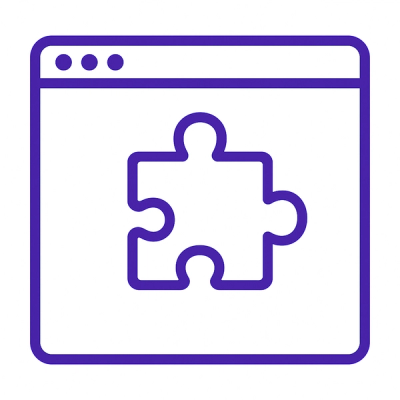
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
express-mongo-sanitize
Advanced tools
Sanitize your express payload to prevent MongoDB operator injection.
The express-mongo-sanitize npm package is designed to prevent MongoDB Operator Injection attacks by sanitizing user-supplied data. It removes any keys that start with '$' or contain a '.' from objects, which are common vectors for injection attacks.
Sanitize Request Body
This feature sanitizes the request body to remove any keys that start with '$' or contain a '.', preventing MongoDB Operator Injection attacks.
const express = require('express');
const mongoSanitize = require('express-mongo-sanitize');
const app = express();
// Middleware to sanitize request body
app.use(express.json());
app.use(mongoSanitize());
app.post('/data', (req, res) => {
res.send('Data received safely');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Sanitize Request Query
This feature sanitizes the request query parameters to remove any keys that start with '$' or contain a '.', replacing them with an underscore ('_').
const express = require('express');
const mongoSanitize = require('express-mongo-sanitize');
const app = express();
// Middleware to sanitize request query
app.use(mongoSanitize.sanitize({
replaceWith: '_'
}));
app.get('/search', (req, res) => {
res.send('Query sanitized');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Sanitize Request Params
This feature sanitizes the request parameters to remove any keys that start with '$' or contain a '.', and logs a warning when a key is sanitized.
const express = require('express');
const mongoSanitize = require('express-mongo-sanitize');
const app = express();
// Middleware to sanitize request params
app.use(mongoSanitize.sanitize({
onSanitize: ({ req, key }) => {
console.warn(`This request[${key}] is sanitized`, req);
}
}));
app.get('/user/:id', (req, res) => {
res.send('Params sanitized');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
The mongo-sanitize package is a lightweight library that removes any keys containing '$' or '.' from objects, similar to express-mongo-sanitize. However, it does not provide middleware for Express applications and must be used manually to sanitize objects.
The sanitize package provides a broader range of sanitization functions, including HTML sanitization and SQL injection prevention. While it can be used to sanitize MongoDB queries, it is not specifically designed for MongoDB and does not offer the same focused functionality as express-mongo-sanitize.
The validator package is a library of string validators and sanitizers. It includes functions to escape and sanitize strings, which can be used to prevent injection attacks. However, it does not specifically target MongoDB operator injection and requires more manual implementation compared to express-mongo-sanitize.
Express 4.x middleware which sanitizes user-supplied data to prevent MongoDB Operator Injection.
This module searches for any keys in objects that begin with a $
sign or contain a .
, from req.body
, req.query
or req.params
. It can then either:
The behaviour is governed by the passed option, replaceWith
. Set this option to have the sanitizer replace the prohibited characters with the character passed in.
The config option allowDots
can be used to allow dots in the user-supplied data. In this case, only instances of $
will be sanitized.
See the spec file for more examples.
Object keys starting with a $
or containing a .
are reserved for use by MongoDB as operators. Without this sanitization, malicious users could send an object containing a $
operator, or including a .
, which could change the context of a database operation. Most notorious is the $where
operator, which can execute arbitrary JavaScript on the database.
The best way to prevent this is to sanitize the received data, and remove any offending keys, or replace the characters with a 'safe' one.
npm install express-mongo-sanitize
Add as a piece of express middleware, before defining your routes.
const express = require('express');
const bodyParser = require('body-parser');
const mongoSanitize = require('express-mongo-sanitize');
const app = express();
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// By default, $ and . characters are removed completely from user-supplied input in the following places:
// - req.body
// - req.params
// - req.headers
// - req.query
// To remove data using these defaults:
app.use(mongoSanitize());
// Or, to replace these prohibited characters with _, use:
app.use(
mongoSanitize({
replaceWith: '_',
}),
);
// Or, to sanitize data that only contains $, without .(dot)
// Can be useful for letting data pass that is meant for querying nested documents.
// NOTE: This may cause some problems on older versions of MongoDb
// READ MORE: https://github.com/fiznool/express-mongo-sanitize/issues/36
app.use(
mongoSanitize({
allowDots: true,
}),
);
// Both allowDots and replaceWith
app.use(
mongoSanitize({
allowDots: true,
replaceWith: '_',
}),
);
onSanitize
onSanitize
callback is called after the request's value was sanitized.
app.use(
mongoSanitize({
onSanitize: ({ req, key }) => {
console.warn(`This request[${key}] is sanitized`, req);
},
}),
);
dryRun
You can run this middleware as dry run mode.
app.use(
mongoSanitize({
dryRun: true,
onSanitize: ({ req, key }) => {
console.warn(`[DryRun] This request[${key}] will be sanitized`, req);
},
}),
);
You can also bypass the middleware and use the module directly:
const mongoSanitize = require('express-mongo-sanitize');
const payload = {...};
// Remove any keys containing prohibited characters
mongoSanitize.sanitize(payload);
// Replace any prohibited characters in keys
mongoSanitize.sanitize(payload, {
replaceWith: '_'
});
// Exclude sanitization of . (dot), only sanitize data that contains $.
// NOTE: This may cause some problems on older versions of MongoDb
// READ MORE: https://github.com/fiznool/express-mongo-sanitize/issues/36
mongoSanitize.sanitize(payload, {
allowDots: true
});
// Both allowDots and replaceWith
mongoSanitize.sanitize(payload, {
allowDots: true,
replaceWith: '_'
});
// Check if the payload has keys with prohibited characters
const hasProhibited = mongoSanitize.has(payload);
// Check if the payload has keys with prohibited characters (`.` is excluded).
// If the payload only has `.` it will return false (since it doesn't see the data with `.` as malicious)
const hasProhibited = mongoSanitize.has(payload, true);
PRs are welcome! Please add test coverage for any new features or bugfixes, and make sure to run npm run prettier
before submitting a PR to ensure code consistency.
Inspired by mongo-sanitize.
MIT
[2.2.0] - 2022-01-14
config
option:
allowDots
boolean: if set, allows dots in the user-supplied data #41dryRun
option #88FAQs
Sanitize your express payload to prevent MongoDB operator injection.
The npm package express-mongo-sanitize receives a total of 163,418 weekly downloads. As such, express-mongo-sanitize popularity was classified as popular.
We found that express-mongo-sanitize demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.