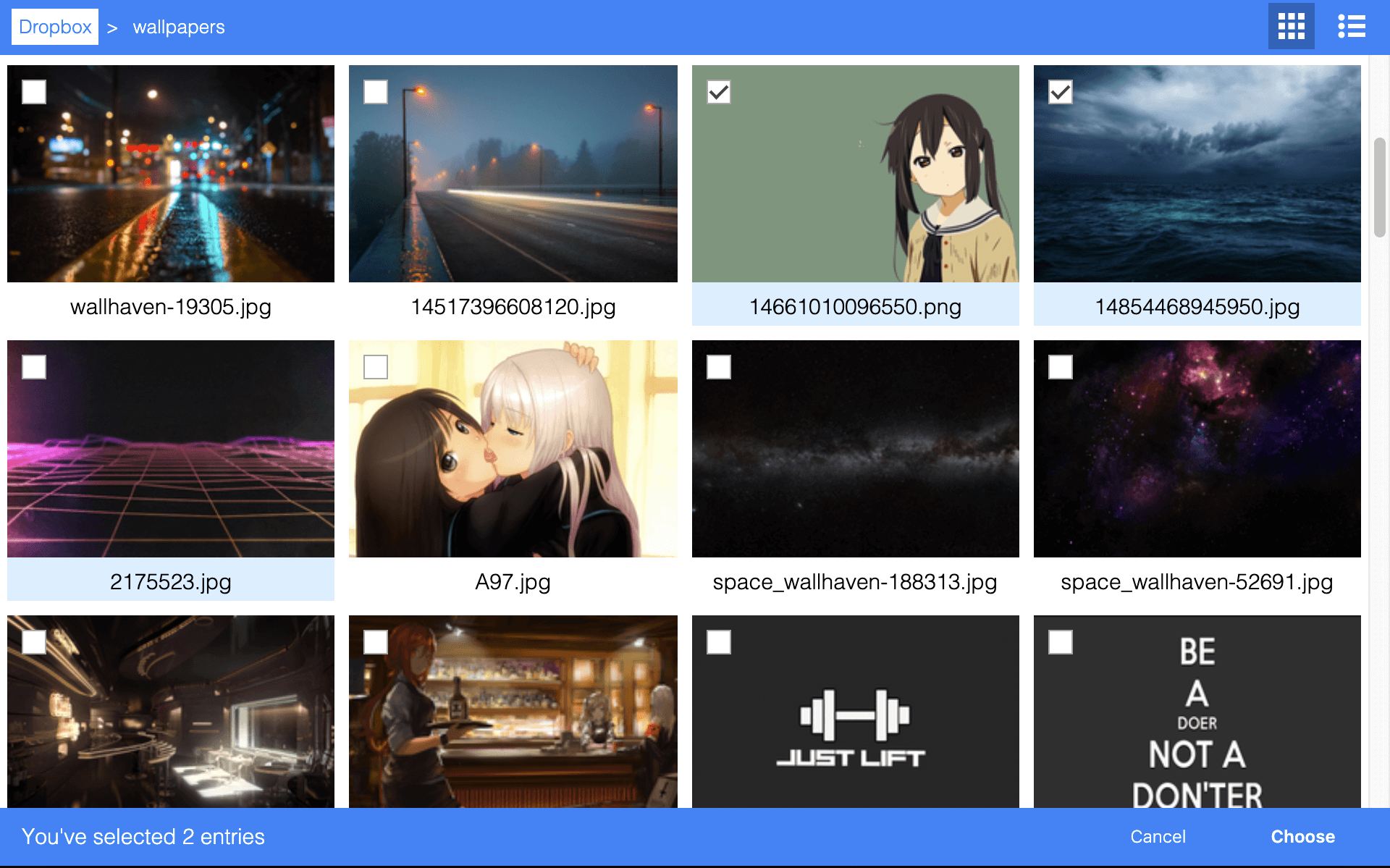
Features:
- Independent from default Dropbox chooser
- Without using of external window or iframe
- Supports image files previews
- Ability to select multiple files & folders at the same time
- Supports grid and list layout modes
- Localization support
- Adjustable grid layout columns count
Installation:
npm i dropbox-file-picker
Notes:
- Currently works only as modal window
- No ability to upload files/authorize/create folders (will be implemented later)
Usage:
Minimal setup:
import dropboxPicker from 'dropbox-file-picker';
dropboxPicker.open({ accessToken: 'dropbox_access_token_here' })
.then(result => onSuccess(result));
Advanced setup:
import dropboxPicker from 'dropbox-file-picker';
dropboxPicker.open({
accessToken: 'dropbox_access_token_here',
allowedExtensions: extensions,
allowFolderSelection: false,
isMultiple: true,
loadPreviews: true,
hideCountLabel: false,
hideCheckboxes: false,
rows: 4,
defaultLayout: 'list',
disableLayoutSelection: true,
width: '700px',
previewSettings: {
size: 'w256h256',
},
localization: {
title: 'Dropbox',
cancel: 'Cancel',
choose: 'Choose',
entriesSelectionLabel: 'You\'ve selected {0} entries'
}
})
.then(
result => onSuccess(result),
result => onClose(result)
);
Supported previews size: w32h32
w64h64
w128h128
w256h256
w480h320
w640h480
w960h640
w1024h768
w2048h1536
Response format example:
[
{
.tag: "folder"
id: <folder_id>
name: <folder_name>
path_display: <full_folder_path>
path_lower: <full_folder_path_lowercase>
},
{
.tag: "file"
client_modified: <iso_timtestamp>
content_hash: <content_hash>
id: <file_id>
is_downloadable: <bool_is_downloadable>
name: <string_file_name_with_extension>
path_display: <full_file_path>
path_lower: <full_file_path_lowercase>
rev: <rev_id>
server_modified: <iso_timtestamp>
size: <number_file_size>
thumbnailUrl: <string_base64_thumbnail>
}
]