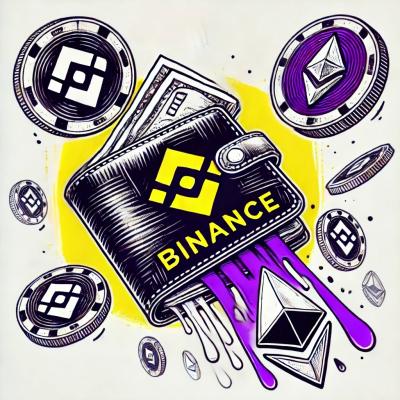
Research
Security News
Malicious npm Packages Target BSC and Ethereum to Drain Crypto Wallets
Socket uncovered four malicious npm packages that exfiltrate up to 85% of a victim’s Ethereum or BSC wallet using obfuscated JavaScript.
eslint-plugin-svelte
Advanced tools
eslint-plugin-svelte
is the official ESLint plugin for Svelte.
It leverages the AST generated by svelte-eslint-parser to provide custom linting for Svelte.
Note that eslint-plugin-svelte
and svelte-eslint-parser
cannot be used alongside eslint-plugin-svelte3.
npm install --save-dev svelte eslint eslint-plugin-svelte globals
[!NOTE]
Requirements:
- ESLint v8.57.1, v9.0.0, and above
- Node.js v18.18.0, v20.9.0, v21.1.0 and above
Use the eslint.config.js
file to configure rules. For more details, see the ESLint documentation.
// eslint.config.js
import js from '@eslint/js';
import svelte from 'eslint-plugin-svelte';
import globals from 'globals';
import svelteConfig from './svelte.config.js';
/** @type {import('eslint').Linter.Config[]} */
export default [
js.configs.recommended,
...svelte.configs.recommended,
{
languageOptions: {
globals: {
...globals.browser,
...globals.node // Add this if you are using SvelteKit in non-SPA mode
}
}
},
{
files: ['**/*.svelte', '**/*.svelte.js'],
languageOptions: {
parserOptions: {
// We recommend importing and specifying svelte.config.js.
// By doing so, some rules in eslint-plugin-svelte will automatically read the configuration and adjust their behavior accordingly.
// While certain Svelte settings may be statically loaded from svelte.config.js even if you don’t specify it,
// explicitly specifying it ensures better compatibility and functionality.
svelteConfig
}
}
},
{
rules: {
// Override or add rule settings here, such as:
// 'svelte/rule-name': 'error'
}
}
];
npm install --save-dev typescript-eslint
// eslint.config.js
import js from '@eslint/js';
import svelte from 'eslint-plugin-svelte';
import globals from 'globals';
import ts from 'typescript-eslint';
import svelteConfig from './svelte.config.js';
export default ts.config(
js.configs.recommended,
...ts.configs.recommended,
...svelte.configs.recommended,
{
languageOptions: {
globals: {
...globals.browser,
...globals.node
}
}
},
{
files: ['**/*.svelte', '**/*.svelte.ts', '**/*.svelte.js'],
// See more details at: https://typescript-eslint.io/packages/parser/
languageOptions: {
parserOptions: {
projectService: true,
extraFileExtensions: ['.svelte'], // Add support for additional file extensions, such as .svelte
parser: ts.parser,
// Specify a parser for each language, if needed:
// parser: {
// ts: ts.parser,
// js: espree, // Use espree for .js files (add: import espree from 'espree')
// typescript: ts.parser
// },
// We recommend importing and specifying svelte.config.js.
// By doing so, some rules in eslint-plugin-svelte will automatically read the configuration and adjust their behavior accordingly.
// While certain Svelte settings may be statically loaded from svelte.config.js even if you don’t specify it,
// explicitly specifying it ensures better compatibility and functionality.
svelteConfig
}
}
},
{
rules: {
// Override or add rule settings here, such as:
// 'svelte/rule-name': 'error'
}
}
);
[!WARNING] The TypeScript parser uses a singleton internally, meaning it only respects the options provided during its initial initialization. If you try to change the options for a different file or override them later, the parser will ignore the new options, which may lead to errors. For more context, see typescript-eslint/typescript-eslint#6778.
