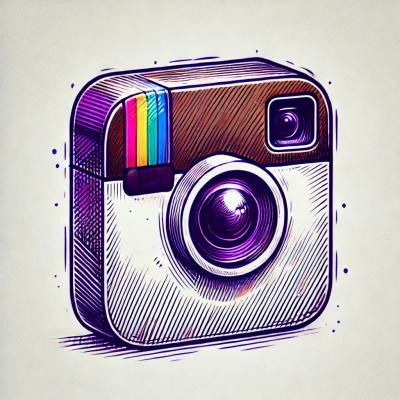
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
extra-iterable
Advanced tools
An iterable is a sequence of values.
š¦ Node.js,
š Web,
š Files,
š° Docs,
š Wiki.
This is a collection of functions for operating upon iterables. Assumption here is that an iterable can only be iterated over once. Methods which require multiple iterations preserve old values in a backup array using toMany. Many methods accept both compare and map functions, and in some cases using only a map function enables faster comparision (like unique). I borrowed a lot of ideas from Haskell, Elm, Python, Basic, Lodash, and other NPM packages. These are mentioned in references of each method.
This package is available in Node.js and Web formats. To use it on the web,
simply use the extra_iterable
global variable after loading with a <script>
tag from the jsDelivr CDN.
Stability: Experimental.
const xiterable = require('extra-iterable');
// import * as xiterable from "extra-iterable";
// import * as xiterable from "https://unpkg.com/extra-iterable/index.mjs"; (deno)
var x = [2, 4, 6, 8];
xiterable.get(x, 1);
// ā 4
var x = [1, 2, 3, 4];
[...xiterable.swap(x, 0, 1)];
// ā [ 2, 1, 3, 4 ]
var x = [1, 2, 3];
[...xiterable.cycle(x, 0, 4)];
// ā [1, 2, 3, 1]
var x = [1, 2, 3, 4];
xiterable.reduce(x, (acc, v) => acc+v);
// ā 10
Property | Description |
---|---|
is | Check if value is an iterable. |
isIterator | Check if value is an iterator. |
isList | Check if value is a list (iterable & !string). |
iterator | Get iterator of an iterable. |
keys | List all indices. |
values | List all values. |
entries | List all index-value pairs. |
from | Convert an iterable-like to iterable. |
fromIterator | Convert an iterator to iterable. |
fromRange | Generate iterable from given number range. |
fromInvocation | Generate iterable from repeated function invocation. |
fromApplication | Generate iterable from repeated function application. |
isOnce | Check if an iterable can iterated only once. |
isMany | Check if an iterable can be iterated many times. |
toMany | Convert a once-like iterable to many. |
toInvokable | Generate a function that iterates over values upon invocation. |
isEmpty | Check if an iterable is empty. |
length | Find the length of an iterable. |
compare | Compare two iterables. |
isEqual | Check if two iterables are equal. |
index | Get zero-based index for element in iterable. |
indexRange | Get index range for part of iterable. |
get | Get value at index. |
getAll | Get values at indices. |
getPath | Get value at path in a nested iterable. |
hasPath | Check if nested iterable has a path. |
set | Set value at index. |
swap | Exchange two values. |
remove | Remove value at index. |
count | Count values which satisfy a test. |
countAs | Count occurrences of values. |
min | Find smallest value. |
max | Find largest value. |
range | Find smallest and largest values. |
minEntry | Find smallest entry. |
maxEntry | Find largest entry. |
rangeEntries | Find smallest and largest entries. |
slice | Get part of an iterable. |
head | Get first value. |
last | Get last value. |
tail | Get values except first. |
init | Get values except last. |
left | Get values from left. |
right | Get values from right. |
middle | Get values from middle. |
take | Keep first n values only. |
takeRight | Keep last n values only. |
takeWhile | Keep values from left, while a test passes. |
takeWhileRight | Keep values from right, while a test passes. |
drop | Discard first n values only. |
dropRight | Discard last n values only. |
dropWhile | Discard values from left, while a test passes. |
dropWhileRight | Discard values from right, while a test passes. |
includes | Check if iterable has a value. |
indexOf | Find first index of a value. |
lastIndexOf | Find last index of a value. |
find | Find first value passing a test. |
findRight | Find last value passing a test. |
scanWhile | Scan from left, while a test passes. |
scanWhileRight | Scan from right, while a test passes. |
scanUntil | Scan from left, until a test passes. |
scanUntilRight | Scan from right, until a test passes. |
search | Find index of first value passing a test. |
searchRight | Find index of last value passing a test. |
searchAll | Find indices of values passing a test. |
searchValue | Find first index of a value. |
searchValueRight | Find last index of a value. |
searchValueAll | Find indices of a value. |
searchInfix | Find first index of an infix. |
searchInfixRight | Find last index of an infix. |
searchInfixAll | Find indices of an infix. |
searchSubsequence | Find first index of a subsequence. |
hasValue | Check if iterable has a value. |
hasPrefix | Check if iterable starts with a prefix. |
hasSuffix | Check if iterable ends with a suffix. |
hasInfix | Check if iterable contains an infix. |
hasSubsequence | Check if iterable has a subsequence. |
forEach | Call a function for each value. |
some | Check if any value satisfies a test. |
every | Check if all values satisfy a test. |
map | Transform values of an iterable. |
reduce | Reduce values of iterable to a single value. |
filter | Keep the values which pass a test. |
filterAt | Keep the values at given indices. |
reject | Discard the values which pass a test. |
rejectAt | Discard the values at given indices. |
accumulate | Produce accumulating values. |
flat | Flatten nested iterable to given depth. |
flatMap | Flatten nested iterable, based on map function. |
zip | Combine values from iterables. |
fill | Fill with given value. |
push | Add values to the end. |
unshift | Add values to the start. |
copy | Copy part of iterable to another. |
copyWithin | Copy part of iterable within. |
moveWithin | Move part of iterable within. |
splice | Remove or replaces existing values. |
split | Break iterable considering test as separator. |
splitAt | Break iterable considering indices as separator. |
cut | Break iterable when test passes. |
cutRight | Break iterable after test passes. |
cutAt | Break iterable at given indices. |
cutAtRight | Break iterable after given indices. |
group | Keep similar values together and in order. |
partition | Segregate values by test result. |
partitionAs | Segregate values by similarity. |
chunk | Break iterable into chunks of given size. |
cycle | Obtain values that cycle through an iterable. |
repeat | Repeat an iterable given times. |
reverse | Reverse the values. |
rotate | Rotate values in iterable. |
intersperse | Place a separator between every value. |
interpolate | Estimate new values between existing ones. |
intermix | Place values of an iterable between another. |
interleave | Place values from iterables alternately. |
concat | Append values from iterables. |
merge | Merge values from sorted iterables. |
join | Join values together into a string. |
isUnique | Check if there are no duplicate values. |
isDisjoint | Checks if arrays have no value in common. |
unique | Remove duplicate values. |
union | Obtain values present in any iterable. |
intersection | Obtain values present in both iterables. |
difference | Obtain values not present in another iterable. |
symmetricDifference | Obtain values not present in both iterables. |
cartesianProduct | List cartesian product of iterables. |
FAQs
An iterable is a sequence of values.
The npm package extra-iterable receives a total of 797 weekly downloads. As such, extra-iterable popularity was classified as not popular.
We found that extra-iterable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.