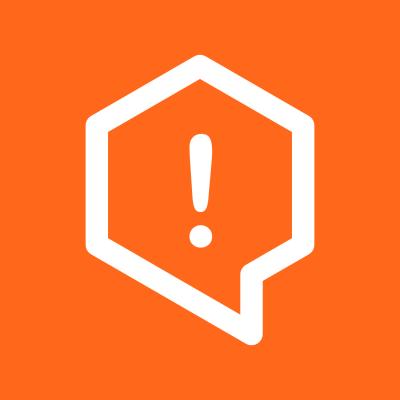
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
The 'fecha' npm package is a lightweight JavaScript library for parsing, formatting, and manipulating dates. It provides a simple and flexible API for handling date and time operations.
Date Formatting
This feature allows you to format JavaScript Date objects into strings using various format patterns. The example formats the current date into 'YYYY-MM-DD' format.
const fecha = require('fecha');
const formattedDate = fecha.format(new Date(), 'YYYY-MM-DD');
console.log(formattedDate); // Outputs: 2023-10-05
Date Parsing
This feature allows you to parse date strings into JavaScript Date objects using specified format patterns. The example parses the string '2023-10-05' into a Date object.
const fecha = require('fecha');
const parsedDate = fecha.parse('2023-10-05', 'YYYY-MM-DD');
console.log(parsedDate); // Outputs: Thu Oct 05 2023 00:00:00 GMT+0000 (Coordinated Universal Time)
Custom Formatting Tokens
This feature allows you to define custom formatting tokens for date formatting. The example defines a custom token 'custom' for time formatting and uses it to format the current time.
const fecha = require('fecha');
fecha.masks.custom = 'HH:mm:ss';
const customFormattedDate = fecha.format(new Date(), 'custom');
console.log(customFormattedDate); // Outputs: current time in 'HH:mm:ss' format
Moment.js is a comprehensive library for parsing, validating, manipulating, and formatting dates. It offers a wide range of features and is widely used, but it is larger in size compared to 'fecha'.
date-fns provides a set of functions for manipulating JavaScript dates in a functional programming style. It is modular and tree-shakeable, making it a lightweight alternative to Moment.js.
Day.js is a minimalist JavaScript library that parses, validates, manipulates, and displays dates and times. It is similar to Moment.js but much smaller in size, making it a good alternative for performance-sensitive applications.
Lightweight date formatting and parsing (~2KB). Meant to replace parsing and formatting functionality of moment.js.
npm install fecha --save
yarn add fecha
Fecha | Moment | |
---|---|---|
Size (Min. and Gzipped) | 2.1KBs | 13.1KBs |
Date Parsing | ✓ | ✓ |
Date Formatting | ✓ | ✓ |
Date Manipulation | ✓ | |
I18n Support | ✓ | ✓ |
format
accepts a Date object (or timestamp) and a string format and returns a formatted string. See below for
available format tokens.
Note: format
will throw an error when passed invalid parameters
import { format } from 'fecha';
type format = (date: Date, format?: string, i18n?: I18nSettings) => str;
// Custom formats
format(new Date(2015, 10, 20), 'dddd MMMM Do, YYYY'); // 'Friday November 20th, 2015'
format(new Date(1998, 5, 3, 15, 23, 10, 350), 'YYYY-MM-DD hh:mm:ss.SSS A'); // '1998-06-03 03:23:10.350 PM'
// Named masks
format(new Date(2015, 10, 20), 'isoDate'); // '2015-11-20'
format(new Date(2015, 10, 20), 'mediumDate'); // 'Nov 20, 2015'
format(new Date(2015, 10, 20, 3, 2, 1), 'isoDateTime'); // '2015-11-20T03:02:01-05:00'
format(new Date(2015, 2, 10, 5, 30, 20), 'shortTime'); // '05:30'
// Literals
format(new Date(2001, 2, 5, 6, 7, 2, 5), '[on] MM-DD-YYYY [at] HH:mm'); // 'on 03-05-2001 at 06:07'
parse
accepts a Date string and a string format and returns a Date object. See below for available format tokens.
