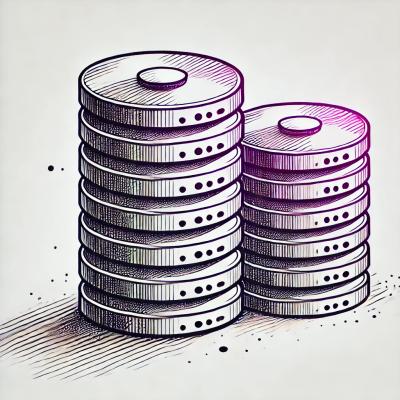
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
ember-router-generator
Advanced tools
MicroLib to add or remove routes from your Ember.js router.
The ember-router-generator npm package is a utility for programmatically manipulating Ember.js router files. It allows developers to add, remove, and modify routes in an Ember.js application with ease.
Add Route
This feature allows you to add a new route to the Ember.js router file. The `addRoute` function takes the path to the router file and an object representing the new route, and returns the updated router content.
const { addRoute } = require('ember-router-generator');
const routerFile = 'app/router.js';
const newRoute = { name: 'about', path: '/about' };
const updatedRouter = addRoute(routerFile, newRoute);
console.log(updatedRouter);
Remove Route
This feature allows you to remove an existing route from the Ember.js router file. The `removeRoute` function takes the path to the router file and the name of the route to be removed, and returns the updated router content.
const { removeRoute } = require('ember-router-generator');
const routerFile = 'app/router.js';
const routeName = 'about';
const updatedRouter = removeRoute(routerFile, routeName);
console.log(updatedRouter);
Modify Route
This feature allows you to modify an existing route in the Ember.js router file. The `modifyRoute` function takes the path to the router file, the name of the route to be modified, and an object representing the new route, and returns the updated router content.
const { modifyRoute } = require('ember-router-generator');
const routerFile = 'app/router.js';
const routeName = 'about';
const newRoute = { name: 'about-us', path: '/about-us' };
const updatedRouter = modifyRoute(routerFile, routeName, newRoute);
console.log(updatedRouter);
Ember CLI is the command line interface for managing Ember.js applications. It provides a wide range of functionalities including generating routes, components, and more. While it offers route generation, it does not provide the same level of programmatic manipulation of the router file as ember-router-generator.
Broccoli is a build tool for JavaScript applications that can be used with Ember.js. It allows for the manipulation of files and directories, but it does not specifically focus on the Ember.js router file like ember-router-generator does.
2.0.0
class
and decorators) in the app/router.js
fileFAQs
MicroLib to add or remove routes from your Ember.js router.
The npm package ember-router-generator receives a total of 175,083 weekly downloads. As such, ember-router-generator popularity was classified as popular.
We found that ember-router-generator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.