Comparing version 0.0.1 to 0.1.0
89
efgh.js
@@ -1,1 +0,88 @@ | ||
"use strict"; | ||
var escapeHTML = require('lodash.escape'); | ||
var extend = require('extend'); | ||
var pad = require('underscore.string/pad'); | ||
var defaults = { | ||
nodelist: false, | ||
avatarWidth: 140, | ||
messageHTML: false | ||
}; | ||
var dtArrayToString = dtArray => [ | ||
dtArray[0], '-', | ||
pad(dtArray[1], 2, '0'), '-', | ||
pad(dtArray[2], 2, '0'), | ||
' ', | ||
pad(dtArray[3], 2, '0'), ':', | ||
pad(dtArray[4], 2, '0'), ':', | ||
pad(dtArray[5], 2, '0') | ||
].join(''); | ||
var TheSynchronousInterface = settings => { | ||
var options = extend(true, {}, defaults, settings); | ||
if( Array.isArray(options.origTime) ){ | ||
options.origTime = dtArrayToString(options.origTime); | ||
} | ||
if( Array.isArray(options.procTime) ){ | ||
options.procTime = dtArrayToString(options.procTime); | ||
} | ||
// Rows: | ||
// 1) from (with an address and a city), time | ||
// 2) to, time | ||
// 3) (OPTIONAL) FGHI URL | ||
// 4) subject | ||
var rowCount = 4; | ||
if( typeof options.URL === 'undefined' ) rowCount--; | ||
var escapedURL = escapeHTML(options.URL); | ||
return [ | ||
'<table class="table table-bordered table-condensed">', | ||
'<tr>', | ||
'<th rowspan=' + rowCount + ' class="avatar inverse" width=1 ', | ||
'style="background-image: url(', escapeHTML(options.avatarURL), | ||
');">', | ||
'<div style="width: ' + options.avatarWidth + 'px;">', | ||
' ', | ||
'</div>', | ||
'</th>', | ||
'<th class="inverse">From</th>', | ||
'<td>' + escapeHTML(options.from) + '</td>', | ||
'<td>' + escapeHTML(options.origAddr) + '</td>', | ||
'<td width=1><nobr>' + options.origTime + '</nobr></td>', | ||
'</tr>', | ||
'<tr>', | ||
'<th class="inverse">To</th>', | ||
'<td>' + escapeHTML(options.to) + '</td>', | ||
// decoded.toAddr is traditionally ignored outside of netmail: | ||
'<td></td>', //'<td>' + (decoded.toAddr ||'') + '</td>', | ||
'<td width=1><nobr>' + options.procTime + '</nobr></td>', | ||
'</tr>', | ||
( typeof options.URL === 'undefined' ) ? '' : [ | ||
'<tr>', | ||
'<th class="inverse" nowrap>FGHI URL</th>', | ||
'<td colspan=3>', | ||
`<a href="${escapedURL}">${escapedURL}</a>`, | ||
'</td>', | ||
'</tr>' | ||
].join(''), | ||
'<tr>', | ||
'<th class="inverse">Subj</th>', | ||
'<td colspan=3>' + escapeHTML(options.subj) + '</td>', | ||
'</tr>', | ||
( options.messageHTML === false ) ? '</table>' : [ | ||
'<tr><td colspan=5 class="messageText">', | ||
( options.messageHTML === true ) ? '' : ( | ||
`${ options.messageHTML }</td></tr></table>` | ||
) | ||
].join('') | ||
].join(''); | ||
}; | ||
module.exports = { | ||
sync: TheSynchronousInterface | ||
}; |
{ | ||
"name": "efgh", | ||
"main": "efgh.js", | ||
"version": "0.0.1", | ||
"version": "0.1.0", | ||
"description": "Echomail in Fidonet: generator of headers.", | ||
@@ -12,3 +12,12 @@ "keywords": ["Fidonet", "FTN", "echomail", "header", "headers"], | ||
"url": "https://github.com/Mithgol/efgh.git" | ||
}, | ||
"dependencies": { | ||
"extend": "~3.0.0", | ||
"lodash.escape": "~4.0.0", | ||
"underscore.string": "~3.3.4" | ||
}, | ||
"scripts": { | ||
"pretest": "jshint --verbose efgh.js test/", | ||
"test": "mocha --reporter spec" | ||
} | ||
} |
@@ -1,6 +0,89 @@ | ||
# efgh | ||
Echomail in Fidonet: generator of headers. | ||
[](https://npmjs.org/package/efgh) | ||
## Echomail of Fidonet: generator of headers | ||
This module (`efgh`) is a generator of HTML5 representations of headers for Fidonet echomail messages. | ||
This module is written in JavaScript and requires [Node.js](http://nodejs.org/) to run. It uses some ECMAScript 2015 features, and thus a relatively recent Node.js is required. The module is tested against Node.js v4.x and the latest stable Node.js version. | ||
This module is currently in an early phase of its development and thus does not have the desired level of feature completeness. | ||
## Installing EFGH | ||
[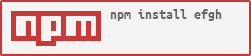](https://npmjs.org/package/efgh) | ||
* Latest packaged version: `npm install efgh` | ||
* Latest githubbed version: `npm install https://github.com/Mithgol/efgh/tarball/master` | ||
You may visit https://github.com/Mithgol/efgh#readme occasionally to read the latest `README` because the package's version is not planned to grow after changes when they happen in `README` only. (And `npm publish --force` is [forbidden](http://blog.npmjs.org/post/77758351673/no-more-npm-publish-f) nowadays.) | ||
## Using EFGH | ||
When you `require()` the installed module, an object is returned. | ||
