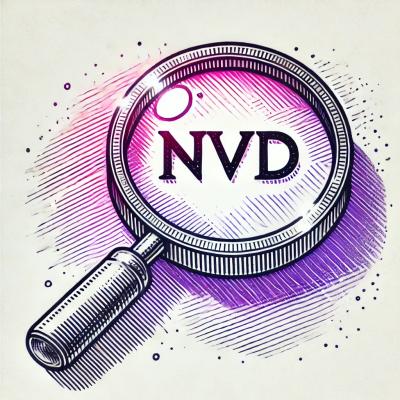
Security News
NIST Under Federal Audit for NVD Processing Backlog and Delays
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
elevenlabs-node
Advanced tools
This is an open source Eleven Labs NodeJS package for converting text to speech using the Eleven Labs API
Eleven Labs NodeJS package for converting text to speech!
Drop us a ⭐ on GitHub to help the project improve!
This is an open source Eleven Labs NodeJS package for converting text to speech using the Eleven Labs API.
Function | Parameters | Endpoint |
---|---|---|
textToSpeech | ({voiceId, fileName, textInput, stability, similarityBoost, modelId, style, speakerBoost}) | /v1/text-to-speech/{voice_id} |
textToSpeechStream | ({voiceId, textInput, stability, similarityBoost, modelId, responseType, style, speakerBoost}) | /v1/text-to-speech/{voice_id}/stream |
editVoiceSettings | ({voiceId, stability, similarityBoost}) | /v1/voices/{voice_id}/settings/edit |
getVoiceSettings | ({voiceId}) | /v1/voices/{voice_id}/settings |
deleteVoice | ({voiceId}) | /v1/voices/{voice_id} |
getVoice | ({voiceId}) | /v1/voices/{voice_id} |
getVoices | N/A | /v1/voices |
getModels | N/A | /v1/models |
getUserInfo | N/A | /v1/user |
getUserSubscription | N/A | /v1/user/subscription |
getDefaultVoiceSettings | N/A | /v1/voices/settings/default |
Variable | Description | Type |
---|---|---|
fileName | Name and file path for your audio file e.g (./gen/hello ) | String |
textInput | Text to be converted into audio e.g (Hello ) | String |
stability | Stability for Text to Speech default (0 ) | Float |
similarityBoost | Similarity Boost for Text to Speech default (0 ) | Float |
voiceId | ElevenLabs Voice ID e.g (pNInz6obpgDQGcFmaJgB ) | String |
modelId | ElevenLabs Model ID e.g (eleven_multilingual_v2 ) | String |
responseType | Streaming response type e.g (stream ) | String |
speakerBoost | Speaker Boost for Text to Speech e.g (true ) | Boolean |
style | Style Exaggeration for Text to Speech (0-100) default (0 ) | Integer |
To install the Elevenlabs package, run the following command:
npm install elevenlabs-node
Setup the ElevenLabs configurations for your project.
Variable | Description | Default |
---|---|---|
apiKey | (Required ) Your API key from Elevenlabs | N/A |
voiceId | (Optional ) A Voice ID from Elevenlabs | Adam (pNInz6obpgDQGcFmaJgB ) |
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
voiceId: "pNInz6obpgDQGcFmaJgB", // A Voice ID from Elevenlabs
}
);
Generating an audio file from text.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
voiceId: "pNInz6obpgDQGcFmaJgB", // A Voice ID from Elevenlabs
}
);
voice.textToSpeech({
// Required Parameters
fileName: "audio.mp3", // The name of your audio file
textInput: "mozzy is cool", // The text you wish to convert to speech
// Optional Parameters
voiceId: "21m00Tcm4TlvDq8ikWAM", // A different Voice ID from the default
stability: 0.5, // The stability for the converted speech
similarityBoost: 0.5, // The similarity boost for the converted speech
modelId: "eleven_multilingual_v2", // The ElevenLabs Model ID
style: 1, // The style exaggeration for the converted speech
speakerBoost: true // The speaker boost for the converted speech
}).then((res) => {
console.log(res);
});
Generating an audio stream from text.
const ElevenLabs = require("elevenlabs-node");
const fs = require("fs-extra");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
voiceId: "pNInz6obpgDQGcFmaJgB", // A Voice ID from Elevenlabs
}
);
const voiceResponse = voice.textToSpeechStream({
// Required Parameters
textInput: "mozzy is cool", // The text you wish to convert to speech
// Optional Parameters
voiceId: "21m00Tcm4TlvDq8ikWAM", // A different Voice ID from the default
stability: 0.5, // The stability for the converted speech
similarityBoost: 0.5, // The similarity boost for the converted speech
modelId: "eleven_multilingual_v2", // The ElevenLabs Model ID
style: 1, // The style exaggeration for the converted speech
responseType: "stream", // The streaming type (arraybuffer, stream, json)
speakerBoost: true // The speaker boost for the converted speech
}).then((res) => {
res.pipe(fs.createWriteStream(fileName));
});
Editing voice settings.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.editVoiceSettings({
// Required Parameters
voiceId: "pNInz6obpgDQGcFmaJgB", // The ID of the voice you want to edit
stabilityBoost: 0.5, // The Stability Boost for the voice
similarityBoost: 0.5, // The Similarity Boost for the voice
}).then((res) => {
console.log(res);
});
Getting voice settings.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getVoiceSettings({
// Required Parameters
voiceId: "pNInz6obpgDQGcFmaJgB" // The ID of the voice you want to get
}).then((res) => {
console.log(res);
});
Delete voice.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.deleteVoice({
// Required Parameters
voiceId: "pNInz6obpgDQGcFmaJgB" // The ID of the voice you want to delete
}).then((res) => {
console.log(res);
});
Getting voice details.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getVoice({
// Required Parameters
voiceId: "pNInz6obpgDQGcFmaJgB" // The ID of the voice you want to get
}).then((res) => {
console.log(res);
});
Getting all voice details.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getVoices().then((res) => {
console.log(res);
});
Getting all model details.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getModels().then((res) => {
console.log(res);
});
Getting user info associated with the API Key.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getUserInfo().then((res) => {
console.log(res);
});
Getting user subscription info associated with the API Key.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getUserSubscription().then((res) => {
console.log(res);
});
Getting default voice settings.
const ElevenLabs = require("elevenlabs-node");
const voice = new ElevenLabs(
{
apiKey: "0e2c037kl8561005671b1de345s8765c", // Your API key from Elevenlabs
}
);
const voiceResponse = voice.getDefaultVoiceSettings().then((res) => {
console.log(res);
});
Contributions are welcome :)
Read our CONTRIBUTING.md to learn more.
FAQs
This is an open source Eleven Labs NodeJS package for converting text to speech using the Eleven Labs API
The npm package elevenlabs-node receives a total of 1,846 weekly downloads. As such, elevenlabs-node popularity was classified as popular.
We found that elevenlabs-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.
Security News
TypeScript Native Previews offers a 10x faster Go-based compiler, now available on npm for public testing with early editor and language support.