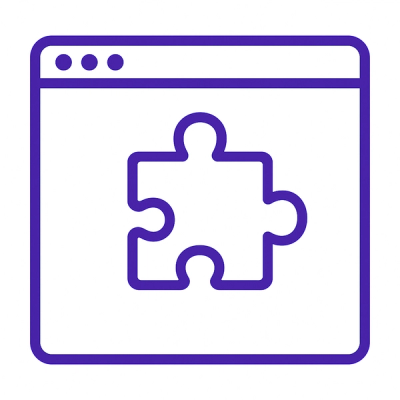
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
dus-ip-geolocation-node-client
Advanced tools
dus-ip-geolocation-node-client
is a Node.js client for interacting with the D.U.S IP GeoLocator Server, a self-hosted solution for IP geolocation. The server uses WHOIS databases from sources like RIPE, APNIC, and others to provide accurate and reliable IP-based geolocation information.
You can install the package via npm:
npm install dus-ip-geolocation-node-client
Here are some examples of how to use the client to retrieve geolocation information for one or more IP addresses.
const DUSIpGeolocationNodeClient = require('dus-ip-geolocation-node-client');
(async () => {
const client = new DUSIpGeolocationNodeClient("http://0.0.0.0:5000/");
// Example 1: Get geolocation for a single IP
const ip = "199.232.38.73";
const result = await client.getGeolocationByIp(ip);
console.log(result);
// Example 2: Get geolocation for multiple IPs
const ips2 = [
"17.248.145.105",
"216.58.209.42",
"18.198.146.59",
"2a03:2880:f203:c5:face:b00c:0:167"
];
const result2 = await client.geolocateMultipleIps(ips2);
console.log(result2);
})().catch((error) => {
console.log(error);
process.exit(1);
});
If you're using TypeScript, you can import and use the client as follows:
import DUSIpGeolocationNodeClient from 'dus-ip-geolocation-node-client';
(async () => {
const client = new DUSIpGeolocationNodeClient("http://0.0.0.0:5000/");
// Example 1: Get geolocation for a single IP
const ip = "199.232.38.73";
const result = await client.getGeolocationByIp(ip);
console.log(result);
// Example 2: Get geolocation for multiple IPs
const ips2 = [
"17.248.145.105",
"216.58.209.42",
"18.198.146.59",
"2a03:2880:f203:c5:face:b00c:0:167"
];
const result2 = await client.geolocateMultipleIps(ips2);
console.log(result2);
})().catch((error) => {
console.log(error);
process.exit(1);
});
getGeolocationByIp
(Single IP){
"asn_name": "FASTLY",
"asn_number": 54113,
"city_name": "Jacksonville",
"country": "United States",
"ip": "199.232.38.73",
"ip_version": 4,
"is_private": false,
"iso_code": "US",
"mnt_by": "Unknown",
"netname": "SKYCA-3",
"status": "valid",
"db_version": "6.0.0"
}
geolocateMultipleIps
(Multiple IPs)[
{
"asn_name": "APPLE-ENGINEERING",
"asn_number": 714,
"city_name": "Unknown",
"country": "United States",
"ip": "17.248.145.105",
"ip_version": 4,
"is_private": false,
"iso_code": "US",
"mnt_by": "Unknown",
"netname": "APPLE-WWNET",
"status": "valid",
"db_version": "6.0.0"
},
{
"asn_name": "GOOGLE",
"asn_number": 15169,
"city_name": "Unknown",
"country": "United States",
"ip": "216.58.209.42",
"ip_version": 4,
"is_private": false,
"iso_code": "US",
"mnt_by": "Unknown",
"netname": "GOOGLE",
"status": "valid",
"db_version": "6.0.0"
},
{
"asn_name": "AMAZON-02",
"asn_number": 16509,
"city_name": "Frankfurt am Main",
"country": "Germany",
"ip": "18.198.146.59",
"ip_version": 4,
"is_private": false,
"iso_code": "DE",
"mnt_by": "Unknown",
"netname": "AMAZO-ZFRA",
"status": "valid",
"db_version": "6.0.0"
},
{
"asn_name": "FACEBOOK",
"asn_number": 32934,
"city_name": "Unknown",
"country": "United States",
"ip": "2a03:2880:f203:c5:face:b00c:0:167",
"ip_version": 6,
"is_private": false,
"iso_code": "US",
"mnt_by": "RIPE-NCC-HM-MNT",
"netname": "IE-FACEBOOK-201100822",
"status": "valid",
"db_version": "6.0.0"
}
]
The D.U.S IP GeoLocator Server (short for "Di Unni Sii IP GeoLocator Server") is a simple, custom, and self-hosted solution for geolocating IP addresses. Built upon the openIPDb, it provides a reliable method for parsing and leveraging IP address data from RIPE, APNIC, and other databases.
This server offers a straightforward yet effective approach to IP geolocation, allowing users to query and obtain location-based information from IP addresses. Whether for analytical purposes, cybersecurity, or enhancing user experiences, the D.U.S IP GeoLocator Server offers a flexible and accessible solution tailored to your geolocation needs.
For more details about the server, including setup instructions and Docker images, visit the D.U.S IP GeoLocator Server repository.
If you'd like to contribute to the project, feel free to open an issue or submit a pull request on GitHub.
This project is released under the MIT License. See the LICENSE
file for more details.
FAQs
Node.js client for DUS IP Geolocation API
The npm package dus-ip-geolocation-node-client receives a total of 3 weekly downloads. As such, dus-ip-geolocation-node-client popularity was classified as not popular.
We found that dus-ip-geolocation-node-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.