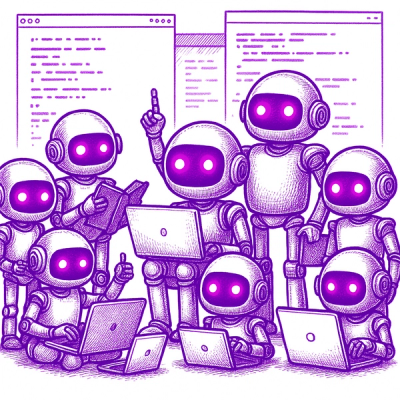
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600Ć Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
filemanagementsystemfornode
Advanced tools
A file management system for node.js application making managements of files easier, all in one code, you can delete, move, etc, files all in one code, making ability to manage a system eg a application requiring transfering of files in folders easier
A powerful, flexible, and chainable file management system for Node.js.
A powerful, flexible, and chainable file management system for Node.js.
This system provides an easy way to manage files and directories while supporting:
ā Rename, Move, Copy, and Delete files
ā Append content to files
ā Retrieve compressed file content (getInfo()
)
ā Retrieve file metadata (getMetaInfo()
)
ā Create, Delete, and List directories
ā Check if a file/folder exists (syncExist()
)
ā Custom error handling (e.g., auto-create missing files on error)
ā Add custom chainable methods (addMethod()
)
ā Invoke custom chain methods dynamically (invokeMethod()
)
To install and use this package, run:
git clone https://github.com/Whitzzscott/FileManagement.git
cd FileManagement
npm install
This example demonstrates:
import { FileWrapper } from "./wrappers/FileWrapper";
const file = new FileWrapper("./testFolder/sample.txt");
// ā
Custom error handling: Auto-create missing files when they are not found
file.errorOnFound = (error) => {
if (error.code === "ENOENT") {
file.sync().writeFile("Default content");
console.log(`š File created: ${file.getPath()}`);
}
};
// ā
Add a custom method (e.g., logging the file path)
file.addMethod("logPath", function () {
console.log("File is at:", this.getPath());
return this;
});
// ā
Chainable file operations
(async () => {
file
.rename("renamed.txt") // Renames file
.invokeMethod("logPath") // Logs new file path
.move("./testFolder/moved") // Moves file to a new folder
.copy("./backup") // Creates a copy in the backup folder
.append("\nMore content added.") // Appends text to the file
.delete(); // Deletes the file
console.log("š Compressed File Content:", file.getInfo());
console.log("š File Metadata:", file.getMetaInfo());
console.log("š Files in directory:", file.list());
})();
This file management system offers multiple operations for handling files and folders.
Method | Description | Example |
---|---|---|
rename(newName: string) | Renames the file | file.rename("newName.txt") |
move(destination: string) | Moves the file to a new directory | file.move("./newFolder") |
copy(destination: string) | Copies the file to a new location | file.copy("./backup") |
delete() | Deletes the file permanently | file.delete() |
writeFile(content: string) | Writes new content to the file (overwrites existing content) | file.writeFile("Hello, world!") |
append(content: string) | Appends new content to the file | file.append("\nNew content") |
Method | Description | Example |
---|---|---|
syncExist() | Checks if a file or folder exists | if (file.syncExist()) {...} |
sync() | Ensures the directory exists (creates it if missing) | file.sync() |
list() | Lists all files in the directory | console.log(file.list()) |
Method | Description | Example |
---|---|---|
getInfo() | Reads and compresses the entire file content | console.log(file.getInfo()) |
getMetaInfo() | Retrieves metadata such as size, creation date, and modification date | console.log(file.getMetaInfo()) |
Method | Description | Example |
---|---|---|
errorOnFound = (error) => {...} | Custom error handling (e.g., auto-create files) | file.errorOnFound = (err) => {...} |
addMethod(name, method) | Adds a custom chainable method | file.addMethod("customMethod", function () {...}) |
invokeMethod(name, ...args) | Calls a custom method dynamically | file.invokeMethod("customMethod") |
npm install filemanagementsystemfornode
npm run start
src/main.ts
to test different operations.src/Wrapper
if necessary.This project is open-source and free to use.
We plan to add:
š Enjoy simplified file management with powerful chaining!
Feel free to suggest new features or improvements! šÆ
WhitzScott - Developer of the File Management System.
If you have questions or ideas, feel free to reach out!
FAQs
A file management system for node.js application making managements of files easier, all in one code, you can delete, move, etc, files all in one code, making ability to manage a system eg a application requiring transfering of files in folders easier
We found that filemanagementsystemfornode demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.