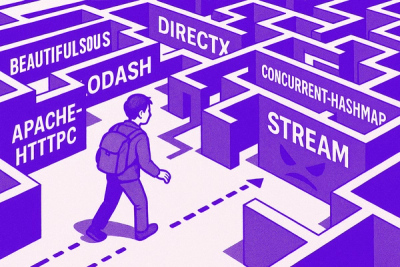
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
file2md5 is a browser-side implementation of file conversion to md5 format based on SparkMD5, which supports typescript friendly.
file2md5 is a browser-side implementation of file conversion to md5 format based on SparkMD5, which supports typescript friendly.
Supports Typescript
Promise API
All browsers supported
Npm
npm install file2md5 --save
Yarn
yarn add file2md5
Html:
<input type="file" id="upload" />
Javascript:
import file2md5 from 'file2md5';
const el = document.querySelector('#upload');
const onProgress = progress => console.log('progress', progress);
const onChange = async e => {
if (!e.target.files || !e.target.files[0]) {
return;
}
const file = e.target.files[0];
const abort = file2md5.abort;
// Assuming that it takes 3s to convert the md5 value,
// the simulation terminates the operation at 1s
setTimeout(
() => abort(),
1000
);
try {
const md5 = await file2md5(file, {chunkSize: 3 * 1024 * 1024, onProgress});
console.log('md5 string:', md5); // d18bb890790c4335e57eadbedc801c2c
}
catch (e) {
console.error('error', e);
}
};
el.addEventListener(
'change',
onChange,
false
);
You can call the abort method to terminate before the end of the file conversion to the md5 value.
const abort = file2md5.abort;
abort();
Name | Required | Description | Type | Default value |
---|---|---|---|---|
file | true | The file is used to convert md5 format. | File | - |
options | false | Optional configuration items. | IOptions | {} |
Name | Required | Description | Type | Default value |
---|---|---|---|---|
chunkSize | false | The conversion is performed in chunks, so you can customize the size of each chunk. | number | 2 * 1024 * 1024 |
raw | false | If raw is true, the result as a binary string will be returned instead. | boolean | false |
onProgress | false | Callback function for monitoring progress. | (progress: number) => unknown | - |
file2md5 is licensed under a MIT License
FAQs
file2md5 is a browser-side implementation of file conversion to md5 format based on SparkMD5, which supports typescript friendly.
The npm package file2md5 receives a total of 361 weekly downloads. As such, file2md5 popularity was classified as not popular.
We found that file2md5 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.