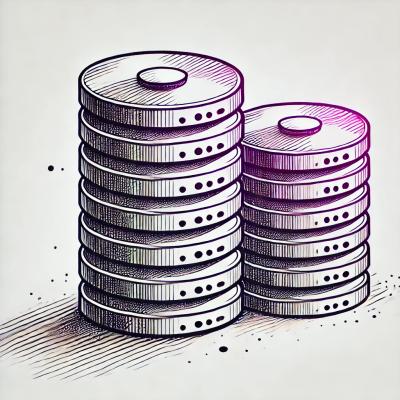
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
falcor-path-utils
Advanced tools
This repository contains utilities for transforming and manipulating Falcor paths.
This repository contains utilities for transforming and manipulating Falcor paths.
collapse(paths)
Simplifies a set of paths. Example:
var util = require("falcor-path-utils");
var collapsedPaths = util.collapse([
["genres", 0, "titles", 0, "name"],
["genres", 0, "titles", 0, "rating"],
["genres", 0, "titles", 1, "name"],
["genres", 0, "titles", 1, "rating"]
]);
// collapsed paths is ["genres", 0, "titles", {from: 0, to: 1}, ["name", "rating"]]
iterateKeySet(keySet, note)
Takes in a keySet
and a note
and attempts to iterate over it.
toTree(paths)
Converts paths
to a tree with null leaves. (see spec)
toPaths(lengths)
Converts a lengthTree
to paths. (see spec)
pathsComplementFromTree(paths, tree)
Returns a list of these paths
that are not in the tree
. (see spec)
pathsComplementFromLengthTree(paths, lengthTree)
Like above, but for use with length tree.
hasIntersection(tree, path, depth)
Tests to see if the intersection should be stripped from the total paths.
optimizePathSets(cache, paths, maxRefFollow)
(see spec)
pathCount(pathSet)
Returns the number of paths in a PathSet.
var util = require("falcor-path-utils");
console.log(util.pathCount(["titlesById", [512, 628], ["name","rating"]]))
// prints 4, because ["titlesById", [512, 628], ["name","rating"]] contains...
// ["titlesById", 512, "name"]
// ["titlesById", 512, "rating"]
// ["titlesById", 628, "name"]
// ["titlesById", 628, "rating"]
escape(string)
Escapes untrusted input to make it safe to include in a path.
unescape(string)
Unescapes a string encoded with escape.
materialize(pathSet, value)
Construct a JsonGraph of value at pathSet paths.
FAQs
This repository contains utilities for transforming and manipulating Falcor paths.
The npm package falcor-path-utils receives a total of 0 weekly downloads. As such, falcor-path-utils popularity was classified as not popular.
We found that falcor-path-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 16 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.