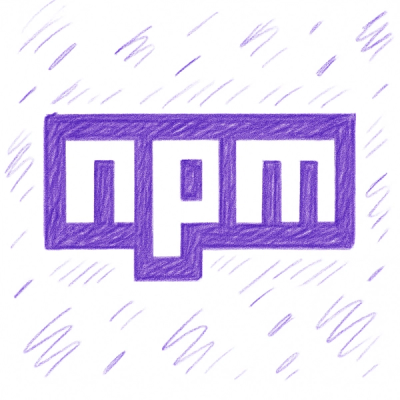
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
es6-weak-map
Advanced tools
The es6-weak-map npm package provides a polyfill for the ES6 WeakMap implementation. It allows for the creation of collections of key/value pairs where the keys are objects and the values can be arbitrary values. The key feature of WeakMaps is that they do not prevent garbage collection if there are no other references to the key object, making them useful for managing memory in large applications.
Creating and using a WeakMap
This code demonstrates how to create a WeakMap, add a key/value pair to it, retrieve the value using the key, and then allow the key to be garbage collected by removing references to it.
{"var WeakMap = require('es6-weak-map');\nvar myWeakMap = new WeakMap();\nvar obj = {};\nmyWeakMap.set(obj, 'value');\nconsole.log(myWeakMap.get(obj)); // 'value'\nobj = null; // Now obj can be garbage collected if no other references exist"}
Checking for a key's presence and deleting a key
This example shows how to check if a WeakMap contains a certain key using the `has` method and how to remove a key/value pair from the WeakMap using the `delete` method.
{"var WeakMap = require('es6-weak-map');\nvar myWeakMap = new WeakMap();\nvar obj = {};\nmyWeakMap.set(obj, 'value');\nconsole.log(myWeakMap.has(obj)); // true\nmyWeakMap.delete(obj);\nconsole.log(myWeakMap.has(obj)); // false"}
This package provides a polyfill for WeakMap for environments where it's not supported natively. It's similar to es6-weak-map but might have different performance characteristics or compatibility nuances.
Another implementation of the WeakMap specification, offering similar functionality to es6-weak-map. It might differ in aspects such as API design, performance optimizations, or additional features.
Roughly inspired by Mark Miller's and Kris Kowal's WeakMap implementation.
Differences are:
$ npm install es6-weak-map
To port it to Browser or any other (non CJS) environment, use your favorite CJS bundler. No favorite yet? Try: Browserify, Webmake or Webpack
If you want to make sure your environment implements WeakMap
, do:
require("es6-weak-map/implement");
If you'd like to use native version when it exists and fallback to polyfill if it doesn't, but without implementing WeakMap
on global scope, do:
var WeakMap = require("es6-weak-map");
If you strictly want to use polyfill even if native WeakMap
exists, do:
var WeakMap = require("es6-weak-map/polyfill");
Best is to refer to specification. Still if you want quick look, follow example:
var WeakMap = require("es6-weak-map");
var map = new WeakMap();
var obj = {};
map.set(obj, "foo"); // map
map.get(obj); // 'foo'
map.has(obj); // true
map.delete(obj); // true
map.get(obj); // undefined
map.has(obj); // false
map.set(obj, "bar"); // map
map.has(obj); // false
$ npm test
FAQs
ECMAScript6 WeakMap polyfill
The npm package es6-weak-map receives a total of 5,827,663 weekly downloads. As such, es6-weak-map popularity was classified as popular.
We found that es6-weak-map demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.