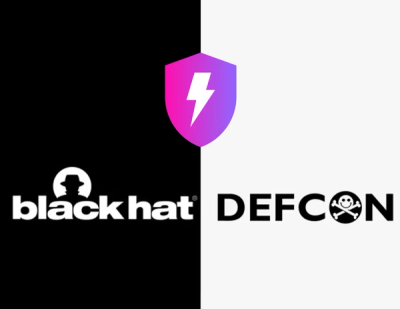
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
excel-row-stream
Advanced tools
Fast and simple transform stream for excel file parsing
npm i excel-row-stream
Here is an example:
import { createReadStream } from "fs";
import { Writable } from "stream";
import { pipeline } from "stream/promises";
import createExcelWorkbookStream, { Row } from "excel-row-stream";
const fileStream = createReadStream("./some.xlsx");
const workbookStream = createExcelWorkbookStream({
matchSheet: /sheet name/i,
dropEmptyRows: true,
});
const resultStream = new Writable({
objectMode: true,
write(row: Row, _encoding, callback) {
console.log(row.index, row.values);
callback();
},
});
await pipeline(fileStream, workbookStream, resultStream);
console.log("Done!");
The workbookStream
will only return rows from matched sheets.
hh:mm
format (without seconds) in excel but the underlying data has more resolution. Defaults to true
, because this library is intended for data analysis, not replicating what the user saw in Excel. Also because this is what pandas would do. This is only applied to when the time field has a format type of CUSTOM . Which is what Excel automatically applies when it infers a field is a time field.All row.values
have unknown
type. Please always validate your data. For example, you can do it with the excellent io-ts library.
This library provides several streams to make your life easier
Creates a stream that converts rows with values into objects with column names. The column names come from the first row (index = 1).
Options: ā sanitizeColumnName optional function to transform column names.
const fileStream = createReadStream("file.xlsx");
const parserStream = createExcelParserStream({
matchSheet: /.*/,
dropEmptyRows: true,
});
const withColumnsStream = createRowToRowWithColumnsStream({
sanitizeColumnName: (columnName) =>
columnName.toLowerCase().replace(/\W/g, "_"),
});
const resultStream = new Writable({
objectMode: true,
write(row: RowWithColumns, _encoding, callback) {
console.log(row.index, row.columns);
callback();
},
});
await pipeline(fileStream, parserStream, withColumnsStream, resultStream);
Creates a stream that strips the index
from rows and returns the data directly, either values
or columns
.
const fileStream = createReadStream("file.xlsx");
const parserStream = createExcelParserStream({
matchSheet: /.*/,
dropEmptyRows: true,
});
const asObjectsStream = createRowToRowAsObjectStream();
const resultStream = new Writable({
objectMode: true,
write(row: unknown[], _encoding, callback) {
console.log("values", row);
callback();
},
});
await pipeline(fileStream, parserStream, asObjectsStream, resultStream);
Creates a stream that checks if no data flows through it and throws an error with message
.
const fileStream = createReadStream("file.xlsx");
const parserStream = createExcelParserStream({
matchSheet: /.*/,
dropEmptyRows: true,
});
const filterStream = new Transform({
objectMode: true,
write(row: RowWithValues, _encoding, callback) {
// skip all the data
callback();
},
});
const throwIfEmpty = createThrowIfEmptyStream({
message: "Can not believe it",
});
// will throw
await pipeline(fileStream, parserStream, filterStream, throwIfEmpty);
License
FAQs
Fast and simple transform stream for excel files parsing
The npm package excel-row-stream receives a total of 20 weekly downloads. As such, excel-row-stream popularity was classified as not popular.
We found that excel-row-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.