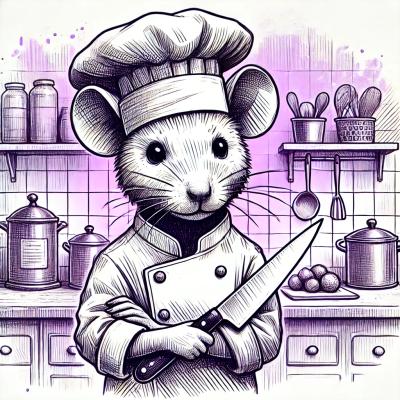
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
A facade for side-effects: write test-friendly, async/await-style, JS code.
Care about unit testing around side-effects? You may fall in love with Episode 7.
Inspired by the test-friendly generator architecture of redux-saga
and the pure side-effects of Elm.
ES6/Generator support.
npm install episode-7 --save
See: CHANGELOG
const Episode7 = require('episode-7');
const fetch = require('node-fetch');
// Compose an Episode 7 Generator for an async call sequence.
//
function* findFirstMovie(searchTerm) {
// Wrap side-effects with Episode 7's `call`
//
let results = yield Episode7.call(
fetchJson,
`http://www.omdbapi.com/?s=${encodeURIComponent(searchTerm)}`
);
// Do something programmatic with the result.
//
let firstResultId = results.Search[0].imdbID;
// Use that transformed value to make the next call.
//
let movie = yield Episode7.call(
fetchJson,
`http://www.omdbapi.com/?i=${encodeURIComponent(firstResultId)}`
);
return movie;
}
// Side-effect function,
// a barebones helper to fetch & decode JSON.
//
function fetchJson(url) {
return fetch(url).then( response => response.json() );
}
// Run the generator with Episode 7, returning a promise.
//
Episode7.run(findFirstMovie, 'Episode 7')
.then( movie => console.log(movie.Title) )
.catch( error => console.error(error) )
catch
after Episode7.run
to handle errors from the async flow (log|exit|retry).
Avoid catching within side-effect functions. Allow their errors to bubble up.
Declare generator functions as named function expressions; this makes stacktraces & error messages more meaningful.
I created this module to make it easy to code & test sequences of web service API requests interleaved with programmatic logic. I'm pulling the essence of redux-saga
into the smallest, plainest surface area conceivable.
FAQs
Sequence async side-effects with ES6 generators and easily test them.
The npm package episode-7 receives a total of 0 weekly downloads. As such, episode-7 popularity was classified as not popular.
We found that episode-7 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.