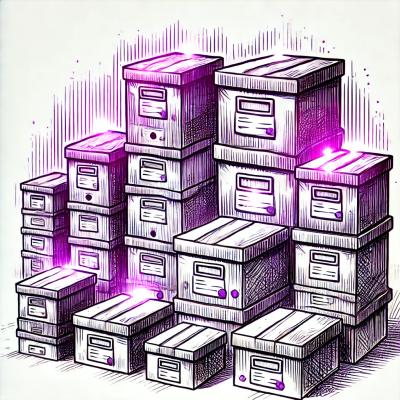
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
electron-updater
Advanced tools
The electron-updater npm package provides a way to automatically update Electron applications. It integrates with the Electron framework to enable seamless updates of desktop applications built with Electron. The package allows for easy distribution of updates by checking for new versions and downloading them in the background.
Auto Update
This feature allows the application to automatically check for updates and notify the user when an update is available. It can also download and install the updates in the background.
const { autoUpdater } = require('electron-updater');
autoUpdater.checkForUpdatesAndNotify();
Update Events
This feature allows developers to listen for specific events during the update process, such as when an update is available or when it has been downloaded. Developers can then take appropriate actions, like prompting the user or automatically restarting the application.
const { autoUpdater } = require('electron-updater');
autoUpdater.on('update-available', (info) => {
console.log('Update available.');
});
autoUpdater.on('update-downloaded', (info) => {
console.log('Update downloaded; will install in 5 seconds');
setTimeout(() => {
autoUpdater.quitAndInstall();
}, 5000);
});
Manual Update Checks
This feature allows developers to manually trigger an update check instead of relying on automatic checks. This can be useful for giving users the option to check for updates on demand.
const { autoUpdater } = require('electron-updater');
autoUpdater.checkForUpdates();
A lightweight updater for Electron apps that works with GitHub Releases. It is simpler than electron-updater but does not provide as many configuration options or features such as differential updates.
A smart release server for Electron apps that uses GitHub as a backend for serving updates. It is not an npm package but a server that you can deploy. It provides similar functionality but requires more setup compared to electron-updater.
Squirrel is a set of tools for installing and updating Windows and macOS applications. While not an npm package, it is often used in conjunction with Electron to provide update functionality. It is more complex and provides more control over the update process compared to electron-updater.
This module allows you to automatically update your application. You only need to install this module and write two lines of code! To publish your updates you just need simple file hosting, it does not require a dedicated server.
See Auto Update for more information.
Supported OS:
Thanks to Evolve Labs for donating the npm package name.
FAQs
Cross platform updater for electron applications
The npm package electron-updater receives a total of 192,744 weekly downloads. As such, electron-updater popularity was classified as popular.
We found that electron-updater demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.