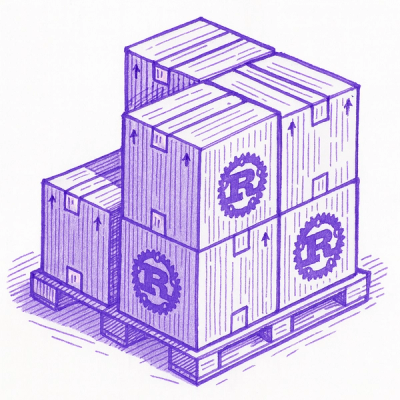
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Wrapper around miniglob / minimatch combo to allow multiple patterns matching and include-exclude ability
The fileset npm package allows you to find files in a directory that match a given set of glob patterns. It is useful for tasks such as file manipulation, batch processing, and automation scripts.
Basic File Matching
This feature allows you to find all JavaScript files in a directory, excluding those in the node_modules directory. The code sample demonstrates how to use the fileset function to match files based on glob patterns.
const fileset = require('fileset');
fileset('**/*.js', 'node_modules/**', function(err, files) {
if (err) return console.error(err);
console.log(files);
});
Multiple Patterns
This feature allows you to match files based on multiple patterns. The code sample shows how to find all JavaScript and CSS files in a directory, excluding those in the node_modules directory.
const fileset = require('fileset');
fileset(['**/*.js', '**/*.css'], 'node_modules/**', function(err, files) {
if (err) return console.error(err);
console.log(files);
});
Excluding Patterns
This feature allows you to exclude files based on patterns. The code sample demonstrates how to find all files in a directory, excluding those in the node_modules and dist directories.
const fileset = require('fileset');
fileset('**/*', ['node_modules/**', 'dist/**'], function(err, files) {
if (err) return console.error(err);
console.log(files);
});
The glob package is a popular alternative for matching files using glob patterns. It offers similar functionality to fileset but is more widely used and has a larger community. It also provides synchronous and asynchronous methods for file matching.
The fast-glob package is another alternative that focuses on performance. It is faster than both fileset and glob, especially for large sets of files. It also supports advanced features like concurrency and custom matching options.
The minimatch package is a lightweight alternative that provides basic glob pattern matching. It is often used as a dependency in other packages and is known for its simplicity and ease of use.
Exposes a basic wrapper on top of Glob / minimatch combo both written by @isaacs. Glob now uses JavaScript instead of C++ bindings which makes it usable in Node.js 0.6.x and Windows platforms.
Adds multiples patterns matching and exlude ability. This is basically just a sugar API syntax where you can specify a list of includes and optional exclude patterns. It works by setting up the necessary miniglob "fileset" and filtering out the results using minimatch.
npm install fileset
Can be used with callback or emitter style.
foo/**/*.js *.md src/lib/**/*
foo/**/*.js *.md
The callback is optional since the fileset method return an instance of EventEmitter which emit different events you might use:
var fileset = require('fileset');
fileset('**/*.js', '**.min.js', function(err, files) {
if (err) return console.error(err);
console.log('Files: ', files.length);
console.log(files);
});
var fileset = require('fileset');
fileset('**.coffee README.md *.json Cakefile **.js', 'node_modules/**')
.on('match', console.log.bind(console, 'error'))
.on('include', console.log.bind(console, 'includes'))
.on('exclude', console.log.bind(console, 'excludes'))
.on('end', console.log.bind(console, 'end'));
fileset
returns an instance of EventEmitter, with an includes
property
which is the array of Fileset objects (inheriting from
miniglob.Miniglob
) that were used during the mathing process, should
you want to use them individually.
Check out the tests for more examples.
Run npm test
Mainly for a build tool with cake files, to provide me an easy way to get a list of files by either using glob or path patterns, optionally allowing exclude patterns to filter out the results.
All the magic is happening in Glob and minimatch. Check them out!
0.1.8
FAQs
Wrapper around miniglob / minimatch combo to allow multiple patterns matching and include-exclude ability
The npm package fileset receives a total of 1,728,263 weekly downloads. As such, fileset popularity was classified as popular.
We found that fileset demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.