This plugin provides the following configurations:
eslintPluginSvelte.configs.base
... Enables correct Svelte parsing.eslintPluginSvelte.configs.recommended
... Includes base
configuration, plus rules to prevent errors or unintended behavior.eslintPluginSvelte.configs.prettier
... Disables rules that may conflict with Prettier. You still need to configure Prettier to work with Svelte manually, for example, by using prettier-plugin-svelte.eslintPluginSvelte.configs.all
... Includes all available rules. Note: This configuration is not recommended for production use, as it changes with every minor and major version of eslint-plugin-svelte
. Use at your own risk.For more details, see the rule list to explore the rules provided by this plugin.
You can customize the behavior of this plugin using specific settings.
// eslint.config.js
export default [
// ...
{
settings: {
svelte: {
// Specifies an array of rules to ignore reports within the template.
// For example, use this to disable rules in the template that may produce unavoidable false positives.
ignoreWarnings: [
'@typescript-eslint/no-unsafe-assignment',
'@typescript-eslint/no-unsafe-member-access'
],
// Specifies options for Svelte compilation.
// This affects rules that rely on Svelte compilation,
// such as svelte/valid-compile and svelte/no-unused-svelte-ignore.
// Note that this setting does not impact ESLint’s custom parser.
compileOptions: {
// Specifies options related to PostCSS. You can disable the PostCSS processing by setting it to false.
postcss: {
// Specifies the path to the directory that contains the PostCSS configuration.
configFilePath: './path/to/my/postcss.config.js'
}
},
// Even if settings.svelte.kit is not specified, the rules will attempt to load information from svelte.config.js.
// However, if the default behavior does not work as expected, you should specify settings.svelte.kit explicitly.
// If you are using SvelteKit with a non-default configuration, you need to set the following options.
// The schema is a subset of SvelteKit’s configuration, so refer to the SvelteKit documentation for more details.
// https://svelte.dev/docs/kit/configuration
kit: {
files: {
routes: 'src/routes'
}
}
}
}
}
// ...
];
Visual Studio Code
Install dbaeumer.vscode-eslint.
Configure .svelte
files in .vscode/settings.json
:
{
"eslint.validate": ["javascript", "javascriptreact", "svelte"]
}
If you’re migrating from eslint-plugin-svelte
v1 or @ota-meshi/eslint-plugin-svelte
, see the migration guide.
This project follows Semantic Versioning. Unlike ESLint’s versioning policy, new rules may be added to the recommended config in minor releases. If these rules cause unwanted warnings, you can disable them.
:wrench: Indicates that the rule is fixable, and using --fix
option on the command line can automatically fix some of the reported problems.
:bulb: Indicates that some problems reported by the rule are manually fixable by editor suggestions.
:star: Indicates that the rule is included in the plugin:svelte/recommended
config.
