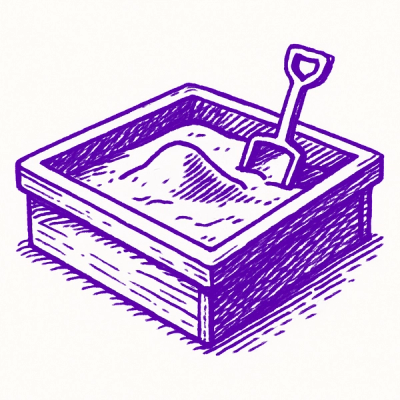
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
eslint-config-standard-react
Advanced tools
JavaScript Standard Style React/JSX support - ESLint Shareable Config
This module is for advanced users. You probably want to use standard
instead :)
npm install eslint-config-standard-react
Shareable configs are designed to work with the extends
feature of .eslintrc
files.
You can learn more about
Shareable Configs on the
official ESLint website.
This Shareable Config adds React and JSX to the baseline JavaScript Standard Style rules
provided in eslint-config-standard
.
Here's how to install everything you need:
npm install --save-dev @babel/core @babel/eslint-parser eslint-config-standard eslint-config-standard-jsx eslint-config-standard-react eslint-plugin-promise eslint-plugin-import eslint-plugin-node eslint-plugin-react
Then, add this to your .eslintrc
file:
{
"parser": "@babel/eslint-parser",
"extends": ["standard", "standard-jsx", "standard-react"]
}
Note: We omitted the eslint-config-
prefix since it is automatically assumed by ESLint.
You can override settings from the shareable config by adding them directly into your
.eslintrc
file.
The easiest way to use JavaScript Standard Style to check your code is to use the
standard
package. This comes with a global
Node command line program (standard
) that you can run or add to your npm test
script
to quickly check your style.
Use this in one of your projects? Include one of these badges in your readme to let people know that your code is using the standard style.
[](https://github.com/standard/standard)
[](https://github.com/standard/standard)
For the full listing of rules, editor plugins, FAQs, and more, visit the main JavaScript Standard Style repo.
MIT. Copyright (c) Feross Aboukhadijeh.
FAQs
JavaScript Standard Style React/JSX support - ESLint Shareable Config
The npm package eslint-config-standard-react receives a total of 136,997 weekly downloads. As such, eslint-config-standard-react popularity was classified as popular.
We found that eslint-config-standard-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.