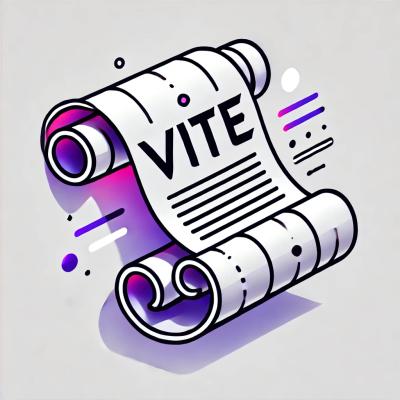
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
eslint-import-resolver-alias
Advanced tools
a simple Node behavior import resolution plugin for eslint-plugin-import, supporting module alias.
The eslint-import-resolver-alias package is an ESLint plugin that allows you to resolve import paths using custom alias mappings. This is particularly useful in projects where you have complex directory structures and want to simplify import statements.
Custom Alias Resolution
This feature allows you to define custom alias mappings for your project. In the example, `@components` is mapped to `./src/components` and `@utils` is mapped to `./src/utils`. This makes it easier to import modules without having to use relative paths.
{"settings":{"import/resolver":{"alias":{"map":[["@components","./src/components"],["@utils","./src/utils"]],"extensions":[".js",".jsx",".ts",".tsx"]}}}}
Support for Multiple Extensions
You can specify multiple file extensions that the resolver should consider. In this example, the resolver will look for files with `.js`, `.jsx`, `.ts`, and `.tsx` extensions when resolving the `@components` alias.
{"settings":{"import/resolver":{"alias":{"map":[["@components","./src/components"]],"extensions":[".js",".jsx",".ts",".tsx"]}}}}
The eslint-import-resolver-webpack package allows you to use your Webpack configuration to resolve import paths. This is useful if you are already using Webpack and want to maintain consistency between your Webpack and ESLint configurations. Unlike eslint-import-resolver-alias, which focuses on custom alias mappings, eslint-import-resolver-webpack leverages the entire Webpack configuration.
The eslint-import-resolver-node package resolves import paths using Node.js' resolution algorithm. This is useful for projects that follow Node.js conventions and do not require custom alias mappings. It is simpler compared to eslint-import-resolver-alias and is ideal for straightforward Node.js projects.
This is a simple Node.js module import resolution plugin for eslint-plugin-import
, which supports native Node.js module resolution, module alias/mapping and custom file extensions.
Prerequisites: Node.js >=4.x and corresponding version of npm.
npm install eslint-plugin-import eslint-import-resolver-alias --save-dev
Pass this resolver and its parameters to eslint-plugin-import
using your eslint
config file, .eslintrc
or .eslintrc.js
.
// .eslintrc.js
module.exports = {
settings: {
'import/resolver': {
alias: {
map: [
['babel-polyfill', 'babel-polyfill/dist/polyfill.min.js'],
['helper', './utils/helper'],
['material-ui/DatePicker', '../custom/DatePicker'],
['material-ui', 'material-ui-ie10']
],
extensions: ['.ts', '.js', '.jsx', '.json']
}
}
}
};
Note:
map
and extensions
, both of which are array typesmap
array is also array type which contains 2 string
map
item ['helper', './utils/helper']
means that the modules which match helper
or helper/*
will be resolved to ./utils/helper
or ./utils/helper/*
which are located relative to the process current working directory
(almost the project root directory). If you just want to resolve helper
to ./utils/helper
, use ['^helper$', './utils/helper']
instead. See issue #3extensions
property is ['.js', '.json', '.node']
if it is assigned to an empty array or not specifiedIf the extensions
property is not specified, the config object can be simplified to the map
array.
// .eslintrc.js
module.exports = {
settings: {
'import/resolver': {
alias: [
['babel-polyfill', 'babel-polyfill/dist/polyfill.min.js'],
['helper', './utils/helper'],
['material-ui/DatePicker', '../custom/DatePicker'],
['material-ui', 'material-ui-ie10']
]
}
}
};
When the config is not a valid object (such as true
), the resolver falls back to native Node.js module resolution.
// .eslintrc.js
module.exports = {
settings: {
'import/resolver': {
alias: true
}
}
};
[1.1.2] - 2018-12-08
path/to/file
to path/from/file
with the config ['to', 'from']
. see [#7][issue7]FAQs
a simple Node behavior import resolution plugin for eslint-plugin-import, supporting module alias.
The npm package eslint-import-resolver-alias receives a total of 805,222 weekly downloads. As such, eslint-import-resolver-alias popularity was classified as popular.
We found that eslint-import-resolver-alias demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.