NOTE: parse
will throw an error when passed invalid string format or missing format. You MUST specify a format.
import { parse } from 'fecha';
type parse = (dateStr: string, format: string, i18n?: I18nSettingsOptional) => Date|null;
// Custom formats
parse('February 3rd, 2014', 'MMMM Do, YYYY'); // new Date(2014, 1, 3)
parse('10-12-10 14:11:12', 'YY-MM-DD HH:mm:ss'); // new Date(2010, 11, 10, 14, 11, 12)
// Named masks
parse('5/3/98', 'shortDate'); // new Date(1998, 4, 3)
parse('November 4, 2005', 'longDate'); // new Date(2005, 10, 4)
parse('2015-11-20T03:02:01-05:00', 'isoDateTime'); // new Date(2015, 10, 20, 3, 2, 1)
// Override i18n
parse('4 de octubre de 1983', 'M de MMMM de YYYY', {
monthNames: [
'enero',
'febrero',
'marzo',
'abril',
'mayo',
'junio',
'julio',
'agosto',
'septiembre',
'octubre',
'noviembre',
'diciembre'
]
}); // new Date(1983, 9, 4)
import {setGlobalDateI18n} from 'fecha';
/*
Default I18n Settings
{
dayNamesShort: ['Sun', 'Mon', 'Tue', 'Wed', 'Thur', 'Fri', 'Sat'],
dayNames: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'],
monthNamesShort: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'],
monthNames: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'],
amPm: ['am', 'pm'],
// D is the day of the month, function returns something like... 3rd or 11th
DoFn(dayOfMonth) {
return dayOfMonth + [ 'th', 'st', 'nd', 'rd' ][ dayOfMonth % 10 > 3 ? 0 : (dayOfMonth - dayOfMonth % 10 !== 10) * dayOfMonth % 10 ];
}
}
*/
setGlobalDateI18n({
dayNamesShort: ['Sun', 'Mon', 'Tue', 'Wed', 'Thur', 'Fri', 'Sat'],
dayNames: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'],
monthNamesShort: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'],
monthNames: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'],
amPm: ['am', 'pm'],
// D is the day of the month, function returns something like... 3rd or 11th
DoFn: function (D) {
return D + [ 'th', 'st', 'nd', 'rd' ][ D % 10 > 3 ? 0 : (D - D % 10 !== 10) * D % 10 ];
}
});
import { format, setGlobalDateMasks } from 'fecha';
/*
Default global masks
{
default: 'ddd MMM DD YYYY HH:mm:ss',
shortDate: 'M/D/YY',
mediumDate: 'MMM D, YYYY',
longDate: 'MMMM D, YYYY',
fullDate: 'dddd, MMMM D, YYYY',
shortTime: 'HH:mm',
mediumTime: 'HH:mm:ss',
longTime: 'HH:mm:ss.SSS'
}
*/
// Create a new mask
setGlobalDateMasks({
myMask: 'HH:mm:ss YY/MM/DD';
});
// Use it
format(new Date(2014, 5, 6, 14, 10, 45), 'myMask'); // '14:10:45 14/06/06'
Token | Output | |
---|---|---|
Month | M | 1 2 ... 11 12 |
MM | 01 02 ... 11 12 | |
MMM | Jan Feb ... Nov Dec | |
MMMM | January February ... November December | |
Day of Month | D | 1 2 ... 30 31 |
Do | 1st 2nd ... 30th 31st | |
DD | 01 02 ... 30 31 | |
Day of Week | d | 0 1 ... 5 6 |
ddd | Sun Mon ... Fri Sat | |
dddd | Sunday Monday ... Friday Saturday | |
Year | YY | 70 71 ... 29 30 |
YYYY | 1970 1971 ... 2029 2030 | |
AM/PM | A | AM PM |
a | am pm | |
Hour | H | 0 1 ... 22 23 |
HH | 00 01 ... 22 23 | |
h | 1 2 ... 11 12 | |
hh | 01 02 ... 11 12 | |
Minute | m | 0 1 ... 58 59 |
mm | 00 01 ... 58 59 | |
Second | s | 0 1 ... 58 59 |
ss | 00 01 ... 58 59 | |
Fractional Second | S | 0 1 ... 8 9 |
SS | 0 1 ... 98 99 | |
SSS | 0 1 ... 998 999 | |
Timezone | Z | -07:00 -06:00 ... +06:00 +07:00 |
ZZ | -0700 -0600 ... +0600 +0700 |
4.2.3
FAQs
Date formatting and parsing
The npm package fecha receives a total of 8,640,992 weekly downloads. As such, fecha popularity was classified as popular.
We found that fecha demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.