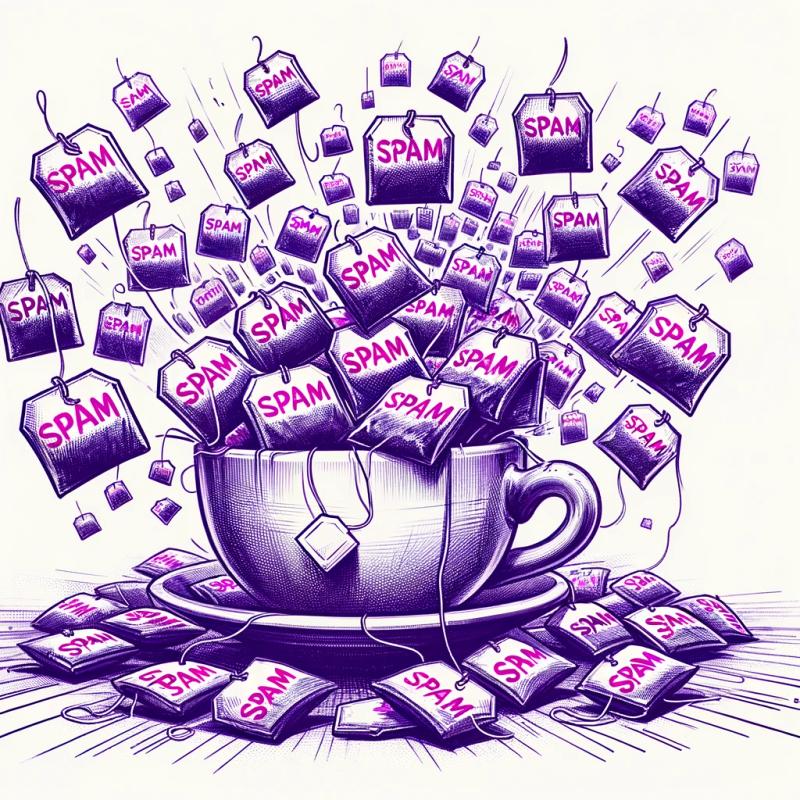
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
easy-ts-camera
Advanced tools
A package which helps with adding camera support to a web application with typescript support
Readme
Version 1.1.2
This package helps you to add camera support to your web application easily and support front and rear camera out of the box.
npm install easy-ts-camera
This package is created based on easy-js-camera
with some improvements and typescript support.
Add your video and canvas as follow,
<video autoplay playsinline></video>
<canvas></canvas>
Import the Camera class as follow,
import ETC from 'easy-ts-camera';
To be able to access to all Video Inputs you first need to get the permission from the user.
Note: On some devices it is not needed to first get the permission but it is better to do that first since on some devices if the permission is not granted it doesn't return all the devices.
var video = document.getElementsByTagName('video')[0];
var canvas = document.getElementsByTagName('canvas')[0];
ETC.initWithUserCamera()
.streamFrom(video)
.drawInto(canvas)
.getCameraAsync()
.then(camera => {
camera.startAsync()
})
.catch(error => {
// Mostly happens if the user blocks the camera or the media devices are not supported
});
Note: In order to make sure that the browser supports MediaDevices you can do as follow.
import { CameraUtils } from 'easy-ts-camera';
CameraUtils.isCameraSupported();
HtmlVideoELement
and HtmlCanvasElement
and also the MediaConstraints
.
4K
resolution if available by the camera. call pick4KResolution
before getting the camera.FullHD
resolution. Please call this method before building camera.MediaDeviceInfo
and if not will log an error.stop
the camera. You can pass the stop
as boolean to prevent this behavior.blob
.front
and rear
. This method returns a promise. It is better that you disable the switch camera button while it is doing its job because on some phones with motorized
selfie camera if the user press the button multiple times the camera will hang and will just stickout and no longer works unless he/she restarts the phone. Please Note that this method accept a boolean which is called tryAgain
and it basically tries to access the rear camera. Sometime the rear camera is not accessible and on catch you can do something like below
function switchCamera(tryAgain = false) {
this.camera.switchAsync(tryAgain)
.catch(() => {
if(tryAgain) return; // This line prevents loops. Because the tryAgain may also fail
this.switchCamera(true);
});
}
FAQs
A package which helps with adding camera support to a web application with typescript support
The npm package easy-ts-camera receives a total of 48 weekly downloads. As such, easy-ts-camera popularity was classified as not popular.
We found that easy-ts-camera demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.