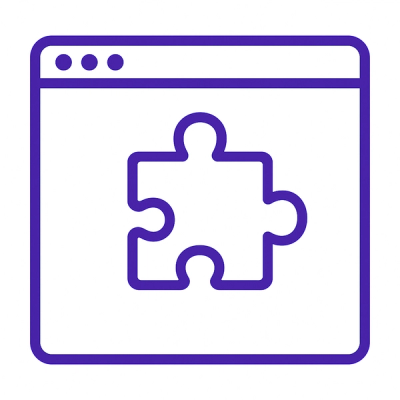
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
eslint-plugin-fp
Advanced tools
ESLint rules for functional programming
$ npm install --save-dev eslint eslint-plugin-fp
Configure it in package.json
.
{
"name": "my-awesome-project",
"eslintConfig": {
"env": {
"es6": true
},
"plugins": [
"fp"
],
"rules": {
"fp/no-arguments": "error",
"fp/no-class": "error",
"fp/no-delete": "error",
"fp/no-events": "error",
"fp/no-get-set": "error",
"fp/no-let": "error",
"fp/no-loops": "error",
"fp/no-mutating-assign": "error",
"fp/no-mutating-methods": "error",
"fp/no-mutation": "error",
"fp/no-nil": "error",
"fp/no-proxy": "error",
"fp/no-rest-parameters": "error",
"fp/no-this": "error",
"fp/no-throw": "error",
"fp/no-unused-expression": "error",
"fp/no-valueof-field": "error",
"no-var": "error"
}
}
}
arguments
.class
.delete
.events
module.let
.Object.assign()
with a variable as first argument.null
and undefined
.Proxy
.this
.throw
.valueOf
fields.This plugin exports a recommended
configuration that enforces good practices.
To enable this configuration, use the extends
property in your package.json
.
{
"name": "my-awesome-project",
"eslintConfig": {
"plugins": [
"fp"
],
"extends": "plugin:fp/recommended"
}
}
See ESLint documentation for more information about extending configuration files.
MIT © Jeroen Engels
[2.3.0] - 2017-01-01
allowUseStrict
option to [no-unused-expression
] to allow 'use strict';
statements.eslint-ast-utils
] to replace some logic.arguments
in [no-arguments
].no-mutating-assign
].FAQs
ESLint rules for functional programming
The npm package eslint-plugin-fp receives a total of 74,950 weekly downloads. As such, eslint-plugin-fp popularity was classified as popular.
We found that eslint-plugin-fp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.