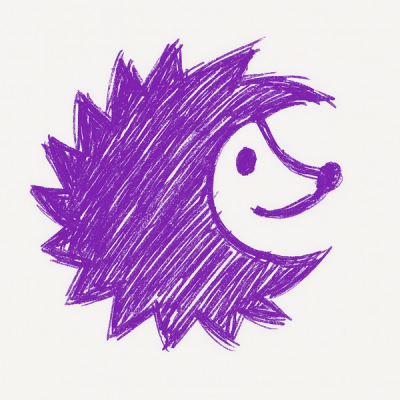
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
filled-array
Advanced tools
The filled-array npm package is a utility that allows you to create an array filled with a specified value. It is useful for initializing arrays with default values or for creating arrays of a specific length with repeated elements.
Create an array filled with a specific value
This feature allows you to create an array of a specified length, filled with a given value. In the code sample, an array of length 5 is created, with each element being the string 'x'.
const filledArray = require('filled-array');
const result = filledArray('x', 5);
console.log(result); // ['x', 'x', 'x', 'x', 'x']
Create an array using a function to generate values
This feature allows you to create an array where each element is generated by a function. The function receives the index of the element as an argument. In the code sample, an array of length 4 is created, with each element being twice its index.
const filledArray = require('filled-array');
const result = filledArray((index) => index * 2, 4);
console.log(result); // [0, 2, 4, 6]
The array-fill package provides similar functionality to filled-array, allowing you to fill an existing array with a specified value. Unlike filled-array, which creates a new array, array-fill modifies an existing one.
Lodash is a popular utility library that includes a method _.fill, which can fill elements of an array with a specified value. While lodash offers a broader range of utilities beyond array filling, its _.fill method is comparable to filled-array's functionality.
This is a polyfill for the native Array.prototype.fill method, which is part of the ECMAScript 2015 (ES6) standard. It provides similar functionality to filled-array, allowing you to fill an array with a static value. Unlike filled-array, this method is built into modern JavaScript engines.
Returns an array filled with the specified input
npm install filled-array
import filledArray from 'filled-array';
filledArray('x', 3);
//=> ['x', 'x', 'x']
filledArray(0, 3);
//=> [0, 0, 0]
filledArray(index => {
return (++index % 3 ? '' : 'Fizz') + (index % 5 ? '' : 'Buzz') || index;
}, 15);
//=> [1, 2, 'Fizz', 4, 'Buzz', 'Fizz', 7, 8, 'Fizz', 'Buzz', 11, 'Fizz', 13, 14, 'FizzBuzz']
Type: unknown
The value to fill the array with.
You can pass a function to generate the array items dynamically. The function is expected to return the value for each iteration and will be called with the following arguments: index, the count you passed in, and the filled array thus far.
Type: number
(non-negative integer)
The number of items to fill the array with.
FAQs
Returns an array filled with the specified input
The npm package filled-array receives a total of 130,064 weekly downloads. As such, filled-array popularity was classified as popular.
We found that filled-array demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.