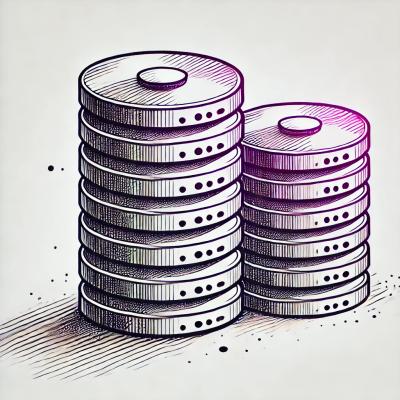
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
esbuild-register
Advanced tools
The esbuild-register package allows you to transpile your code on the fly using esbuild. This is particularly useful for running TypeScript or modern JavaScript that uses JSX or other newer syntax without a separate build step. It hooks into Node.js's require in order to automatically compile files on-the-fly when they are required.
Transpile TypeScript on the fly
This feature allows you to automatically transpile TypeScript files when they are required in Node.js. This is particularly useful for development environments, where you want to avoid a separate build step for TypeScript.
require('esbuild-register/dist/node').register({
loader: 'tsx'
});
require('./your-ts-file.ts');
Transpile modern JavaScript (ES6+) on the fly
With this feature, you can automatically transpile modern JavaScript files that use ES6+ features or JSX. This is useful for running modern JavaScript directly in Node.js without pre-compilation.
require('esbuild-register').register({
loader: 'jsx'
});
require('./your-modern-js-file.js');
ts-node is a TypeScript execution engine and REPL for Node.js. It directly executes TypeScript files and compiles them on the fly. Compared to esbuild-register, ts-node might be slower for some projects because it uses the TypeScript compiler, which is generally slower than esbuild.
babel-register hooks into Node.js's require to automatically transpile files on the fly using Babel. It supports a wide range of JavaScript features and experimental syntax through plugins. While more flexible and powerful, it is typically slower than esbuild-register due to the nature of Babel's compilation process.
Sucrase is a super-fast alternative to Babel for builds and development workflows. It focuses on compiling non-standard JavaScript syntax like JSX, TypeScript, and Flow into standard JavaScript. While similar in performance to esbuild-register for some use cases, it lacks some of esbuild's optimizations and features.
npm i esbuild esbuild-register -D
# Or Yarn
yarn add esbuild esbuild-register --dev
# Or pnpm
pnpm add esbuild esbuild-register -D
node -r esbuild-register file.ts
It will use jsxFactory
, jsxFragmentFactory
and target
options from your tsconfig.json
When using in a project with type: "module"
in package.json
, you need the --loader
flag to load TypeScript files:
node --loader esbuild-register/loader -r esbuild-register ./file.ts
const { register } = require('esbuild-register/dist/node')
const { unregister } = register({
// ...options
})
// Unregister the require hook if you don't need it anymore
unregister()
MIT © EGOIST
FAQs
Transpile JSX, TypeScript and esnext features on the fly with esbuild
We found that esbuild-register demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.