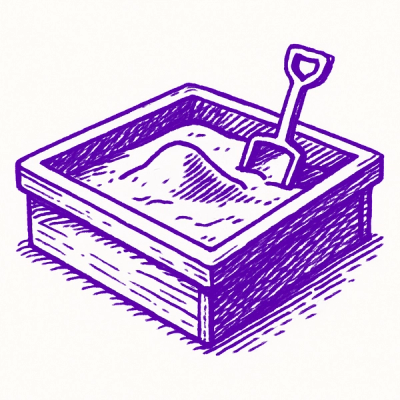
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
The following documents the method to work with EAS attestations via Ethereum Account Abstraction.
Generates 12 words phrase (BIP39) for wallet creation.
static generateMnemonic(): string[]
Derives the private key from the 12-word mnemonic.
static getPrivateKeyFromMnemonic(mnemonic: string[]): string
Derives the Ethereum address from the provided private key.
static getAddressFromPrivateKey(privateKey: string): string
Load the EAS schema to an address.
async registerSchema(): Promise<void>
Creates an abstract account for the wallet using the provided address. Throws an error on fail.
static async createAbstractAccount(address: string): Promise<void>
Prepares an EAS transaction by signing it with the provided private key.
static prepareEASTx(data: any, privateKey: string): string
Prepares a batch of EAS transactions by signing each transaction in the batch.
static prepareEASBatchTx(dataArray: any[], privateKey: string): string
Sends the transaction to the network.
static async sendEASTx(signedTx: string): Promise<string>
Returns the EAS explorer link for the given UID.
static getEASExplorerLink(uid: string): string
async function main() {
// Generate 12 words
const mnemonic: string[] = WalletLibrary.generate12Words()
// Derive private key from mnemonic
const privateKey: string = WalletLibrary.derivePrivateKeyFrom12Words(mnemonic)
// Derive public key and address from private key
const keyInfo = WalletLibrary.derivePublicKeyAndAddress(privateKey)
const address: string = keyInfo.address
// Create abstract account for wallet
await WalletLibrary.createAbstractAccount(address)
// Prepare EAS transaction
const easTransaction: string = WalletLibrary.prepareEASTx({ data: 'sample' }, privateKey)
// Prepare batch of EAS transactions
const easBatchTransaction: string = WalletLibrary.prepareEASBatchTx(
[{ data: 'sample1' }, { data: 'sample2' }],
privateKey
)
// Get EAS explorer link from UID
const explorerLink: string = WalletLibrary.getEASExplorerLink('uid123')
}
You can use the following bash script to register a schema.
Create a schema file and name it schema
with the following content:
uint256 eventId, string[] weights, string comment
The run the following commmand to register it:
./register-schema.js -p ./schema
FAQs
EAS attestations via Ethereum Account Abstraction
The npm package eas-lib receives a total of 0 weekly downloads. As such, eas-lib popularity was classified as not popular.
We found that eas-lib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.