That object's `sync` property represents the synchronous interface. An asynchronous interface is planned, but not (yet) implemented. | ||
### The synchronous interface | ||
When you `require()` the installed module, an object is returned. Its `sync` property represents the synchronous interface. | ||
That property is a function that accepts an object of settings and returns (synchronously) the corresponding HTML5 representation of a header for a Fidonet echomail message. | ||
The header is returned as a JavaScript string. | ||
An object of settings has the following fields: | ||
*  `nodelist` — by default, `false`. It may also contain an object constructed by the constructor returned from the [Fidonet nodelist](https://github.com/Mithgol/node-fidonet-nodelist) module. In the latter case, the Fidonet nodelist is used to determine the physical location (town, suburb, city, etc.) of the message's sender (using the Fidonet address); that location is displayed in the header. | ||
* `avatarWidth` — width (in CSS pixels) of an avatar of the message's author (or of a default avatar). By default, `140`. This value does not have to be equal to the avatar's width in physical pixels (for example, where `window.devicePixelRatio != 1` in your client-side code). | ||
* `avatarURL` — URL of the avatar (i.e. of the picture). | ||
* `from` — the sender's name. | ||
* `origAddr` — the sender's address. | ||
* `to` — the recipient's name. | ||
* `origTime` — the time when the message was written. Either a string or an array of year, month, day, hour, minute, second. | ||
* `procTime` — the time when the message was processed by an echoprocessor. Either a string or an array of year, month, day, hour, minute, second. | ||
* `subj` — the message's subject line. | ||
* `URL` — [FGHI URL](https://github.com/Mithgol/FGHI-URL/) of the message. | ||
* `messageHTML` — by default, `false`. It means that the HTML5 `table` is closed by its closing tag (`</table>`). | ||
* If `messageHTML === true`, the header (HTML5 `<table>`) is not completed and thus the returned string ends with open `tr` and `td` elements; the message's main text (in HTML) can be added after those elements and ended with `</td></tr></table>` to end the table. The `td` element is given the class `messageText`. | ||
* Also `messageHTML` can be a JavaScript string containing the message's main text (in HTML). In such case the string returned from `.sync()` contains the whole message (not only the header as an HTML table, but also the main text in the last row of the same HTML table). | ||
## CSS rules | ||
Three [Bootstrap](http://getbootstrap.com/) classes ('table', 'table-bordered', 'table-condensed') are assigned to the header's table. | ||
Two additional classes are used for EFGH purposes: | ||
* `inverse` — this class is assigned to individual headers (`From`, `To`, `Subj`, `FGHI URL`) and provides an inverted look (white text on dark background) | ||
* `avatar` — this class is assigned to the `TH` element of the avatar (user's picture) and ensures its proper display (for example, `background-size: contain`). | ||
CSS rules for these two EFGH classes are provided in the file `efgh.css`. Users of EFGH are expected to reference that file or include it in their CSS. | ||
HTML5 headers generated by EFGH may not be displayed properly if HTML5 representation is provided without the corresponding CSS rules (for example, in `description` elements of RSS items). | ||
## Testing EFGH | ||
[](https://travis-ci.org/Mithgol/efgh) | ||
It is necessary to install [Mocha](http://visionmedia.github.io/mocha/) and [JSHint](http://jshint.com/) for testing. | ||
* You may install Mocha globally (`npm install mocha -g`) or locally (`npm install mocha` in the directory of EFGH). | ||
* You may install JSHint globally (`npm install jshint -g`) or locally (`npm install jshint` in the directory of EFGH). | ||
After that you may run `npm test` (in the directory of EFGH). | ||
## License | ||
MIT license (see the `LICENSE` file). |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Empty package
Supply chain riskPackage does not contain any code. It may be removed, is name squatting, or the result of a faulty package publish.
Found 1 instance in 1 package
11296
8
88
89
0
3
+ Addedextend@~3.0.0
+ Addedlodash.escape@~4.0.0
+ Addedunderscore.string@~3.3.4
+ Addedextend@3.0.2(transitive)
+ Addedlodash.escape@4.0.1(transitive)
+ Addedsprintf-js@1.1.3(transitive)
+ Addedunderscore.string@3.3.6(transitive)
+ Addedutil-deprecate@1.0.2(transitive)