These rules relate to possible syntax or logic errors in Svelte code:
Rule ID | Description | |
---|---|---|
svelte/infinite-reactive-loop | Svelte runtime prevents calling the same reactive statement twice in a microtask. But between different microtask, it doesn't prevent. | :star: |
svelte/no-dom-manipulating | disallow DOM manipulating | :star: |
svelte/no-dupe-else-if-blocks | disallow duplicate conditions in {#if} / {:else if} chains | :star: |
svelte/no-dupe-on-directives | disallow duplicate on: directives | :star: |
svelte/no-dupe-style-properties | disallow duplicate style properties | :star: |
svelte/no-dupe-use-directives | disallow duplicate use: directives | :star: |
svelte/no-not-function-handler | disallow use of not function in event handler | :star: |
svelte/no-object-in-text-mustaches | disallow objects in text mustache interpolation | :star: |
svelte/no-raw-special-elements | Checks for invalid raw HTML elements | :star::wrench: |
svelte/no-reactive-reassign | disallow reassigning reactive values | :star: |
svelte/no-shorthand-style-property-overrides | disallow shorthand style properties that override related longhand properties | :star: |
svelte/no-store-async | disallow using async/await inside svelte stores because it causes issues with the auto-unsubscribing features | :star: |
svelte/no-top-level-browser-globals | disallow using top-level browser global variables | |
svelte/no-unknown-style-directive-property | disallow unknown style:property | :star: |
svelte/require-store-callbacks-use-set-param | store callbacks must use set param | :bulb: |
svelte/require-store-reactive-access | disallow to use of the store itself as an operand. Need to use $ prefix or get function. | :star::wrench: |
svelte/valid-compile | disallow warnings when compiling. | |
svelte/valid-style-parse | require valid style element parsing |
These rules relate to security vulnerabilities in Svelte code:
Rule ID | Description | |
---|---|---|
svelte/no-at-html-tags | disallow use of {@html} to prevent XSS attack | :star: |
svelte/no-target-blank | disallow target="_blank" attribute without rel="noopener noreferrer" |
These rules relate to better ways of doing things to help you avoid problems:
Rule ID | Description | |
---|---|---|
svelte/block-lang | disallows the use of languages other than those specified in the configuration for the lang attribute of <script> and <style> blocks. | :bulb: |
svelte/button-has-type | disallow usage of button without an explicit type attribute | |
svelte/no-add-event-listener | Warns against the use of addEventListener | :bulb: |
svelte/no-at-debug-tags | disallow the use of {@debug} | :star::bulb: |
svelte/no-ignored-unsubscribe | disallow ignoring the unsubscribe method returned by the subscribe() on Svelte stores. | |
svelte/no-immutable-reactive-statements | disallow reactive statements that don't reference reactive values. | :star: |
svelte/no-inline-styles | disallow attributes and directives that produce inline styles | |
svelte/no-inspect | Warns against the use of $inspect directive | :star: |
svelte/no-reactive-functions | it's not necessary to define functions in reactive statements | :star::bulb: |
svelte/no-reactive-literals | don't assign literal values in reactive statements | :star::bulb: |
svelte/no-svelte-internal | svelte/internal will be removed in Svelte 6. | :star: |
svelte/no-unnecessary-state-wrap | Disallow unnecessary $state wrapping of reactive classes | :star::bulb: |
svelte/no-unused-class-name | disallow the use of a class in the template without a corresponding style | |
svelte/no-unused-props | Warns about defined Props properties that are unused | :star: |
svelte/no-unused-svelte-ignore | disallow unused svelte-ignore comments | :star: |
svelte/no-useless-children-snippet | disallow explicit children snippet where it's not needed | :star: |
svelte/no-useless-mustaches | disallow unnecessary mustache interpolations | :star::wrench: |
svelte/prefer-const | Require const declarations for variables that are never reassigned after declared | :wrench: |
svelte/prefer-destructured-store-props | destructure values from object stores for better change tracking & fewer redraws | :bulb: |
svelte/prefer-writable-derived | Prefer using writable $derived instead of $state and $effect | :star::bulb: |
svelte/require-each-key | require keyed {#each} block | :star: |
svelte/require-event-dispatcher-types | require type parameters for createEventDispatcher | :star: |
svelte/require-optimized-style-attribute | require style attributes that can be optimized | |
