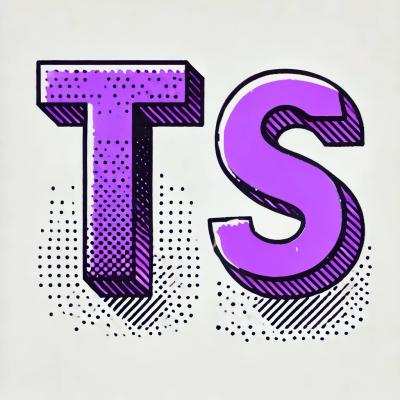
Security News
Node.js Moves Toward Stable TypeScript Support with Amaro 1.0
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
elastic-search-builder
Advanced tools
This lib working with elasticsearch.js, flatten search params and query bodies.
npm install elastic-search-builder --save
/* in ES 5 */
const elasticsearch = require('elasticsearch');
const esb = require('elastic-search-builder');
/* in ES6 */
import elasticsearch from 'elasticsearch';
import esb from 'elastic-search-builder';
/* new elasticsearch client */
const client = new elasticsearch.Client({
host: 'localhost:9200'
});
/* build search params by elastic-search-builder */
const searchParams = esb()
.option()
.indices(['2016.01.01'])
.body()
.query({
match: {
dialogs: 'hello world'
}
}).build();
client.search(searchParams).then(body => {
console.log(body);
})
esb()
.body()
.query()
.bool()
.boolMust({
"term" : { "user" : "kimchy" }
}, {
"term" : { "user" : "blob" }
})
.boolNot([{
"term": { "user": "john" }
},{
"term": { "user": "belly" }
}])
.build();
// {
// body: {
// query: {
// bool: {
// must: [
// {
// "term" : { "user" : "kimchy" }
// },
// {
// "term" : { "user" : "blob" }
// }
// ],
// must_not: [
// {
// "term": { "user": "john" }
// },
// {
// "term": { "user": "belly" }
// }
// ]
// }
// }
// }
// }
esb()
.body()
.aggs()
.appendAggs('all_name', 'terms', {
"field": "name"
})
.subAggs()
.appendAggs('all_gender', 'terms', {
"field": "gender"
})
.subAggs()
.appendAggs('all_city', 'terms', {
"field": "city"
})
.build()
// {
// "aggs": {
// "all_name": {
// "terms": {
// "field": "name"
// },
// "aggs": {
// "all_gender": {
// "terms": {
// "field": "gender"
// },
// "aggs": {
// "all_city": {
// "terms": {
// "field": "city"
// }
// }
// }
// }
// }
// }
// }
// }
build nested aggragation without callback
esb()
.body()
.aggs()
.appendAggs('by_gender', 'terms', {
"field": "gender"
})
.subAggs()
.forkAggs()
.appendAggs('by_city', 'terms', {
"field": "city"
})
.subAggs()
.appendAggs('all_name', 'terms', {
"field": "name"
})
.mergeAggs()
.appendAggs('by_language', 'terms', {
"field": "language"
})
.subAggs()
.appendAggs('all_name', 'terms', {
"field": "name"
})
.build()
// {
// "aggs": {
// "by_gender": {
// "terms": {
// "field": "gender"
// },
// "aggs": {
// "by_city": {
// "terms": {
// "field": "city"
// },
// "aggs": {
// "all_name": {
// "terms": {
// "field": "name"
// }
// }
// }
// },
// "by_language": {
// "terms": {
// "field": "language"
// },
// "aggs": {
// "all_name": {
// "terms": {
// "field": "name"
// }
// }
// }
// }
// }
// }
// }
}
npm run dev
npm run test
npm run build:docs
npm login
npm publish
FAQs
An elasticsearch search option builder.
The npm package elastic-search-builder receives a total of 0 weekly downloads. As such, elastic-search-builder popularity was classified as not popular.
We found that elastic-search-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.