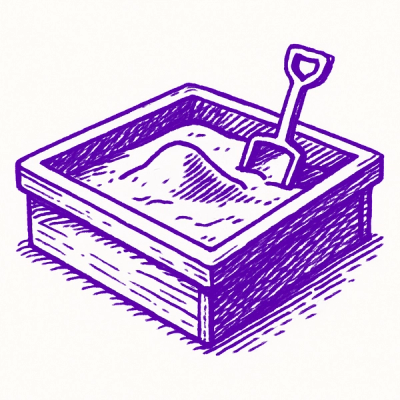
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
expo-auth-session
Advanced tools
The expo-auth-session package is a library for handling authentication flows in Expo and React Native applications. It provides tools to manage OAuth 2.0 and OpenID Connect authentication, making it easier to integrate with various identity providers.
OAuth 2.0 Authentication
This feature allows you to initiate an OAuth 2.0 authentication flow. The code sample demonstrates how to start an authentication session using a specified authorization URL and handle the result.
import * as AuthSession from 'expo-auth-session';
const authUrl = 'https://example.com/auth';
const redirectUrl = AuthSession.makeRedirectUri();
async function authenticate() {
const result = await AuthSession.startAsync({ authUrl, returnUrl: redirectUrl });
if (result.type === 'success') {
console.log('Authentication successful:', result.params);
}
}
OpenID Connect Support
This feature supports OpenID Connect, which is an identity layer on top of OAuth 2.0. The code sample shows how to use the discovery document to configure an authentication request and handle the response.
import * as AuthSession from 'expo-auth-session';
const discovery = AuthSession.useAutoDiscovery('https://example.com');
async function authenticateWithOIDC() {
const request = new AuthSession.AuthRequest({
clientId: 'your-client-id',
scopes: ['openid', 'profile'],
redirectUri: AuthSession.makeRedirectUri(),
});
const result = await AuthSession.startAsync({ authUrl: request.makeAuthUrl(), returnUrl: request.redirectUri });
if (result.type === 'success') {
console.log('OIDC Authentication successful:', result.params);
}
}
Token Exchange
This feature allows you to exchange an authorization code for an access token. The code sample demonstrates how to perform a token exchange using the authorization code obtained from a successful authentication.
import * as AuthSession from 'expo-auth-session';
async function exchangeToken(authCode) {
const tokenResponse = await AuthSession.exchangeCodeAsync({
clientId: 'your-client-id',
code: authCode,
redirectUri: AuthSession.makeRedirectUri(),
extraParams: {
client_secret: 'your-client-secret',
},
});
console.log('Access Token:', tokenResponse.accessToken);
}
react-native-app-auth is a library for OAuth 2.0 and OpenID Connect authentication in React Native applications. It provides a more native experience by using native modules for iOS and Android, which can result in better performance and reliability compared to expo-auth-session, which is more suitable for Expo managed workflows.
Passport is a popular authentication middleware for Node.js. It supports a wide range of authentication strategies, including OAuth 2.0 and OpenID Connect. While it is not specifically designed for React Native, it can be used in conjunction with a backend server to handle authentication flows, offering more flexibility and control compared to expo-auth-session.
oidc-client is a JavaScript library for managing OpenID Connect and OAuth 2.0 authentication. It is primarily used in web applications but can be adapted for use in React Native. It provides more comprehensive support for OpenID Connect features compared to expo-auth-session, which is more focused on ease of use in Expo environments.
AuthSession
is the easiest way to implement web browser based authentication (for example, browser-based OAuth flows) to your app, built on top of expo-web-browser.
For managed Expo projects, please follow the installation instructions in the API documentation for the latest stable release.
For bare React Native projects, you must ensure that you have installed and configured the expo
package before continuing.
npx expo install expo-auth-session expo-crypto
To use this module, you need to set up React Native deep linking in your application. For more information, check out React Native documentation.
Android:
Add intent filter and set the launchMode
of your MainActivity to singleTask
in AndroidManifest.yml
:
<activity
android:name=".MainActivity"
android:launchMode="singleTask">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accepts URIs that begin with "example://gizmos” -->
<data android:scheme="example"
android:host="gizmos" />
</intent-filter>
For more information about the available configuration, check out Android documentation.
iOS:
Add following lines to your AppDelegate.m
:
#import <React/RCTLinkingManager.h>
// iOS 9.x or newer
- (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options
{
return [RCTLinkingManager application:application openURL:url options:options];
}
// iOS 8.x or older
- (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
sourceApplication:(NSString *)sourceApplication
annotation:(id)annotation
{
return [RCTLinkingManager application:application openURL:url
sourceApplication:sourceApplication annotation:annotation];
}
Add following lines to Info.plist
:
<dict>
...
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLName</key>
<string>gizmos</string>
<key>CFBundleURLSchemes</key>
<array>
<string>example</string>
</array>
</dict>
</array>
</dict>
Contributions are very welcome! Please refer to guidelines described in the contributing guide.
FAQs
Expo module for browser-based authentication
The npm package expo-auth-session receives a total of 274,803 weekly downloads. As such, expo-auth-session popularity was classified as popular.
We found that expo-auth-session demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 27 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.