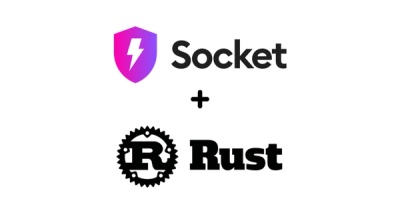
Product
Introducing Rust Support in Socket
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
es6-promise
Advanced tools
A lightweight library that provides tools for organizing asynchronous code
The es6-promise package is a polyfill for the ECMAScript 6 Promise. It provides a way to handle asynchronous operations in JavaScript by allowing you to associate handlers with an asynchronous action's eventual success value or failure reason. This lets asynchronous methods return values like synchronous methods: instead of immediately returning the final value, the asynchronous method returns a promise to supply the value at some point in the future.
Creating a Promise
This feature allows you to create a new Promise object. The constructor of Promise takes a function that takes two arguments, resolve and reject, which are functions themselves. You perform the asynchronous operation within this function and call resolve upon successful completion with the result, or reject with an error.
var promise = new Promise(function(resolve, reject) {
// do something asynchronous which eventually calls either:
// resolve(someValue); // fulfilled
// or
// reject("failure reason"); // rejected
});
Using a Promise
This feature demonstrates how to use a Promise. Once a Promise has been created, you can attach success and failure handlers to it using the .then method. The first function passed to .then is called if the Promise is resolved, and the second is called if it is rejected.
promise.then(function(value) {
// success
}, function(value) {
// failure
});
Chaining Promises
Promises can be chained, meaning that the result of one promise can be used to create another promise, forming a chain of promises. This is useful for performing a series of asynchronous operations in sequence.
new Promise(function(resolve, reject) {
setTimeout(() => resolve(1), 1000);
}).then(function(result) {
console.log(result); // 1
return result * 2;
}).then(function(result) {
console.log(result); // 2
return result * 2;
}).then(function(result) {
console.log(result); // 4
return result * 2;
});
Bluebird is a fully featured promise library with focus on innovative features and performance. It is known for its rich API and being one of the fastest promise libraries. Compared to es6-promise, Bluebird offers more utilities such as .map, .reduce, and .filter for promises, making it a more comprehensive solution for handling asynchronous operations.
Q is an earlier promise library that influenced many aspects of the Promise specification. It provides a robust set of features for creating and composing promises. While es6-promise aims to provide a polyfill for native ES6 Promises with minimalistic API, Q offers additional features like promise cancellation, better error handling, and progress notifications.
This is a polyfill of the ES6 Promise. The implementation is a subset of rsvp.js extracted by @jakearchibald, if you're wanting extra features and more debugging options, check out the full library.
For API details and how to use promises, see the JavaScript Promises HTML5Rocks article.
Promise
if missing or broken.es6-promise-auto
above.To use via a CDN include this in your html:
<!-- Automatically provides/replaces `Promise` if missing or broken. -->
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.js"></script>
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.js"></script>
<!-- Minified version of `es6-promise-auto` below. -->
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.min.js"></script>
To install:
yarn add es6-promise
or
npm install es6-promise
To use:
var Promise = require('es6-promise').Promise;
catch
and finally
are reserved keywords in IE<9, meaning
promise.catch(func)
or promise.finally(func)
throw a syntax error. To work
around this, you can use a string to access the property as shown in the
following example.
However most minifiers will automatically fix this for you, making the resulting code safe for old browsers and production:
promise['catch'](function(err) {
// ...
});
promise['finally'](function() {
// ...
});
To polyfill the global environment (either in Node or in the browser via CommonJS) use the following code snippet:
require('es6-promise').polyfill();
Alternatively
require('es6-promise/auto');
Notice that we don't assign the result of polyfill()
to any variable. The polyfill()
method will patch the global environment (in this case to the Promise
name) when called.
You will need to have PhantomJS installed globally in order to run the tests.
npm install -g phantomjs
npm run build
to buildnpm test
to run testsnpm start
to run a build watcher, and webserver to testnpm run test:server
for a testem test runner and watching builderFAQs
A lightweight library that provides tools for organizing asynchronous code
The npm package es6-promise receives a total of 9,933,049 weekly downloads. As such, es6-promise popularity was classified as popular.
We found that es6-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.