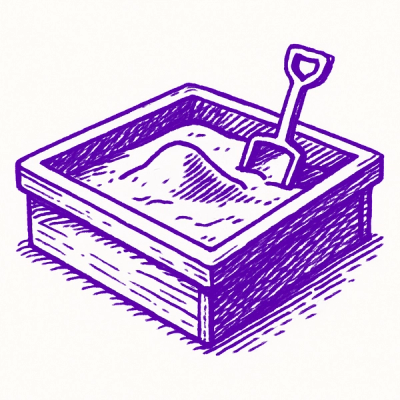
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
The env-cmd npm package allows you to easily set environment variables from a file or inline before running a command. This is particularly useful for managing different environments (development, testing, production) without hardcoding environment variables in your code.
Load environment variables from a file
This feature allows you to load environment variables from a specified file before running your command. The file should follow the standard .env format.
env-cmd -f ./path/to/.env node app.js
Load environment variables from multiple files
You can load environment variables from multiple files. This is useful for layering configurations, such as having a base configuration and an environment-specific override.
env-cmd -f ./path/to/.env -f ./path/to/.env.local node app.js
Inline environment variables
This feature allows you to set environment variables inline directly in the command. This is useful for quick tests or temporary overrides.
env-cmd -e 'NODE_ENV=production' node app.js
JSON configuration
You can also pass environment variables as a JSON object. This is useful for more complex configurations that might be easier to manage in JSON format.
env-cmd -e '{"NODE_ENV": "production", "API_KEY": "12345"}' node app.js
dotenv is a popular package for loading environment variables from a .env file into process.env. Unlike env-cmd, dotenv is typically used by requiring it in your code, rather than as a command-line tool.
cross-env allows you to set environment variables across different platforms (Windows, Linux, macOS) in a consistent manner. It is similar to env-cmd but focuses more on cross-platform compatibility.
dotenv-cli is a command-line interface for dotenv, allowing you to load environment variables from a .env file before running a command, similar to env-cmd. It provides a simpler interface but lacks some of the advanced features of env-cmd.
A simple node program for executing commands using an environment from an env file.
npm install env-cmd
or npm install -g env-cmd
Environment file ./.env
# This is a comment
ENV1=THANKS
ENV2=FOR ALL
ENV3=THE FISH
Package.json
{
"scripts": {
"test": "env-cmd mocha -R spec"
}
}
Terminal
./node_modules/.bin/env-cmd node index.js
To use a custom env filename or path, pass the -f
flag. This is a major breaking change from prior versions < 9.0.0
Terminal
./node_modules/.bin/env-cmd -f ./custom/path/.env node index.js
Usage: _ [options] <command> [...args]
Options:
-v, --version output the version number
-e, --environments [env1,env2,...] The rc file environment(s) to use
-f, --file [path] Custom env file path (default path: ./.env)
--fallback Fallback to default env file path, if custom env file path not found
--no-override Do not override existing environment variables
-r, --rc-file [path] Custom rc file path (default path: ./.env-cmdrc(|.js|.json)
--silent Ignore any env-cmd errors and only fail on executed program failure.
--use-shell Execute the command in a new shell with the given environment
--verbose Print helpful debugging information
-x, --expand-envs Replace $var in args and command with environment variables
-h, --help output usage information
.rc
file usageFor more complex projects, a .env-cmdrc
file can be defined in the root directory and supports
as many environments as you want. Simply use the -e
flag and provide which environments you wish to
use from the .env-cmdrc
file. Using multiple environment names will merge the environment variables
together. Later environments overwrite earlier ones in the list if conflicting environment variables
are found.
.rc file ./.env-cmdrc
{
"development": {
"ENV1": "Thanks",
"ENV2": "For All"
},
"test": {
"ENV1": "No Thanks",
"ENV3": "!"
},
"production": {
"ENV1": "The Fish"
}
}
Terminal
./node_modules/.bin/env-cmd -e production node index.js
# Or for multiple environments (where `production` vars override `test` vars,
# but both are included)
./node_modules/.bin/env-cmd -e test,production node index.js
--no-override
optionPrevents overriding of existing environment variables on process.env
and within the current
environment.
--fallback
file usage optionIf the .env
file does not exist at the provided custom path, then use the default
fallback location ./.env
env file instead.
--use-shell
Executes the command within a new shell environment. This is useful if you want to string multiple commands together that share the same environment variables.
Terminal
./node_modules/.bin/env-cmd -f ./test/.env --use-shell "npm run lint && npm test"
EnvCmd supports reading from asynchronous .env
files. Instead of using a .env
file, pass in a .js
file that exports either an object or a Promise
resolving to an object ({ ENV_VAR_NAME: value, ... }
). Asynchronous .rc
files are also supported using .js
file extension and resolving to an object with top level environment
names ({ production: { ENV_VAR_NAME: value, ... } }
).