svelte/require-stores-init | require initial value in store | |
svelte/valid-each-key | enforce keys to use variables defined in the {#each} block | :star: |
These rules relate to style guidelines, and are therefore quite subjective:
Rule ID | Description | |
---|---|---|
svelte/consistent-selector-style | enforce a consistent style for CSS selectors | |
svelte/derived-has-same-inputs-outputs | derived store should use same variable names between values and callback | :bulb: |
svelte/first-attribute-linebreak | enforce the location of first attribute | :wrench: |
svelte/html-closing-bracket-new-line | Require or disallow a line break before tag's closing brackets | :wrench: |
svelte/html-closing-bracket-spacing | require or disallow a space before tag's closing brackets | :wrench: |
svelte/html-quotes | enforce quotes style of HTML attributes | :wrench: |
svelte/html-self-closing | enforce self-closing style | :wrench: |
svelte/indent | enforce consistent indentation | :wrench: |
svelte/max-attributes-per-line | enforce the maximum number of attributes per line | :wrench: |
svelte/mustache-spacing | enforce unified spacing in mustache | :wrench: |
svelte/no-extra-reactive-curlies | disallow wrapping single reactive statements in curly braces | :bulb: |
svelte/no-restricted-html-elements | disallow specific HTML elements | |
svelte/no-spaces-around-equal-signs-in-attribute | disallow spaces around equal signs in attribute | :wrench: |
svelte/prefer-class-directive | require class directives instead of ternary expressions | :wrench: |
svelte/prefer-style-directive | require style directives instead of style attribute | :wrench: |
svelte/require-event-prefix | require component event names to start with "on" | |
svelte/shorthand-attribute | enforce use of shorthand syntax in attribute | :wrench: |
svelte/shorthand-directive | enforce use of shorthand syntax in directives | :wrench: |
svelte/sort-attributes | enforce order of attributes | :wrench: |
svelte/spaced-html-comment | enforce consistent spacing after the <!-- and before the --> in a HTML comment | :wrench: |
These rules extend the rules provided by ESLint itself, or other plugins to work well in Svelte:
Rule ID | Description | |
---|---|---|
svelte/no-inner-declarations | disallow variable or function declarations in nested blocks | :star: |
svelte/no-trailing-spaces | disallow trailing whitespace at the end of lines | :wrench: |
These rules relate to SvelteKit and its best Practices.
Rule ID | Description | |
---|---|---|
svelte/no-export-load-in-svelte-module-in-kit-pages | disallow exporting load functions in *.svelte module in SvelteKit page components. | :star: |
svelte/no-navigation-without-base | disallow using navigation (links, goto, pushState, replaceState) without the base path | |
svelte/valid-prop-names-in-kit-pages | disallow props other than data or errors in SvelteKit page components. | :star: |
:warning: These rules are considered experimental and may change or be removed in the future:
Rule ID | Description | |
---|---|---|
svelte/experimental-require-slot-types | require slot type declaration using the $$Slots interface | |
svelte/experimental-require-strict-events | require the strictEvents attribute on <script> tags |
These rules relate to this plugin works:
Rule ID | Description | |
---|---|---|
svelte/comment-directive | support comment-directives in HTML template | :star: |
svelte/system | system rule for working this plugin | :star: |
Rule ID | Replaced by |
---|---|
svelte/@typescript-eslint/no-unnecessary-condition | This rule is no longer needed when using svelte-eslint-parser>=v0.19.0. |
svelte/no-dynamic-slot-name | Now Svelte compiler itself throws an compile error. |
svelte/no-goto-without-base | svelte/no-navigation-without-base |
Contributions are welcome! Please open an issue or submit a PR. For more details, see CONTRIBUTING.md.
Refer to svelte-eslint-parser for AST details.
See LICENSE (MIT).
FAQs
ESLint plugin for Svelte using AST
The npm package eslint-plugin-svelte receives a total of 422,164 weekly downloads. As such, eslint-plugin-svelte popularity was classified as popular.
We found that eslint-plugin-svelte demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket uncovered four malicious npm packages that exfiltrate up to 85% of a victim’s Ethereum or BSC wallet using obfuscated JavaScript.
Security News
TC39 advances 9 JavaScript proposals, including Array.fromAsync, Error.isError, and Explicit Resource Management, which are now headed into the ECMAScript spec.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.