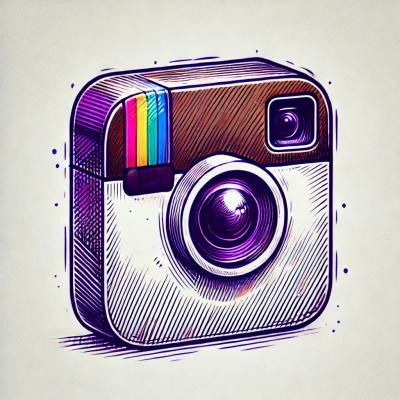
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
dropletapi
Advanced tools
(C) Oliwer Helsén (oliwer.helsen@live.com) 2015
A wrapper for DigitalOceans API v2
See Version History for changes
npm install dropletapi
npm install dropletapi --save
-- DROPLETS
-- Actions
Create a new Droplet
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
var myNewDropletData = {
"name": "example.com",
"region": "nyc3",
"size": "512mb",
"image": "ubuntu-14-04-x64",
"ssh_keys": null,
"backups": false,
"ipv6": true,
"user_data": null,
"private_networking": null
}
digitalocean.createDroplet(myNewDropletData, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Retrieve an existing Droplet by id
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.getDropletById(PUT THE DROPLETID HERE, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
List all Droplets in your account
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.listDroplets(function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Delete a Droplet by id
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.deleteDroplet(PUT THE DROPLETID HERE, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Retrieve a list of all kernels available to a Dropet
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.availableKernelsForDroplet(PUT THE DROPLETID HERE, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Retrieve the snapshots that have been created from a Droplet
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.getSnapshotsForDroplet(PUT THE DROPLETID HERE, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Retrieve any backups associated with a Droplet
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.getBackupsForDroplet(PUT THE DROPLETID HERE, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Retrieve all actions that have been executed on a Droplet
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.getActionsForDroplet(PUT THE DROPLETID HERE, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
Retrieve a list of droplets that are scheduled to be upgraded
var DIGITALOCEAN = require('dropletapi').Droplets;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.listDropletUpgrades(function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
List all of the actions that have been executed on the current account
var DIGITALOCEAN = require('dropletapi').Actions;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.listAllActions({page: 1, per_page:1},function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
List all of the actions that have been executed on the current account
var DIGITALOCEAN = require('dropletapi').Actions;
var digitalocean = new DIGITALOCEAN('Your API-TOKEN');
digitalocean.getActionById(YOUR ACTIONS ID, function (error, result) {
if (error) {
console.log(error);
}
else {
console.log(result);
}
});
FAQs
Wrapper for the DigitalOcean API V2
We found that dropletapi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.