Terminal
./node_modules/.bin/env-cmd -f ./async-file.js node index.js
-x
expands vars in argumentsEnvCmd supports expanding $var
values passed in as arguments to the command. The allows a user
to provide arguments to a command that are based on environment variable values at runtime.
Terminal
# $USER will be expanded into the env value it contains at runtime
./node_modules/.bin/env-cmd -x node index.js --user=$USER
--silent
suppresses env-cmd errorsEnvCmd supports the --silent
flag the suppresses all errors generated by env-cmd
while leaving errors generated by the child process and cli signals still usable. This
flag is primarily used to allow env-cmd
to run in environments where the .env
file might not be present, but still execute the child process without failing
due to a missing file.
You can find examples of how to use the various options above by visiting the examples repo env-cmd-examples.
These are the currently accepted environment file formats. If any other formats are desired please create an issue.
.env
as key=value
.env.json
Key/value pairs as JSON.env.js
JavaScript file exporting an object
or a Promise
that resolves to an object
.env-cmdrc
as valid json or .env-cmdrc.json
in execution directory with at least one environment { "dev": { "key1": "val1" } }
.env-cmdrc.js
JavaScript file exporting an object
or a Promise
that resolves to an object
that contains at least one environmentThis lib attempts to follow standard bash
path rules. The rules are as followed:
Home Directory = /Users/test
Working Directory = /Users/test/Development/app
Type | Input Path | Expanded Path |
---|---|---|
Absolute | /some/absolute/path.env | /some/absolute/path.env |
Home Directory with ~ | ~/starts/on/homedir/path.env | /Users/test/starts/on/homedir/path.env |
Relative | ./some/relative/path.env or some/relative/path.env | /Users/test/Development/app/some/relative/path.env |
Relative with parent dir | ../some/relative/path.env | /Users/test/Development/some/relative/path.env |
EnvCmd
A function that executes a given command in a new child process with the given environment and options
options
{ object
}
command
{ string
}: The command to execute (node
, mocha
, ...)commandArgs
{ string[]
}: List of arguments to pass to the command
(['-R', 'Spec']
)envFile
{ object
}
filePath
{ string
}: Custom path to .env file to read from (defaults to: ./.env
)fallback
{ boolean
}: Should fall back to default ./.env
file if custom path does not existrc
{ object
}
environments
{ string[]
}: List of environment to read from the .rc
filefilePath
{ string
}: Custom path to the .rc
file (defaults to: ./.env-cmdrc(|.js|.json)
)options
{ object
}
expandEnvs
{ boolean
}: Expand $var
values passed to commandArgs
(default: false
)noOverride
{ boolean
}: Prevent .env
file vars from overriding existing process.env
vars (default: false
)silent
{ boolean
}: Ignore any errors thrown by env-cmd, used to ignore missing file errors (default: false
)useShell
{ boolean
}: Runs command inside a new shell instance (default: false
)verbose
{ boolean
}: Prints extra debug logs to console.info
(default: false
)Promise<object>
}: key is env var name and value is the env var valueGetEnvVars
A function that parses environment variables from a .env
or a .rc
file
options
{ object
}
envFile
{ object
}
filePath
{ string
}: Custom path to .env file to read from (defaults to: ./.env
)fallback
{ boolean
}: Should fall back to default ./.env
file if custom path does not existrc
{ object
}
environments
{ string[]
}: List of environment to read from the .rc
filefilePath
{ string
}: Custom path to the .rc
file (defaults to: ./.env-cmdrc(|.js|.json)
)verbose
{ boolean
}: Prints extra debug logs to console.info
(default: false
)Promise<object>
}: key is env var name and value is the env var valueBecause sometimes it is just too cumbersome passing a lot of environment variables to scripts. It is usually just easier to have a file with all the vars in them, especially for development and testing.
🚨Do not commit sensitive environment data to a public git repo! 🚨
cross-env
- Cross platform setting of environment scripts
Special thanks to cross-env
for inspiration (uses the
same cross-spawn
lib underneath too).
I welcome all pull requests. Please make sure you add appropriate test cases for any features added. Before opening a PR please make sure to run the following scripts:
npm run lint
checks for code errors and format according to ts-standardnpm test
make sure all tests passnpm run test-cover
make sure the coverage has not decreased from current master10.1.0
-x
flag.
Note: only supports $var
syntax--silent
flag that ignores env-cmd errors and missing files and
only terminates on caught signals--verbose
flag that prints additional debugging info to console.info
commander
to 4.x
sinon
, nyc
, and ts-standard
FAQs
Executes a command using the environment variables in an env file
The npm package env-cmd receives a total of 1,196,532 weekly downloads. As such, env-cmd popularity was classified as popular.
We found that env-cmd demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.