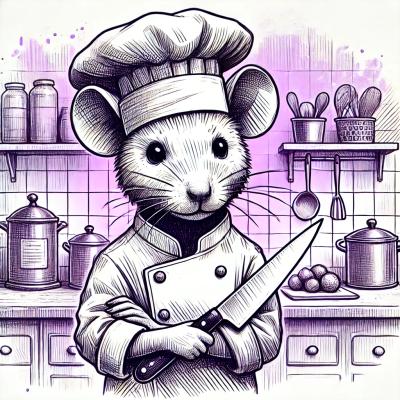
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The es6-map npm package provides an implementation of the Map data structure as specified in ECMAScript 6 (ES6). This package is particularly useful for environments that do not fully support ES6 features, allowing developers to use Map functionalities such as maintaining key-value pairs where keys can be of any type and preserving the insertion order of keys.
Creating and initializing a Map
This feature allows the creation of a Map and initialization with key-value pairs. The 'get' method retrieves the value associated with a key.
const ES6Map = require('es6-map');
const map = new ES6Map([['key1', 'value1'], ['key2', 'value2']]);
console.log(map.get('key1')); // Outputs: 'value1'
Checking existence of a key
This feature checks whether a specific key exists in the Map using the 'has' method.
const map = new ES6Map([['key1', 'value1']]);
console.log(map.has('key1')); // Outputs: true
console.log(map.has('key2')); // Outputs: false
Iterating over Map entries
This feature demonstrates how to iterate over the entries of the Map, accessing both keys and values.
const map = new ES6Map([['key1', 'value1'], ['key2', 'value2']]);
for (let [key, value] of map) {
console.log(key + ': ' + value);
}
The 'immutable' package provides persistent immutable data structures including Map, List, Set, etc. Unlike es6-map, which only offers a Map polyfill, 'immutable' offers a wide range of data structures and features like lazy operation chaining, deep persistence, and complex data nesting.
The 'collections' package offers data structures with performance in mind, including Map, Set, List, and others. It provides additional utilities compared to es6-map, such as sorted maps and sets, and specialized collections like Deque.
Warning:
v0.1 version does not ensure O(1) algorithm complexity (but O(n)). This shortcoming will be addressed in v1.0
It’s safest to use es6-map as a ponyfill – a polyfill which doesn’t touch global objects:
var Map = require('es6-map');
If you want to make sure your environment implements Map
globally, do:
require('es6-map/implement');
If you strictly want to use the polyfill even if the native Map
exists, do:
var Map = require('es6-map/polyfill');
$ npm install es6-map
To port it to Browser or any other (non CJS) environment, use your favorite CJS bundler. No favorite yet? Try: Browserify, Webmake or Webpack
Best is to refer to specification. Still if you want quick look, follow examples:
var Map = require('es6-map');
var x = {}, y = {}, map = new Map([['raz', 'one'], ['dwa', 'two'], [x, y]]);
map.size; // 3
map.get('raz'); // 'one'
map.get(x); // y
map.has('raz'); // true
map.has(x); // true
map.has('foo'); // false
map.set('trzy', 'three'); // map
map.size // 4
map.get('trzy'); // 'three'
map.has('trzy'); // true
map.has('dwa'); // true
map.delete('dwa'); // true
map.size; // 3
map.forEach(function (value, key) {
// { 'raz', 'one' }, { x, y }, { 'trzy', 'three' } iterated
});
// FF nightly only:
for (value of map) {
// ['raz', 'one'], [x, y], ['trzy', 'three'] iterated
}
var iterator = map.values();
iterator.next(); // { done: false, value: 'one' }
iterator.next(); // { done: false, value: y }
iterator.next(); // { done: false, value: 'three' }
iterator.next(); // { done: true, value: undefined }
map.clear(); // undefined
map.size; // 0
$ npm test
FAQs
ECMAScript6 Map polyfill
The npm package es6-map receives a total of 763,700 weekly downloads. As such, es6-map popularity was classified as popular.
We found that es6-map demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.