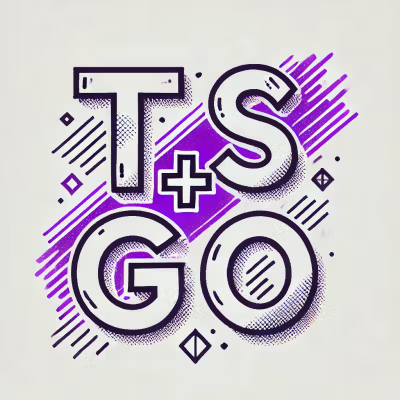
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Tiny chainable DOM manipulation lib.
npm i el.js
Or just download index.js
.
Add El.js to your project:
<script src="path/el.js"></script>
Create an instance for the element you want to manipulate, for example,
let's grab a button with the id coolButton
, which is rendered in hidden
state with the style display: none
, make it visible and disable it when clicked:
const $coolButton = new El('#coolButton')
$coolButton
.show()
.on('click', () => $coolButton.disable())
Once you created an instance you can chain API methods if you need to do multiple manipulations of your element.
For example:
const $title = new El('#title')
$title
.html('Alice in Chains rocks!')
.addClass('cool')
.show()
constructor(element)
exists()
get()
show()
showInline()
hide()
disable()
enable()
style(styleName, value)
addClass(className)
removeClass(className)
remove()
setRandomBackground({path = '', range = [0, 5], length = 3, ext = 'jpg'})
appear()
disappear()
focus()
html(value)
appendHtml(value)
prependHtml(value)
val(value)
clear()
isVisible()
toggle()
caretEnd()
src(value)
scrollBottom()
on(event, callback)
static injectStyles(styles)
constructor (element)
Where element
can be any valid CSS selector or a reference to an element.
For the HTML:
<ul class="user-list">
...
</ul>
It's valid to construct an instance with:
const $userList = new El('.user-list')
And:
const userListElement = document.querySelector('.user-list')
const $userList = new El(userListElement)
$myEl.exists()
Returns a boolean.
$myEl.get()
$myEl.show()
Works with elements hidden with display: none
by switching to display: block
.
$myEl.showInline()
Works with elements hidden with display: none
by switching to display: inline
.
$myEl.hide
Hides an element using display: none
.
$myEl.disable()
Sets disabled
attribute to true
.
$myEl.disable()
Sets disabled
attribute to false
.
$myEl.style()
Returns styles object containing all styles set on element.
$myEl.style('color')
Returns requested style value.
$myEl.style('color', 'black')
Sets passed style attribute with passed value.
$myEl.attr('data-id')
Returns value for attribute data-id
.
$myEl.attr('data-id', 42)
Sets attribute data-id
to 42
.
$myEl.attr('data-id', false)
Removes attribute data-id
from element.
$myEl.addClass('active')
Appends passed class to element's className
attribute.
$myEl.removeClass('active')
Removes passed class from element's className
attribute.
$myEl.remove()
$myEl.setRandomBackground({
path: 'some/path',
range: [0, 10],
length: 3,
ext: 'jpg'
})
Sets a background image with the following format:
url(${path}/${name}.${ext})
Where:
${path}
is the given path
.${name}
is a random number in the given range with the specified length.${ext}
is the file extension, as passed on ext
.Default values:
[0, 5]
3
jpg
If we have the following files:
img/00100.jpg
img/00101.jpg
img/00102.jpg
img/00103.jpg
img/00104.jpg
img/00105.jpg
To randomly select a background image we should:
$myEl.setRandomBackground({
path: 'img',
range: [100, 105],
length: 5
})
$myEl.appear()
Sets opacity
to 1
.
$myEl.disappear()
Sets opacity
to 0
.
$myEl.focus()
$myEl.html('<strong>Rock it!</strong>')
$myEl.html()
$myEl.appendHtml('<i>Pizza!</i>')
Adds passed HTML content at the end of the element.
$myEl.prependHtml('<b>Thanks to:</b>')
Adds passed HTML content at the beggining of the element.
$myEl.val(42)
Sets passed value as element's value.
Applies for inputs and textareas, but you can set a value on any element even if it's not rendered.
$myEl.val()
Returns current element's value.
$myEl.clear()
Sets element's value to an empty string.
$myEl.isVisible()
Returns true if the element's display
CSS attribute is not set or one of:
$myEl.toggle()
Uses $myEl.isVisible()
to detect visibility.
Calls $myEl.show()
when element is hidden.
Calls $myEl.hide()
when element is visible.
$myEl.caretEnd()
$myEl.src('img/groovy.jpg')
Sets passed value on the src
attribute.
$myEl.src()
Returns current src
value.
$myEl.scrollBottom()
Moves scroll position to the bottom of the element.
$myEl.on('click', () => console.log('clicked!'))
Listen to element events passing a callback.
El.injectStyles(styles)
Note that this is a static method that's called directly on the El
object.
Example:
El.injectStyles(`
body {
background: black;
color: white;
}
h1 {
font-size: 48px;
}
`)
FAQs
Tiny chainable DOM manipulation lib.
The npm package eldo receives a total of 0 weekly downloads. As such, eldo popularity was classified as not popular.
We found that eldo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.