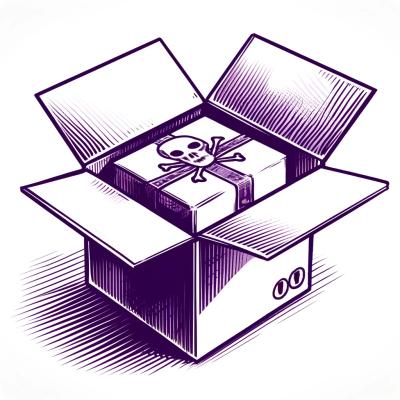
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
eslint-plugin-no-use-extend-native
Advanced tools
ESLint plugin to prevent use of extended native objects
ESLint plugin to prevent use of extended native objects
First, install ESLint via
npm install --save-dev eslint
Then install eslint-plugin-no-use-extend-native
npm install --save-dev eslint-plugin-no-use-extend-native
In your eslint.config.js
file add the plugin as such:
import eslintPluginNoUseExtendNative from 'eslint-plugin-no-use-extend-native'
export default [
{
plugins: {
'no-use-extend-native': eslintPluginNoUseExtendNative,
},
rules: {
'no-use-extend-native/no-use-extend-native': 2,
},
},
]
If you want the default of the single rule being enabled as an error, you can also just use the following instead of all of the above:
import eslintPluginNoUseExtendNative from 'eslint-plugin-no-use-extend-native'
export default [
eslintPluginNoUseExtendNative.configs.recommended,
]
With this plugin enabled, ESLint will find issues with using extended native objects:
import colors from 'colors';
console.log('unicorn'.green);
// => ESLint will give an error stating 'Avoid using extended native objects'
[].customFunction();
// => ESLint will give an error stating 'Avoid using extended native objects'
More examples can be seen in the tests.
ESLint's no-extend-native
rule verifies code is not modifying a native prototype. e.g., with the no-extend-native
rule enabled, the following lines are each considered incorrect:
String.prototype.shortHash = function() { return this.substring(0, 7); };
Object.defineProperty(Array.prototype, "times", { value: 999 });
no-use-extend-native
verifies code is not using a non-native prototype. e.g., with the no-use-extend-native
plugin enabled, the following line is considered incorrect:
"50bda47b09923e045759db8e8dd01a0bacd97370".shortHash() === "50bda47";
The no-use-extend-native
plugin is designed to work with ESLint's no-extend-native
rule. no-extend-native
ensures that native prototypes aren't extended, and should a third party library extend them, no-use-extend-native
ensures those changes aren't depended upon.
MIT © Dustin Specker
FAQs
ESLint plugin to prevent use of extended native objects
The npm package eslint-plugin-no-use-extend-native receives a total of 91,687 weekly downloads. As such, eslint-plugin-no-use-extend-native popularity was classified as popular.
We found that eslint-plugin-no-use-extend-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.