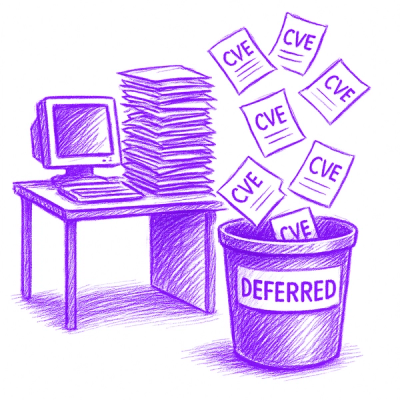
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
ember-cli-dependency-injection-utils
Advanced tools
A utility library for more concise dependency injection through Ember initializers.
# ember-cli > 0.2.3
ember install ember-cli-dependency-injection-utils
# ember-cli <= 0.2.3
ember install:addon ember-cli-dependency-injection-utils
Below is a sample initializer file/pattern you might create. For additional info, please check out the source.
Simple example
// app/initializers/dependency-injection.js
import { injectThroughout, injectDeps } from 'ember-cli-dependency-injection-utils';
export function initialize(application) {
// Inject factory dependencies onto a specific container
injectDeps(application, 'route', [
{ property: 'api', injectionName: 'service:some-api-client' },
{ property: 'foo', injectionName: 'service:foo-service-you-want-on-every-route' }
]);
// Inject a service to in common Ember places: route, controller, view, component
injectThroughout(application, 'service:some-ga-analytics-you-need-everywhere');
}
export default { name: 'dependency-injection', initialize };
DRY, real world usage example
// app/initializers/dependency-injection.js
import { injectThroughout, injectDeps } from 'ember-cli-dependency-injection-utils';
export function initialize(application) {
// Register anything you need
application.register('config:environment', environment, { singleton: false, instantiate: false });
// Service definitions
const environment = { property: 'environment', injectionName: 'config:environment' };
const routing = { property: 'routing', injectionName: 'service:-routing' };
const site = { property: 'site', injectionName: 'service:site' };
const session = { property: 'session', injectionName: 'service:session' };
const api = { property: 'api', injectionName: 'service:some-api-client' };
// Inject factory dependencies, using service definitions
injectDeps(application, 'service:site', [environment]);
injectDeps(application, 'service:some-api-client', [routing, site, session]);
injectDeps(application, 'route', [api]);
// Inject these services in common Ember places
injectThroughout(application, 'service:site');
injectThroughout(application, 'service:session');
injectThroughout(application, 'service:some-ga-analytics');
injectThroughout(application, 'service:any-service-you-need-everywhere');
}
export default { name: 'dependency-injection', initialize };
README
.FAQs
The default blueprint for ember-cli addons.
The npm package ember-cli-dependency-injection-utils receives a total of 0 weekly downloads. As such, ember-cli-dependency-injection-utils popularity was classified as not popular.
We found that ember-cli-dependency-injection-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.