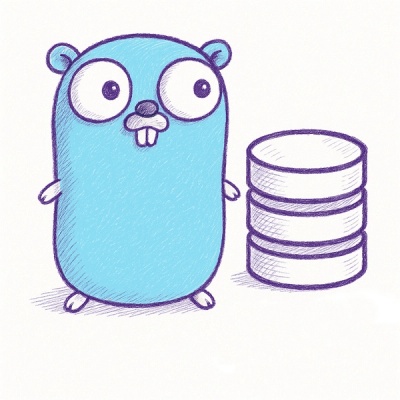
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
emogeez-parser
Advanced tools
This module helps you parsing emojis in text and find emojis to replace it with the emoji name, or utf8 or image.
It works with emogeez-generator data generated. You can either use the fetchTheme
to fetch the JSON file containing the emojis data from the opensource cdn jsdeliver,
https://cdn.jsdelivr.net/gh/arthur-feral/emogeez@latest/packages/emogeez-generator/emojis/apple/apple.png
or setTheme
passing your data if you want to use a custom json. :warning: the, JSON data must be properly formated as the generator whould do if you use custom json.
$ yarn i emogeez-parser
Once installed, the different tools can be imported in your projects:
First configure the package for your personal use.
It require an url where to get the resources generated by the package emogeez-generator.
After running the emogeez-generator
package, the generated files can be hosted by you or you can use the default generated hosted by the repository.
import parserFactory from 'emogeez-parser';
const {
store,
replacer,
matcher,
} = parserFactory();
store.fetchTheme('apple')
.then(() => {
let text = 'Hello ! :) how are you π';
const hasOnlyOneEmoji = matcher.hasOnlyOneEmoji('apple', text);
// => hasOnlyOneEmoji = false
const hasOnlyEmojis = matcher.hasOnlyEmojis('apple', text);
// => hasOnlyEmojis = false
text = replacer.toUTF8('apple', text);
// text = Hello ! π how are you π
text = replacer.UTF8ToHTML('apple', text, (emoji) => {
return `<%${emoji.name}%>`;
});
// text = Hello ! <%grinning-face%> how are you <%smiling-face-with-smiling-eyes%>
});
When you initialize the library you can provide a configuration.
####blackList {array}
The names you want to blacklist. When initialized, it will not store them, so they will not be matched in any use.
Default []
####theme {string}
The default thème.
Default apple
####themesUrl {string}
The url when all emojis themes are hosted.
Default the emogeez-generator repository: https://cdn.jsdelivr.net/gh/arthur-feral/emogeez@latest/packages/emogeez-generator/emojis/apple/apple.json
It stores the emojis data. You can use it to load more themes
It fetch theme from cdn or the url you provide arguments
theme
{string} the theme name check the emogeez-generator for full listdata
{object} the json containing emojis data for the themeYou set your theme manually same args as fetchTheme
The tools provided by replacer
should be used to replace emojis with what you want.
It will replace all aliases to the emoji name.
arguments
text
{string} the text in which we want replace alias to namereturns {string}
const text = 'Hello :)';
replacer.aliasesToNames('apple', text);
-> 'Hello :slightly-smiling-face:'
It will replace all emojis name :emoji-name:
to the utf8 char.
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to replacereturns {string}
const text = 'Hello :slightly-smiling-face:';
replacer.toUTF8('apple', text);
-> 'Hello π'
It will replace all utf8 char to the emoji name.
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to replacereturns {string}
const text = 'Hello π';
replacer.utf8ToNames('apple', text);
-> 'Hello :slightly-smiling-face:'
It will replace all names to an HTML element. You can use the classname generated by the emogeez-generator to display the sprite.
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to replaceHTMLRenderer
{function} The function returning the html. It will provide the matched emoji data to be used in your renderer.returns {string}
const text = 'Hello :slightly-smiling-face:';
replacer.namesToHTML('apple', text, (emoji) => {
return `<span class="emoji-${emoji.name}"></span>`;
});
-> 'Hello <span class="emoji-slightly-smiling-face"></span>'
It will replace all utf8 char to an HTML element. You can use the classname generated by the emogeez-generator to display the sprite.
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to replaceHTMLRenderer
{function} The function returning the html. It will provide the matched emoji data to be used in your renderer.returns {string}
const text = 'Hello π';
replacer.UTF8ToHTML('apple', text, (emoji) => {
return `<span class="emoji-${emoji.name}"></span>`;
});
-> 'Hello <span class="emoji-slightly-smiling-face"></span>'
The tools provided by matcher
should be used to find or match emojis in text.
If you want to check if there is emojis in a text.
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to find if there is emojisreturns {boolean}
Find the list of emojis's names in the text
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to find if there is emojisreturns {array}
Return true if the string contains only emojis in it
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to find if there is emojisreturns {boolean}
Return true if the string contains only one emoji in it
arguments
theme
{string} the theme for what we want to match emojistext
{string} the text in which we want to find if there is emojisreturns {boolean}
Please contribute if you found it useful! β€οΈ
return 'enjoy';
FAQs
A toolbox for parsing emojis in texts.
We found that emogeez-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.