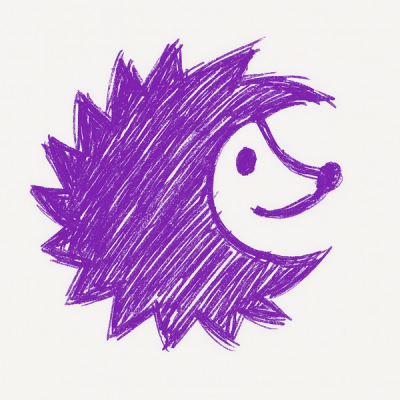
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
dscbots.js
Advanced tools
Goodsie - Helping me with the websocketmanager
dscbots.js is a Node.js module that allows you to interact with the Discord API without any complications.
Node.js 16.9.0 or newer is required.
Installing dscbots.js:
npm install dscbots.js
yarn add dscbots.js
pnpm add dscbots.js
Setup your bot with the following code:
const Dbot = require('dscbots.js')
const bot = new Dbot.BotClient({
intents: [Dbot.Intents.FLAGS.GUILD_MESSAGES, Dbot.Intents.FLAGS.DIRECT_MESSAGES],
token: "<Bot Token>"
})
bot.CreateBot(function () {
console.log(`${bot.user.tag} is ready.`)
});
Afterwards we can create a quite simple message command:
const Dbot = require('dscbots.js')
const bot = new Dbot.BotClient({
intents: [Dbot.Intents.FLAGS.GUILD_MESSAGES, Dbot.Intents.FLAGS.DIRECT_MESSAGES],
token: "<Bot Token>"
})
bot.on('message', (message) => {
if (message.author.isBot) return;
if (message.content === '!hello') {
message.reply("Hi!");
}
})
bot.CreateBot(function () {
console.log(`${bot.user.tag} is ready.`)
});
You can also set your activity!
const Dbot = require('dscbots.js')
const bot = new Dbot.BotClient({
intents: [Dbot.Intents.FLAGS.GUILD_MESSAGES, Dbot.Intents.FLAGS.DIRECT_MESSAGES],
token: "<Bot Token>"
})
bot.CreateBot(function () {
console.log(`${bot.user.tag} is ready.`)
bot.user.setActivity("This server!", "WATCHING");
//Dbot.activities - Returns a list of activities available.
// There is currently 2,5s delay to not cause ratelimit!
});
And you can set the your bot to be on phone.
const Dbot = require('dscbots.js')
const bot = new Dbot.BotClient({
intents: [Dbot.Intents.FLAGS.GUILD_MESSAGES, Dbot.Intents.FLAGS.DIRECT_MESSAGES],
token: "<Bot Token>",
device: Dbot.devices.mobile
// You can also remove this option, it's not required!
})
bot.CreateBot(function () {
console.log(`${bot.user.tag} is ready.`)
bot.user.setActivity("This server!", "WATCHING");
});
For more information go to dscbots.js Server!
If you don't understand something in the package, you are experiencing problems, or you just need a gentle nudge in the right direction, please don't hesitate to join our official dscbots.js Server.
FAQs
Make discord bots with this simple to use Package.
The npm package dscbots.js receives a total of 0 weekly downloads. As such, dscbots.js popularity was classified as not popular.
We found that dscbots.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.