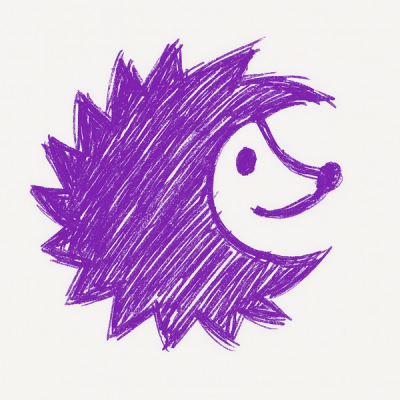
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
fb-graph-api
Advanced tools
With fb-graph-api you can now easily make requests to Facebook's Graph API.
Author: CoericK
License: Apache v2
npm install --save fb-graph-api
yarn add fb-graph-api
// Using require() in ES5
var FBGraphAPI = require('fb-graph-api');
// Using ES2015 import through Babel
import FBGraphAPI from 'fb-graph-api';
// ES5
var FB = new FBGraphAPI({
clientID: '...',
clientSecret: '...',
appAccessToken: '...' // Optional
});
// ES2015 w/ import through Babel
const FB = new FBGraphAPI({
clientID: '...',
clientSecret: '...',
appAccessToken: '...' // Optional
});
Generates the App Access Token for you.
FB.generateAppAccessToken()
.then(appAccessToken => {
console.log('appAccessToken', appAccessToken);
})
.catch(e => console.log('e', e));
Debugs a given token using the App Access Token.
FB.debugToken('EAAJ3bm5M....')
.then(data => {
console.log('debuggedToken', data);
/*
* data would look like this...
{
"app_id": 000000000000000,
"application": "Social Cafe",
"expires_at": 1352419328,
"is_valid": true,
"issued_at": 1347235328,
"scopes": [
"email",
"publish_actions"
],
"user_id": 1207059
}
*/
})
.catch(e => console.log('e', e));
Debugs a given token using the App Access Token and checks if the given token was crated for the App Access Token that was given.
FB.isValid('EAAJ3bm5M....')
.then(valid => {
console.log('valid', valid); // true or false
})
.catch(e => console.log('e', e));
FAQs
NodeJS Library for Facebook Graph API
The npm package fb-graph-api receives a total of 3 weekly downloads. As such, fb-graph-api popularity was classified as not popular.
We found that fb-graph-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.