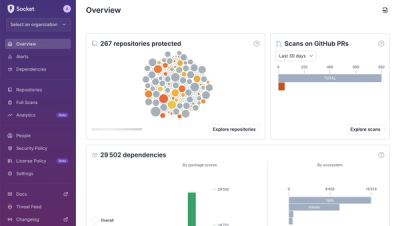
Product
A New Overview in our Dashboard
We redesigned Socket's first logged-in page to display rich and insightful visualizations about your repositories protected against supply chain threats.
es6-promisify
Advanced tools
The es6-promisify package is a utility that converts callback-based functions to return Promises, making it easier to work with asynchronous code in a more modern and readable way.
Promisify a single callback-based function
This feature allows you to convert a single callback-based function, such as `fs.readFile`, into a function that returns a Promise. This makes it easier to handle asynchronous operations using modern async/await syntax or Promise chaining.
const promisify = require('es6-promisify');
const fs = require('fs');
const readFileAsync = promisify(fs.readFile);
readFileAsync('example.txt', 'utf8')
.then(data => console.log(data))
.catch(err => console.error(err));
Promisify an entire object of callback-based functions
This feature allows you to promisify all functions within an object, such as the `fs` module, so that all of its methods return Promises. This is useful for working with modules that have multiple callback-based methods.
const promisify = require('es6-promisify');
const fs = require('fs');
const fsAsync = promisify(fs);
fsAsync.readFile('example.txt', 'utf8')
.then(data => console.log(data))
.catch(err => console.error(err));
The `util.promisify` function is a built-in Node.js utility that provides similar functionality to es6-promisify. It converts callback-based functions to return Promises. Since it is built into Node.js, it does not require an additional package installation. However, it is only available in Node.js versions 8.0.0 and above.
Bluebird is a fully-featured Promise library that includes a `promisify` method. It offers more advanced features and better performance compared to es6-promisify. Bluebird is a good choice if you need additional Promise utilities and optimizations.
Pify is a lightweight utility that promisifies callback-style functions. It is similar to es6-promisify but offers more customization options, such as filtering which methods to promisify and handling multiple arguments. Pify is a good alternative if you need more control over the promisification process.
Converts callback-based functions to Promises, using a boilerplate callback function.
Install with npm
npm install es6-promisify
const {promisify} = require("es6-promisify");
// Convert the stat function
const fs = require("fs");
const stat = promisify(fs.stat);
// Now usable as a promise!
try {
const stats = await stat("example.txt");
console.log("Got stats", stats);
} catch (err) {
console.error("Yikes!", err);
}
const {promisify} = require("es6-promisify");
// Create a promise-based version of send_command
const redis = require("redis").createClient(6379, "localhost");
const client = promisify(redis.send_command.bind(redis));
// Send commands to redis and get a promise back
try {
const pong = await client.ping();
console.log("Got", pong);
} catch (err) {
console.error("Unexpected error", err);
} finally {
redis.quit();
}
const {promisify} = require("es6-promisify");
function test(cb) {
return cb(undefined, 1, 2, 3);
}
// Create promise-based version of test
test[promisify.argumentNames] = ["one", "two", "three"];
const multi = promisify(test);
// Returns named arguments
const result = await multi();
console.log(result); // {one: 1, two: 2, three: 3}
const {promisify} = require("es6-promisify");
// Now uses Bluebird
promisify.Promise = require("bluebird");
const test = promisify(cb => cb(undefined, "test"));
const result = await test();
console.log(result); // "test", resolved using Bluebird
Test with tape
$ npm test
Published under the MIT License.
FAQs
Converts callback-based functions to ES6 Promises
The npm package es6-promisify receives a total of 3,861,832 weekly downloads. As such, es6-promisify popularity was classified as popular.
We found that es6-promisify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We redesigned Socket's first logged-in page to display rich and insightful visualizations about your repositories protected against supply chain threats.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.